How to Check Exit Code in Bash
- Understanding Exit Codes
-
Method 1: Using the
$?
Variable -
Method 2: Using Conditional Execution with
&&
and||
-
Method 3: Using
trap
for Error Handling - Conclusion
- FAQ
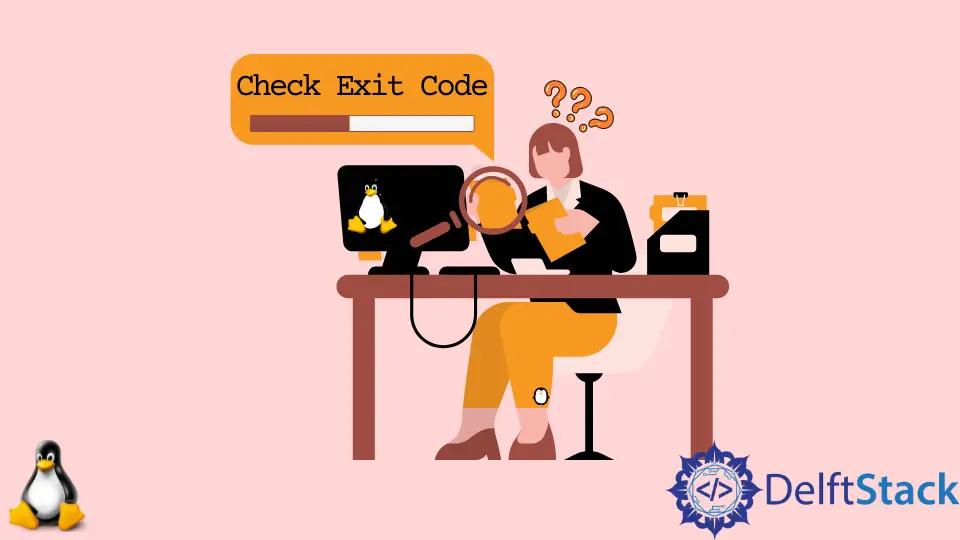
When working with Bash, understanding exit codes is crucial for effective scripting and command execution. Whether you’re a seasoned developer or just starting, knowing how to check the exit status of commands can save you from potential headaches. An exit code is a numeric value returned by a command, indicating whether it was successful or encountered an error.
In this tutorial, we will explore various methods to check the exit code when using Git commands in Bash. By the end, you will have a solid grasp of how to handle exit codes, which will enhance your command-line proficiency.
Understanding Exit Codes
Before diving into the methods, it’s essential to understand what exit codes are and why they matter. In Unix-like systems, every command returns a status code upon completion. A return value of 0 typically signifies success, while any non-zero value indicates an error. This mechanism allows scripts to make decisions based on the success or failure of commands. By checking these exit codes, you can create more robust and error-resistant scripts.
Method 1: Using the $?
Variable
One of the simplest ways to check the exit code of the last executed command in Bash is by using the special variable $?
. This variable holds the exit status of the last command run, making it easy to retrieve and use in your scripts.
Here’s how you can use it with Git commands:
git clone https://github.com/example/repo.git
echo $?
Output:
0
In this example, we attempt to clone a Git repository. After executing the git clone
command, we immediately print the exit code using echo $?
. If the cloning is successful, it will return 0. If there was an error (for example, if the repository does not exist), it will return a non-zero value. This method is straightforward and effective for quick checks.
You can also utilize this in conditional statements. For instance:
git pull origin main
if [ $? -ne 0 ]; then
echo "Git pull failed!"
else
echo "Git pull successful!"
fi
Output:
Git pull successful!
This script attempts to pull changes from the main branch. If the command fails, it prints an error message. This way, you can handle errors gracefully and take appropriate actions based on the exit code.
Method 2: Using Conditional Execution with &&
and ||
Another effective way to manage exit codes in Bash is through conditional execution using &&
and ||
. This method allows you to chain commands based on the success or failure of previous commands.
Here’s how you can use it with Git commands:
git fetch origin && echo "Fetch successful!" || echo "Fetch failed!"
Output:
Fetch successful!
In this example, the git fetch
command is executed first. If it succeeds (exit code 0), the message “Fetch successful!” is printed. If it fails (non-zero exit code), the message “Fetch failed!” is displayed instead. This chaining of commands is a powerful way to handle success and failure scenarios in a clean and concise manner.
You can also combine multiple commands:
git checkout feature-branch && git merge main || echo "Merge failed!"
Output:
Merge failed!
In this case, if the git checkout
command is successful, it proceeds to merge the main branch. If any command fails, it prints “Merge failed!” This method enhances the readability of your scripts and allows for quick debugging.
Method 3: Using trap
for Error Handling
For more advanced error handling in Bash scripts, you can use the trap
command. This allows you to define a function that will be executed when a command fails, making it easier to manage errors globally within your script.
Here’s a basic example using Git commands:
trap 'echo "An error occurred. Exiting..."; exit 1;' ERR
git push origin feature-branch
Output:
An error occurred. Exiting...
In this script, the trap
command sets up a handler for any command that returns a non-zero exit code. If the git push
command fails, the specified message is printed, and the script exits with a status of 1. This method is particularly useful for larger scripts where you want to ensure that any error is handled consistently.
You can also customize the error message based on the command that failed:
trap 'echo "Error occurred during Git operations"; exit 1;' ERR
git clone https://github.com/example/repo.git
git checkout feature-branch
Output:
Error occurred during Git operations
This approach provides a centralized way to handle errors, making your scripts more maintainable and easier to understand.
Conclusion
Checking exit codes in Bash is a fundamental skill that can significantly improve your command-line experience. By understanding and utilizing the methods we’ve discussed, you can create more efficient and error-resistant scripts. Whether you’re using the $?
variable, conditional execution, or the trap
command, each method has its strengths and can be applied based on your specific needs. With practice, these techniques will become second nature, allowing you to navigate the world of Bash and Git with confidence.
FAQ
- what is an exit code in Bash?
An exit code in Bash is a numeric value returned by a command that indicates whether it was successful or encountered an error.
-
how do I check the exit code of a command in Bash?
You can check the exit code of the last executed command using the special variable$?
. -
what does an exit code of 0 mean?
An exit code of 0 typically means that the command was successful. -
can I use exit codes in conditional statements?
Yes, you can use exit codes in conditional statements to handle success and failure scenarios in your scripts. -
what is the purpose of the trap command in Bash?
Thetrap
command allows you to define a function that will be executed when a command fails, providing a way to handle errors globally in your scripts.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn