How to Use of findViewById Function in Kotlin
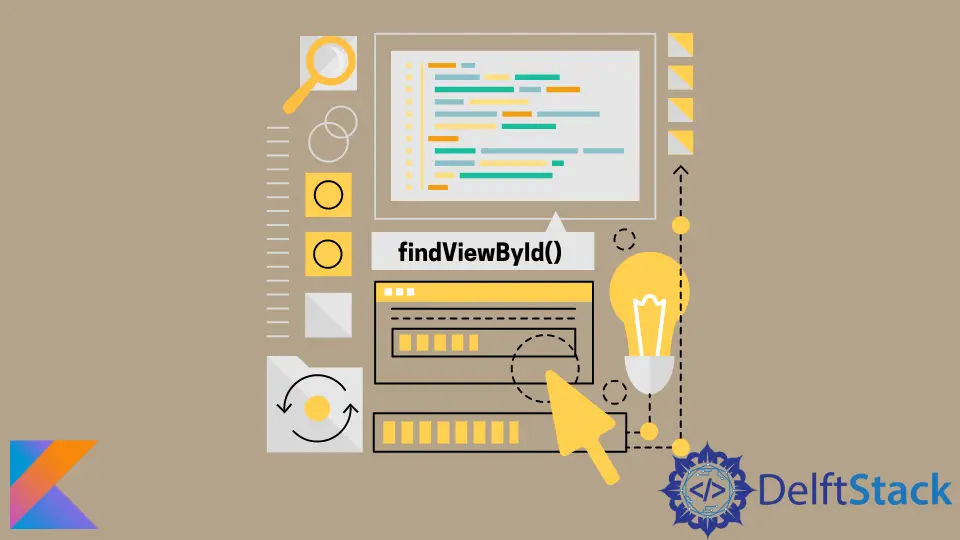
This article will teach you the Kotlin findViewById
function. It will also show you an alternative way to access and update the view without using the findViewById
function.
Use of the findViewById
Function in Kotlin
The findViewById
function is a part of Android’s View
and Activity
classes. It enables us to access and update an existing view through an ID
.
We can use it with any views, including TextView
, LinearLayout
, Button
, etc. To use the Kotlin findViewById
function, we need to assign an ID
to a view while defining it.
We can do this in the .xml
file. Here’s the syntax of assigning an ID
to a view:
<?xml version="1.0" encoding="utf-8"?>
<TextView
android:id="@+id/id_text_view"
android:text="Kotlin Programming!"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Once we have defined the view, we can access it from the main
Kotlin file in the following way:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView myTextView = findViewById(R.id.id_text_view);
}
As you can see above, the Kotlin findViewById
function accepts only one parameter: the ID
.
Alternative Way: Use of Kotlin Android Extensions
While we can use the Kotlin findViewById
, it is recommended to use Kotlin Android extensions. This extension allows more efficient access to Activities
, Fragments
, and Views
.
The plugin generates some extra code that allows accessing the views from the layout XML. The extension plugin also builds a cache.
It means that the first time we access the view, it will use the regular findViewById
function, but every time after that, it will access the view from the cache.
It allows for quicker access to the view. To use the Kotlin Android Extension, we need to add it using the apply
keyword in the Android Module.
apply plugin: 'com.android.application'
apply plugin: 'kotlin-android'
apply plugin: 'kotlin-android-extensions'
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn