How to Use forEach in Kotlin
- Understanding the forEach Function in Kotlin
- Using forEach with Different Collections
- Benefits of Using forEach in Kotlin
- Conclusion
- FAQ
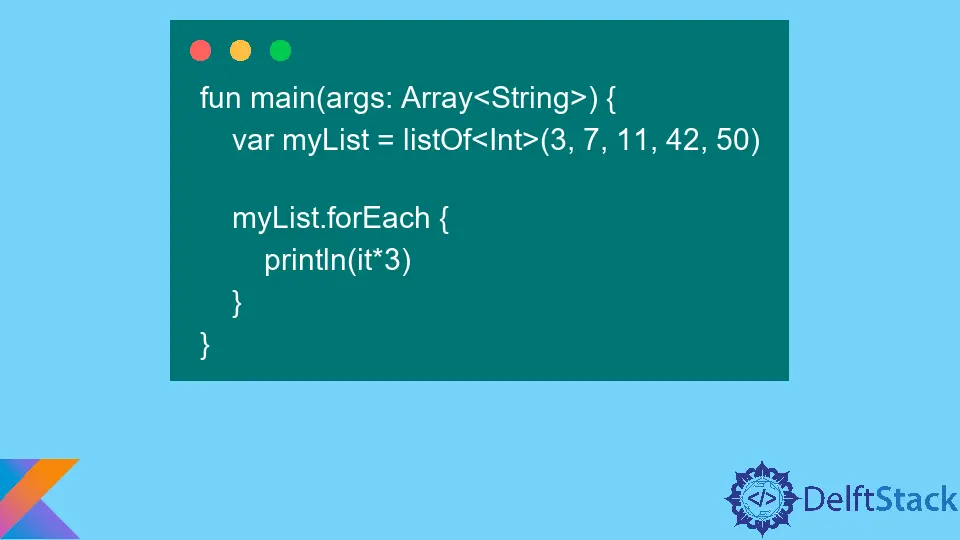
In the world of programming, loops are essential tools that allow us to iterate over collections and perform actions on each element. Kotlin, a modern programming language, offers a variety of looping mechanisms, one of which is the forEach
function.
This article introduces the concept and use of the forEach
keyword in Kotlin. We’ll explore its syntax, functionality, and practical applications through examples, making it easier for you to understand how to implement it in your projects. Whether you’re a beginner or looking to refine your skills, this guide will provide you with the insights you need to effectively use forEach
in Kotlin.
Understanding the forEach Function in Kotlin
The forEach
function is a higher-order function in Kotlin that allows you to iterate through collections such as lists, sets, and maps. It takes a lambda expression as an argument, which defines the action to be performed on each element of the collection. This makes the code cleaner and more concise compared to traditional loops.
Here’s a simple example demonstrating how to use forEach
with a list:
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
numbers.forEach { number ->
println(number)
}
}
Output:
1
2
3
4
5
In this example, we create a list of integers and then use forEach
to print each number in the list. The lambda expression number -> println(number)
is executed for every element in the numbers
list. This approach is not only more readable but also eliminates the need for explicit index management.
Using forEach with Different Collections
Kotlin’s forEach
can be applied to various collections beyond just lists. It can be effectively used with sets and maps, allowing for versatile data manipulation.
Example with a Set
Let’s see how to use forEach
with a set:
fun main() {
val fruits = setOf("Apple", "Banana", "Cherry")
fruits.forEach { fruit ->
println(fruit)
}
}
Output:
Apple
Banana
Cherry
In this example, we define a set of fruits and use forEach
to print each fruit. The order of output may vary since sets do not maintain order. This illustrates how forEach
accommodates different types of collections seamlessly, allowing you to focus on the logic rather than the underlying implementation.
Example with a Map
Using forEach
with maps is equally straightforward. Here’s how you can iterate through a map:
fun main() {
val ages = mapOf("Alice" to 25, "Bob" to 30, "Charlie" to 35)
ages.forEach { (name, age) ->
println("$name is $age years old")
}
}
Output:
Alice is 25 years old
Bob is 30 years old
Charlie is 35 years old
In this case, we have a map where the keys are names and the values are ages. The forEach
function allows us to destructure the key-value pairs directly in the lambda, making the code concise and easy to read. This demonstrates the flexibility of forEach
in handling complex data structures.
Benefits of Using forEach in Kotlin
Using forEach
has several advantages that enhance your programming experience in Kotlin. First, it promotes a functional programming style, making your code more expressive and easier to understand. Instead of focusing on the mechanics of iteration, you can concentrate on the action being performed on each element.
Second, forEach
eliminates the need for boilerplate code associated with traditional loops. This not only reduces the chance of errors but also improves code maintainability. When you need to perform an operation on each item in a collection, forEach
provides a clear and efficient way to do so.
Lastly, forEach
integrates seamlessly with Kotlin’s other collection functions, allowing you to chain operations for more complex data manipulations. This can lead to cleaner code and improved performance, as you can leverage Kotlin’s powerful standard library.
Conclusion
In summary, the forEach
function in Kotlin is a powerful tool for iterating over collections. Its concise syntax and functional programming style make it a preferred choice for many developers. Whether you are working with lists, sets, or maps, forEach
simplifies your code and enhances readability. By incorporating forEach
into your Kotlin programming practices, you can write more efficient and maintainable code.
FAQ
-
What is the purpose of the forEach function in Kotlin?
TheforEach
function is used to iterate over collections and perform actions on each element using a lambda expression. -
Can I use forEach with any collection type in Kotlin?
Yes,forEach
can be used with various collection types, including lists, sets, and maps. -
How does forEach improve code readability?
forEach
eliminates boilerplate code and allows developers to focus on the actions performed on elements, making the code cleaner and more expressive. -
Is forEach a standard function in Kotlin?
Yes,forEach
is a standard library function available for Kotlin collections. -
Can I use forEach with custom objects in Kotlin?
Absolutely! You can useforEach
with collections of custom objects in Kotlin, allowing you to manipulate complex data structures easily.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn