How to Implement Null Check in Kotlin
-
Use Conditional Statements to Implement a
Null
Check in Kotlin -
Use Safe Calls to Implement a
Null
Check in Kotlin -
Use the Elvis Operator to Implement a
Null
Check in Kotlin
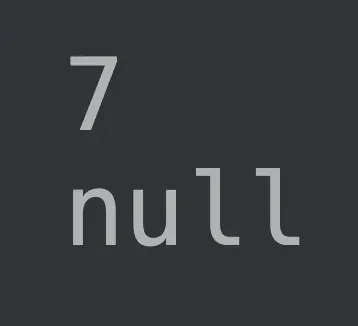
Several primitive types do not accept null
and throw errors. Errors made with null
references can cause application failure or even lead to system crashes; hence, it is vital to check null
references before running a program.
Kotlin allows us to check null
in various ways. This article will teach you how to check for null
in Kotlin and prevent NullPointerException
errors.
Use Conditional Statements to Implement a Null
Check in Kotlin
The easiest way to check null
in Kotlin is by using conditional statements. Here, we can use conditional operators ==
or !=
and execute the conditional statements accordingly.
We can also use ===
instead of ==
and !==
instead of !=
. A structural equality x == null
is anyhow translated to referential equality x === null
; hence, it doesn’t make much difference whether you use ==
or ===
.
Here is an example to show the use of conditional statements for the null
check in Kotlin.
fun main() {
val x: String? = "Android"
if (x != null && x.length > 0) {
print("This is not null, its length is: ${x.length}")
} else {
print("This is a null string!")
}
}
Output:
Click here to check the demo of the example.
Now, if we change the value to null
, it will give the other output.
fun main() {
val x: String? = null
if (x != null && x.length > 0) {
print("This is not null, its length is: ${x.length}")
} else {
print("This is a null string!")
}
}
Output:
Click here to check the demo of the example.
Conditional statements work only if the variable is declared using the val
keyword. The val
keyword makes the word immutable.
If we use a var
variable, there are chances that the value of the variable changes after we check for the null
references.
Use Safe Calls to Implement a Null
Check in Kotlin
Safe calls are another option to access nullable properties for the null
check in Kotlin. We can use the safe call operator ?.
to check the result of an expression.
Based on the expression results, we can then execute the further statements.
Here is an example.
fun main() {
val v = "Android"
val x: String? = null
println(v?.length)
println(x?.length)
}
Output:
Click here to check the demo of the example.
Safe calls are especially useful in creating chains. For instance, we can write:
a?.b?.c?.d
In the above example, if a
is not null
, it will move to b
. If b
is not null
, it will move to c
, and if c
is not null
, it will move to d
.
Whenever the compiler finds a null
value, it stops executing and returns null
.
Use Safe Calls With the let
Function to Handle the Null
in Kotlin
Usually, if one of the values of a list is null
, the compiler returns null
. However, we can combine the let
function with safe calls to work only on non-null
values of a list.
Example:
fun main() {
val myList: List<String?> = listOf("David", "Virat", "Steve", null)
for (i in myList) {
i?.let { println(it) } // prints only non-null values
}
}
Output:
Click here to check the demo of the example.
Use the Elvis Operator to Implement a Null
Check in Kotlin
Another excellent option for Kotlin null
check is using the Elvis operator ?:
. It will return a non-null
or a default value if it finds a null
value in the references.
There are two expressions: on the left and right of the Elvis operator. If the value of the left expression is not null
, the operator returns the value, but if the left expression is null
, the Elvis operator returns the value of the expression on the right side.
The key here is that the Elvis operator will only execute the expression on the right if the left expression is null
.
Example:
fun main(args: Array<String>) {
val i: String? = null
val j: String = i ?: "Welcome! This is Kotlin Programming."
// This print statement uses the Elvis operator
println(i ?: j)
}
Output:
Click here to check the demo of the example.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn