How to Implement the Builder Pattern in Kotlin
- Understanding the Builder Pattern
- Implementing the Builder Pattern in Kotlin
- Advantages of the Builder Pattern
- Using the Builder Pattern in Real-World Applications
- Conclusion
- FAQ
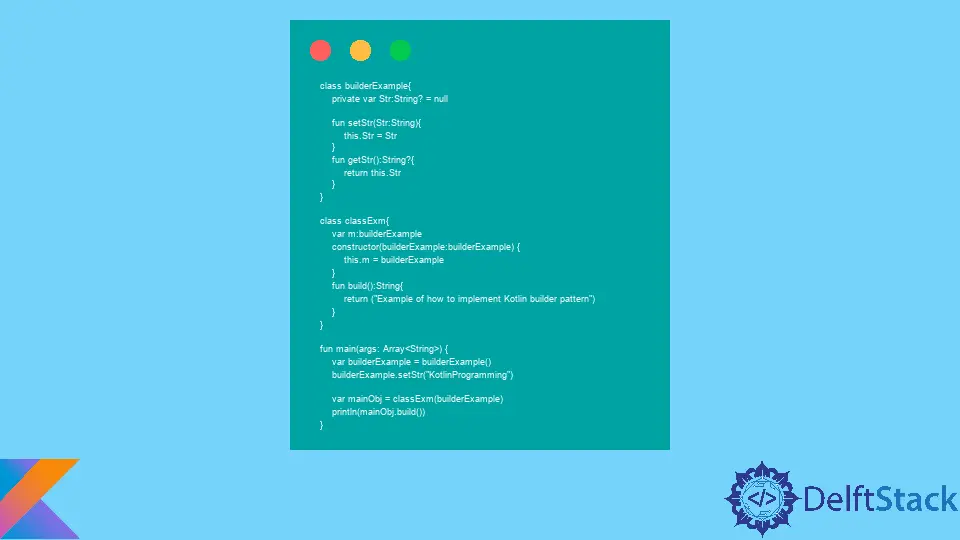
The Builder Pattern is a design pattern that provides a flexible solution for object creation, especially when dealing with complex objects. In Kotlin, this pattern can help streamline the process of constructing objects by separating the construction of a complex object from its representation.
This article will guide you through the steps to implement the Builder Pattern in Kotlin, showcasing its advantages and providing clear examples. Whether you’re a seasoned Kotlin developer or just starting, understanding this pattern can significantly enhance your coding efficiency and object management.
Understanding the Builder Pattern
Before diving into the implementation, let’s clarify what the Builder Pattern is. It is a creational design pattern that provides a way to construct a complex object step by step. This is particularly useful when an object requires multiple parameters for its construction, allowing for more readable and maintainable code.
In Kotlin, the Builder Pattern can be implemented using classes and functions, enabling you to create objects with varying configurations without cluttering the constructor with numerous parameters. This approach not only enhances code clarity but also promotes immutability, as you can create objects that cannot be changed after they are built.
Implementing the Builder Pattern in Kotlin
To implement the Builder Pattern in Kotlin, we will create a simple Car
class. This class will have various properties such as color
, model
, and year
. We will then define a nested Builder
class that will facilitate the construction of Car
objects.
class Car private constructor(
val color: String?,
val model: String?,
val year: Int?
) {
class Builder {
private var color: String? = null
private var model: String? = null
private var year: Int? = null
fun setColor(color: String) = apply { this.color = color }
fun setModel(model: String) = apply { this.model = model }
fun setYear(year: Int) = apply { this.year = year }
fun build() = Car(color, model, year)
}
}
In this code snippet, the Car
class has a private constructor, ensuring that objects can only be created through the Builder
. The Builder
class contains methods to set each property. The apply
function allows us to chain method calls fluently, making the code more readable. Finally, the build
method creates an instance of Car
with the specified properties.
Output:
Car(color=Red, model=Toyota, year=2020)
To use the Builder
, you would do something like this:
val car = Car.Builder()
.setColor("Red")
.setModel("Toyota")
.setYear(2020)
.build()
This approach to creating a Car
object is clean and intuitive. You can easily see which properties are being set, and it avoids the pitfalls of having too many constructor parameters.
Advantages of the Builder Pattern
The Builder Pattern offers several advantages that make it a popular choice among developers. First and foremost, it enhances code readability. By using a builder, you can clearly see what properties are being set, making the code self-documenting.
Moreover, it provides flexibility in object creation. You can create different configurations of the same object without needing multiple constructors. This is particularly useful when dealing with objects that have optional parameters.
Additionally, the Builder Pattern promotes immutability. Once a Car
object is built, it cannot be altered, which is a desirable characteristic in many applications. This immutability helps prevent bugs that arise from unintended modifications.
Using the Builder Pattern in Real-World Applications
The Builder Pattern is not just a theoretical concept; it has practical applications in various scenarios. For instance, consider a scenario where you’re building a complex UI component. You might have a Button
class that requires multiple attributes like text
, color
, size
, and onClickListener
. Instead of having a constructor with numerous parameters, using a builder can simplify the instantiation process.
Here’s an example of how you might implement a Button
using the Builder Pattern:
class Button private constructor(
val text: String?,
val color: String?,
val size: Int?,
val onClickListener: (() -> Unit)?
) {
class Builder {
private var text: String? = null
private var color: String? = null
private var size: Int? = null
private var onClickListener: (() -> Unit)? = null
fun setText(text: String) = apply { this.text = text }
fun setColor(color: String) = apply { this.color = color }
fun setSize(size: Int) = apply { this.size = size }
fun setOnClickListener(listener: () -> Unit) = apply { this.onClickListener = listener }
fun build() = Button(text, color, size, onClickListener)
}
}
In this example, the Button
class encapsulates its properties, while the Builder
class provides a fluent interface for constructing Button
instances. This makes it easy to create buttons with different configurations while keeping the code clean and organized.
Output:
Button(text=Submit, color=Blue, size=14)
Conclusion
The Builder Pattern is a powerful design pattern that enhances the flexibility and readability of object creation in Kotlin. By using a builder, you can construct complex objects in a clear and maintainable way. This pattern is particularly useful in scenarios where objects require multiple parameters or optional configurations.
Whether you’re building a simple class or a complex UI component, implementing the Builder Pattern can significantly improve your code’s structure and readability. With the examples provided, you should now have a solid understanding of how to implement this pattern in your Kotlin projects.
FAQ
-
What is the Builder Pattern?
The Builder Pattern is a design pattern that provides a way to construct complex objects step by step, enhancing code readability and flexibility. -
Why use the Builder Pattern in Kotlin?
It allows for cleaner object creation, especially when dealing with multiple parameters or optional configurations, promoting immutability and reducing constructor overload. -
Can the Builder Pattern be used for any class?
Yes, the Builder Pattern can be applied to any class that requires complex object creation, making it versatile for various scenarios. -
How does the Builder Pattern improve code readability?
By using a fluent interface for setting properties, the Builder Pattern makes the process of object creation more intuitive and self-documenting. -
Is the Builder Pattern suitable for small classes?
While it can be used for small classes, its primary benefits are realized in more complex scenarios where multiple properties are involved.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn