How to Toggle a Text With jQuery
-
Use the
.text()
Method to Toggle the Text With jQuery - Extend jQuery Using a Custom Function to Toggle the Text
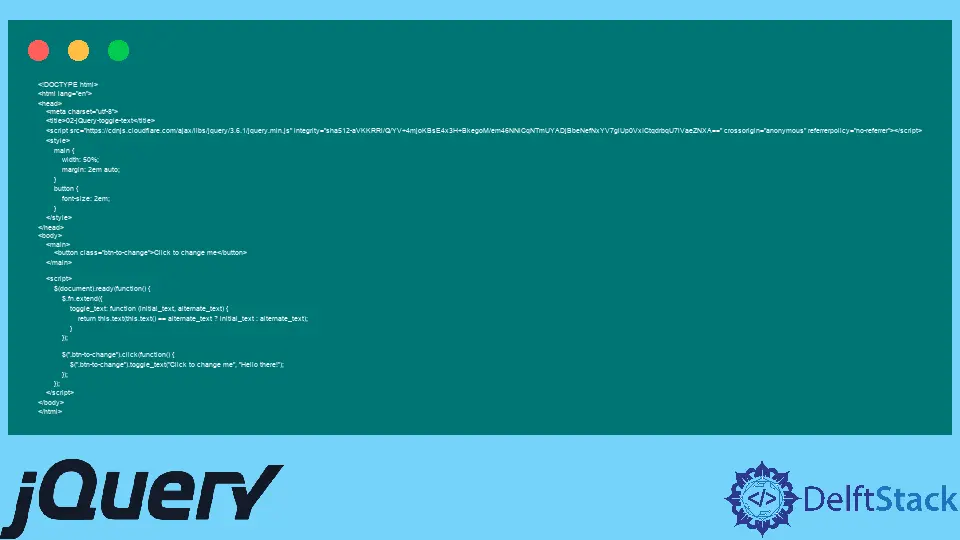
To toggle a text with jQuery, you can employ the .text()
method or extend the jQuery library with a custom function that toggles the text.
This article teaches you how to use both methods with code examples.
Use the .text()
Method to Toggle the Text With jQuery
The jQuery .text()
method can alter the text content of a selected element. With this knowledge, you can set up a conditional statement that’ll swap the text based on its content.
You can also use a ternary operator to keep things simple and on a single line. Now, in the following, there is an anchor tag with the text Reveal Answer
.
When you click on this text, you’ll fire up a click event that changes Reveal Answer
to Hypertext Markup Language
. A second click will revert the text to Reveal Answer
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-toggle-text</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
p {
font-size: 2em;
text-align: center;
}
</style>
</head>
<body>
<main>
<p>What is HTML? <a id="anchor-link" href="#">Reveal Answer</a></p>
</main>
<script>
$(document).ready(function() {
$("#anchor-link").click(function () {
let text = $("#anchor-link").text();
$("#anchor-link").text(
text == "Reveal Answer" ? "Hypertext Markup Language" : "Reveal Answer");
});
});
</script>
</body>
</html>
Output:
Extend jQuery Using a Custom Function to Toggle the Text
You can write a custom function that extends the jQuery library. This function will take two arguments; the first will be the initial text of the element, and the second is the text you want to swap to.
Like the first example, you’ll use a ternary operator to keep the code tidy and on one line. Now, in the HTML, you’ll find an HTML button with a text; when you click on it, it’ll change to another text.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-toggle-text</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
width: 50%;
margin: 2em auto;
}
button {
font-size: 2em;
}
</style>
</head>
<body>
<main>
<button class="btn-to-change">Click to change me</button>
</main>
<script>
$(document).ready(function() {
$.fn.extend({
toggle_text: function (initial_text, alternate_text) {
return this.text(this.text() == alternate_text ? initial_text : alternate_text);
}
});
$(".btn-to-change").click(function() {
$(".btn-to-change").toggle_text("Click to change me", "Hello there!");
});
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn