How to Set Hidden Field Value With jQuery
-
Use the
.val()
Method to Set the Hidden Field - Use CSS Attribute Selector to Set the Hidden Field
-
Use the
.attr()
Method to Set the Hidden Field
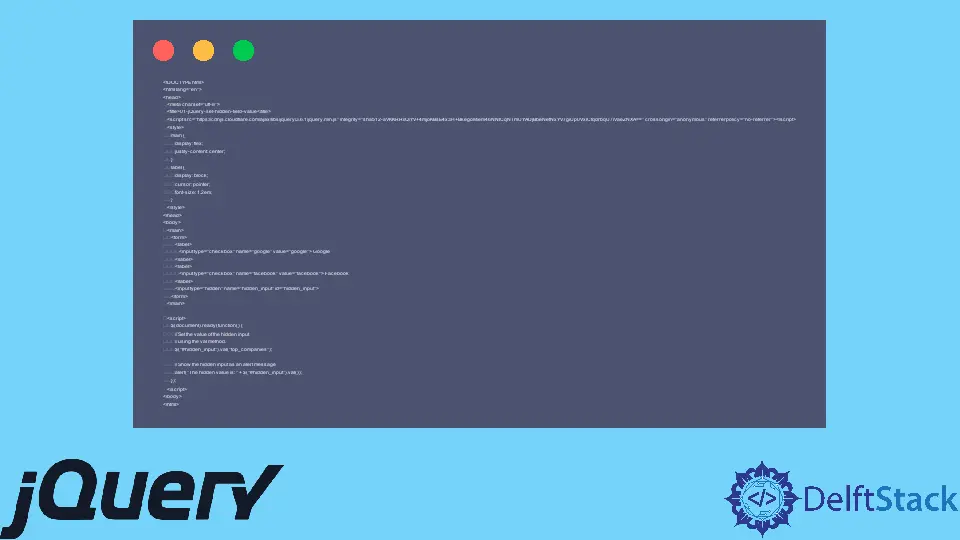
You can use three options to set a hidden field using jQuery. The first and second will use the .val()
and .attr()
methods, while the last will use a CSS attribute selector.
This article teaches how to use them all using code examples.
Use the .val()
Method to Set the Hidden Field
We can use the jQuery .val()
method to set the hidden field’s value. All you need is the right CSS selector that’ll act as a proxy; from there, you can change the value.
The HTML code in the following has an input value with type=hidden
; this makes it a hidden field. Afterward, you can use the .val()
method to set its value.
When you run the code in your web browser, you’ll see the hidden value in a JavaScript alert message.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-set-hidden-field-value</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
display: flex;
justify-content: center;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
</style>
</head>
<body>
<main>
<form>
<label>
<input type="checkbox" name="google" value="google"> Google
</label>
<label>
<input type="checkbox" name="facebook" value="facebook"> Facebook
</label>
<input type="hidden" name="hidden_input" id="hidden_input">
</form>
</main>
<script>
$(document).ready(function() {
// Set the value of the hidden input
// using the val method.
$("#hidden_input").val("top_companies");
// Show the hidden input as an alert message
alert("The hidden value is: " + $("#hidden_input").val());
});
</script>
</body>
</html>
Output:
Use CSS Attribute Selector to Set the Hidden Field
Using a CSS attribute selector to set a hidden field also requires the jQuery .val()
method. This is an option for you if you can’t use HTML IDs or CSS classes.
The following HTML is the same, but we’ve updated the checkboxes. In the jQuery code, you’ll grab the hidden input using a CSS attribute selector and set its value with the .val()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-set-hidden-field-value</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
display: flex;
justify-content: center;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
</style>
</head>
<body>
<main>
<form>
<label>
<input type="checkbox" name="erlang" value="erlang"> Erlang
</label>
<label>
<input type="checkbox" name="elixir" value="elixir"> Elixir
</label>
<input type="hidden" name="hidden_input" id="hidden_input">
</form>
</main>
<script>
$(document).ready(function() {
// Set the value of the hidden input
// using a CSS attribute selector
$("input[id=hidden_input]").val("functional_languages");
// Show the hidden input as an alert message
alert("The hidden value is: " + $("#hidden_input").val());
});
</script>
</body>
</html>
Output:
Use the .attr()
Method to Set the Hidden Field
The .attr()
method is a direct approach to setting a hidden value. The process is to grab the hidden field; use the .attr()
method to write a new hidden value.
From this, you can use the hidden value later in your code. Here, you’ll show it in a JavaScript alert message.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-set-hidden-field-value</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
display: flex;
justify-content: center;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
</style>
</head>
<body>
<main>
<form>
<label>
<input type="checkbox" name="hewlett-packard" value="hewlett-packard"> Hewlett-Packard
</label>
<label>
<input type="checkbox" name="asus" value="asus"> ASUS
</label>
<input type="hidden" name="hidden_input" id="hidden_input">
</form>
</main>
<script>
$(document).ready(function() {
// Set the value of the hidden input
// using
$("#hidden_input").attr("value", "computer_companies");
// Show the hidden input as an alert message
alert("The hidden value is: " + $("#hidden_input").val());
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn