How to Use of Stack Data Structure in Kotlin
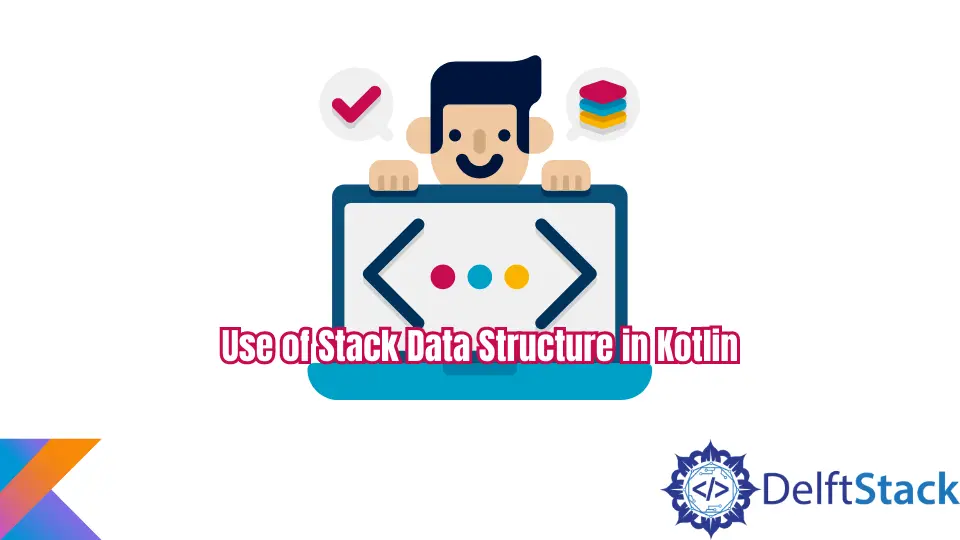
Today, we will learn the use of stack in Kotlin. We will also see standard functions used to update and alter the Kotlin stack that includes push
, pop
, peek
, search
, and isEmpty
.
We will also look at a better alternative than the stack in Kotlin.
Stack Data Structure in Kotlin
Stack is a data structure that follows a last in, first out (LIFO
) mechanism. It means we can use it to create a database of multiple objects.
The use of stack is prominent in Java as it is a part of the Java collections framework, but we can create a stack in Kotlin by importing the Stack
library from Java.
Once the library is imported, we can create a stack and add objects. This example creates a stack named cars
and then uses the addAll()
method to add car brand names.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf("Mercedes-Benz", "BMW", "Ferrari", "Porsche"))
for(c in cars){
println(c)
}
}
Output:
As you can see, the above code example creates a stack and adds four car brands. Next, we will look at using some predefined functions to operate this stack.
push()
Function in Kotlin Stack
The push()
function allows adding a new object to the stack. The added object is moved to the top of the stack by default.
In the below example, we will use the push()
function and add another object to the stack we created above.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf("Mercedes-Benz", "BMW", "Ferrari", "Porsche"))
cars.push("Lamborghini")
for(c in cars){
println(c)
}
}
Output:
pop()
Function in Kotlin Stack
As the name implies, the pop()
function pops out the last element added to the stack. Hence, the last in, first out mechanism is removed first because the element added last.
So, considering the same example, if we want to remove the object on the top of the stack, i.e., Lamborghini
, we can do that using the pop()
function. Here’s the code for the same.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf("Mercedes-Benz", "BMW", "Ferrari", "Porsche"))
cars.push("Lamborghini")
for(c in cars){
println(c)
}
var poppedCar = cars.pop()
println("\nThe car brand that is popped out is: " + poppedCar)
println("\nThe new list of car brands is: ")
for(c in cars){
print(c + ", ")
}
}
Output:
peek()
Function in Kotlin Stack
The peek()
function allows peeking in the Kotlin stack. It means we can find the object at the top of the stack without removing it.
The example below shows the peek()
function. Next, we will add the car brand Lamborghini
to the stack and print it using the peek()
function.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf("Mercedes-Benz", "BMW", "Ferrari", "Porsche"))
cars.push("Lamborghini")
for(c in cars){
println(c)
}
var peekCar = cars.peek()
println("\nPeeked Car is: " + peekCar)
}
Output:
search()
Function in Kotlin Stack
If you want to search a specific element in the stack, we can use the search()
function. We can also search for an object if we are not sure if it is there in the stack or not.
If the searched element is available in the stack, the function will return the number where it is present in the stack. But if the element is unavailable, it will return -1
.
Let’s look at this example where we search for two car brands in the cars
stack. We will look for the elements Ferrari
and Jeep
.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf("Mercedes-Benz", "BMW", "Ferrari", "Porsche"))
cars.push("Lamborghini")
for(c in cars){
println(c)
}
println("\nLooking for Ferrari: " + cars.search("Ferrari"))
println("Looking for Jeep: " + cars.search("Jeep"))
}
Output:
In the above output, the first search()
function returned 3
as the Ferrari is at the 3rd
position. But the second search()
function returns -1
as Jeep is not there in the stack.
isEmpty()
Function in Kotlin Stack
If we try to perform some functions on an empty stack, it throws an error. For example, using the pop()
function on an empty stack throws java.util.EmptyStackException
.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf())
var v = cars.pop()
}
Output:
Hence, it is essential to know whether a stack is empty. You can get to know this using the isEmpty()
function as given below.
import java.util.Stack
fun main(args: Array<String>){
var cars = Stack<String>();
cars.addAll(listOf("Mercedes-Benz", "BMW", "Ferrari", "Porsche", "Lamborghini"))
if(cars.isEmpty()){
println("This stack is empty")
}
else{
println("This stack is not empty")
}
}
Output:
Use of ArrayDeque()
in Kotlin
While stack is a useful data structure, it is not advised to use it in Kotlin. Instead, it is recommended to use Deque
. Deque means a double-ended queue, which can work both as a queue and a stack.
The kotlin.collections.ArrayDeque
was introduced in Kotlin 1.3.70. Here’s how you can use Deque
in place of the Kotlin stack and perform the same operations as with a stack.
import java.util.ArrayDeque
fun main(args: Array<String>){
var stackExample = ArrayDeque<String>()
stackExample.push("Mercedes-Benz")
stackExample.push("BMW")
stackExample.push("Ferrari")
stackExample.push("Porsche")
println(stackExample)
println("\n" + stackExample.isEmpty())
println("\n" + stackExample.pop())
println("\n" + stackExample)
stackExample.push("Lamborghini")
println("\n" + stackExample)
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn