Swift if Let Statement Equivalent in Kotlin
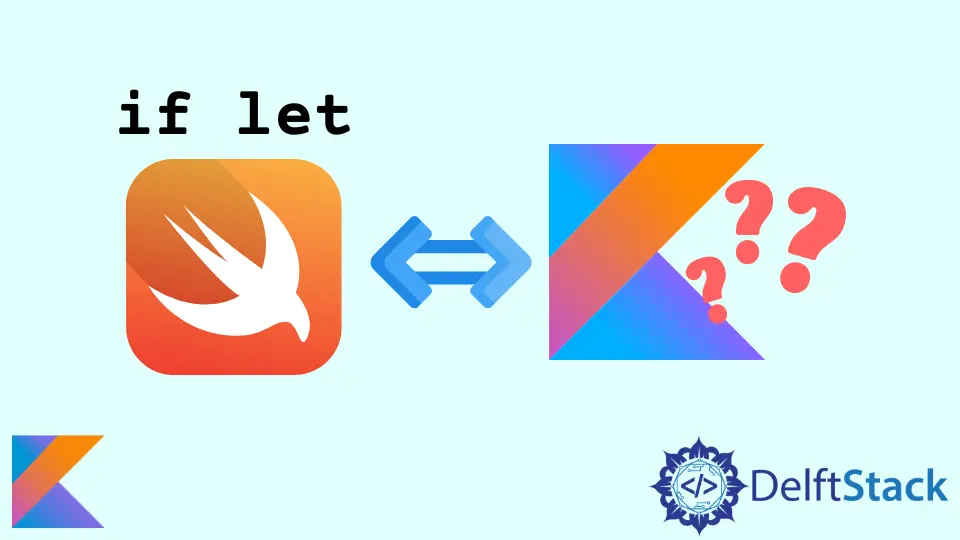
Kotlin has become a popular language for Android development, and its features often draw comparisons to Swift, especially when it comes to handling optionals and nullability. One such feature is the if let
statement in Swift, which is used for safely unwrapping optionals. In Kotlin, the equivalent functionality can be achieved using the let
and run
functions.
This article explores how these Kotlin functions work, their syntax, and provides practical examples to illustrate their usage. By the end of this article, you will have a solid understanding of how to effectively use Kotlin’s let
and run
as alternatives to Swift’s if let
.
Understanding Kotlin let and run
Both let
and run
are scope functions in Kotlin that allow you to work with objects in a concise and readable manner. They enable you to execute code blocks with the object as the context, making it easier to handle nullable types without explicitly checking for null.
Kotlin let
The let
function is called on an object and takes a lambda expression as a parameter. The object itself is passed as an argument to the lambda, allowing you to perform operations on it if it is not null.
Example of Kotlin let
val name: String? = "John"
name?.let {
println("Hello, $it!")
}
Output:
Hello, John!
In this example, the let
function is invoked on the nullable variable name
. The safe call operator ?.
ensures that the let
block only executes if name
is not null. Inside the block, it
refers to the non-null value of name
, allowing us to print a greeting.
Kotlin run
The run
function is similar to let
, but it is used when you want to execute a block of code on an object and return the result of that block. Like let
, run
also works with nullable types, but it does not require the safe call operator.
Example of Kotlin run
val age: Int? = 25
val result = age?.run {
this * 2
}
println("Double the age is: $result")
Output:
Double the age is: 50
In this example, run
is called on the nullable variable age
. If age
is not null, the block multiplies the value by 2 and returns the result. If age
were null, result
would also be null, demonstrating how run
can handle nullable types seamlessly.
Practical Use Cases for let and run
Using let
and run
effectively can streamline your code and improve its readability. Here are some practical scenarios where these functions can be beneficial.
1. Chaining Operations
You can chain multiple operations together using let
and run
, which can be particularly useful when dealing with complex data transformations.
Example of Chaining with let
val user: User? = getUser()
user?.let {
it.updateProfile()
it.sendNotification()
}
Output:
Profile updated
Notification sent
In this example, we retrieve a user object, and if it is not null, we call two methods on it: updateProfile()
and sendNotification()
. The chaining of operations leads to cleaner code.
2. Configuring Objects
The run
function is often used to configure objects before using them. This can be particularly useful in scenarios like building UI components or initializing data classes.
Example of Configuring with run
val userProfile = UserProfile().apply {
name = "Alice"
age = 30
}.run {
"User: $name, Age: $age"
}
println(userProfile)
Output:
User: Alice, Age: 30
Here, we create a UserProfile
object and configure its properties using apply
. The run
function then allows us to create a formatted string with the user’s information, showcasing how you can combine the two functions for effective object configuration.
Conclusion
Understanding how to use Kotlin’s let
and run
functions provides a powerful toolset for managing nullability and executing code blocks in a concise manner. These functions not only enhance the readability of your code but also help you avoid common pitfalls associated with null references. By leveraging these features, you can write cleaner, more maintainable Kotlin code that mirrors the functionality of Swift’s if let
statement. As you continue to explore Kotlin, you’ll find that these scope functions are invaluable in your programming toolkit.
FAQ
-
What is the difference between let and run in Kotlin?
let is used for executing a block of code with the object as the context, while run is used for executing a block of code and returning a result. -
Can I use let and run with nullable types?
Yes, both let and run can be used with nullable types, allowing you to safely execute code only when the object is not null. -
How do I handle null values in Kotlin?
You can use safe calls (?.) along with let and run to handle null values gracefully. -
Are let and run the only scope functions in Kotlin?
No, Kotlin also provides other scope functions like apply and with, each serving different purposes.
- Can I chain multiple let and run calls together?
Yes, you can chain multiple calls to let and run, enabling you to perform complex operations in a concise manner.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn