How to Scroll to Element in jQuery
- Prerequisites for Scroll to Element in jQuery
- jQuery Scroll to Element - Scroll in the Document Window
-
jQuery Animate
ScrollTop
- Smooth Scrolling With theanimate
Method -
jQuery
ScrollTop
to Internal Link - Smooth Scrolling jQuery - jQuery Scroll to Element Inside a Container
- Tips to Keep in Mind
-
Two Quick Short-Cuts for jQuery
ScrollTop
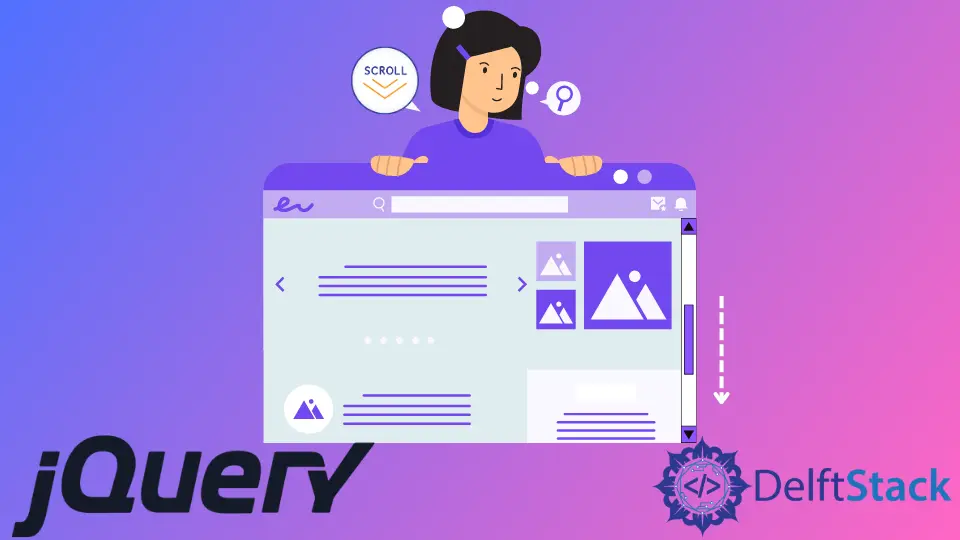
We show easy solutions to scroll to an element for different use cases in jQuery. We use the scrollTop()
and offset()
methods, but we also demo a new way with position()
.
You will see how to animate smooth scrolling with jQuery. Finally, we demo two common shortcuts and a few tips to avoid errors.
Prerequisites for Scroll to Element in jQuery
scrollTop()
offset()
position()
First, let us look at the methods we will use to scroll to an element in jQuery.
scrollTop()
The jQuery scrollTop()
method lets us get and set any element’s scrollTop
property. The value of the scrollTop
property is the number of pixels an element’s content is currently scrolled vertically.
You can know more about the scrollTop
property here.
.scrollTop()
- Get the Present scrollTop
Value
We use scrollTop()
without any argument to return the value of an element’s present scrollTop
value.
console.log($('#container').scrollTop());
We see the value of the element’s scrollTop
view in the console. Let us now scroll the element to a different position and log the value of the scrollTop
property again.
Here, we see the console logs a different value. The jQuery scrollTop()
method in this get
form is key in achieving our scroll effect.
.scrollTop(val)
- Set the New scrollTop
Value
We can pass the value we want to (vertically) scroll to in the jQuery scrollTop()
method in the set
form.
$('document.documentElement').scrollTop(0);
This scrolls the main document to the top because we pass the value 0
. We will see this again as a helpful shortcut.
We will pass in some key values to this set
form of scrollTop()
to achieve our jQuery scroll to element behavior.
You can know about the jQuery scrollTop()
function in detail here.
offset()
- Find the Position of an Element to Help Implement Smooth Scrolling in jQuery
The jQuery offset()
method lets us determine the current position of any element for the document. This method returns two properties, top
and left
; these are the number of pixels of the document’s border-box (i.e., without the margins).
console.log(`The position of the element w.r.t document is : ${
$('#container').offset().top}`);
The offset()
method displays the element’s top position relative to the document.
Read more about the jQuery offset()
method here.
position()
- Another Way to Implement Scroll to Element in jQuery
The position()
method is similar to the offset()
method with a small difference - it returns the element’s position relative to its nearest parent. So, when we are tweaking the relative position of an element, for instance, to animate scrolltop
in jQuery, the position()
method is useful.
You can get the details about the jQuery position()
method at this link.
position()
returns the position of the element, including its margins (here, it differs from the offset()
method).
// displays the position w.r.t. its closest parent
console.log(`This is the position of the element w.r.t. its closest parent:
${$('.filler:first').position().top}`);
// the offset() method displays position relative to the document
console.log(`This is the position of the element w.r.t. the document:
${$('.filler:first').offset().top}`);
With the basics out of our way, let’s go to scroll to an element for different use cases in jQuery.
jQuery Scroll to Element - Scroll in the Document Window
$('#clickButton').on('click', function() {
$([
document.documentElement, document.body
]).scrollTop($('#scrollToMe').offset().top);
});
#clickButton
is the button to click to scroll to the target element.#scrollToMe
is the element we want to scroll to.
Let us break the code down:
We attach an event handler to our button with the .on
method. The event to trigger this handler is the first argument, the "click"
event.
When the user clicks on the button, the code executes the (anonymous) callback function in the second argument to the .on
method.
We first select the entire document to scroll with either of the two selector arguments - document.documentElement
, which is the root element, or in this case, the html
element, and the document.body
element.
We then run the scrollTop()
method on this element in its set
form (see above). We want to scrolltop
to our target element - the image element with the #scrollToMe
ID.
So, we first find the number of pixels from the top of the page to this target image element with the offset().top
method - remember this method finds the position relative to the document.
Then, we pass this value to the scrollTop()
method attached to the document root/body element. So, the entire web page moves to the target image element, as seen in the video demonstration above.
Thus, this method easily achieves the jQuery scroll to element behavior.
But, honestly, this instant scroll to the target element looks boring. Let’s add more code to get the nice-looking smooth scrolling jQuery effect.
jQuery Animate ScrollTop
- Smooth Scrolling With the animate
Method
$('#clickButton').on('click', function() {
$([
document.documentElement, document.body
]).animate({scrollTop: $('#scrollToMe').offset().top}, 500)
});
The code is similar to the previous method. We only run the animate()
method on the selected root/body element.
A first argument is an object with the target CSS property:value
pairs we want to animate to.
Here, we only want to change the scrollTop
property to reach our target image element. So, we assign the value of the number of pixels from the page top to the image.
We find this out with the .offset().top
method on the image element, just like in the previous method.
jQuery ScrollTop
to Internal Link - Smooth Scrolling jQuery
We can also create a reusable jQuery scrolltop
module to any internal link with the following code.
$('a[href^="#"]').on('click', function(e) {
e.preventDefault();
let targetHash = this.hash;
let targetEle = $(targetHash);
$([
document.documentElement, document.body
]).animate({scrollTop: targetEle.offset().top}, 500);
})
Let us drill down into the code logic here. (Please refer to the scrollToInternalLink.html
, scrollToInternalLink.js
, and the scrollToInternalLink.css
files.)
First, we select a
tags with the [href^="#"]
jQuery attribute selector. We use the ^
sign, so jQuery only selects those a
tags with an href
attribute that begins with the #
sign.
We link to any element internally using fragment identifiers. These are references to internal links, and we always precede them with the #
sign.
So, our jQuery selector above selects the internal links on the page.
You can read more about internal links in HTML here.
We then call the e.preventDefault()
method to disable the default behavior of the a
tag that reloads the page.
Now, we extract the hash
of the target element - this is the reference to the “fragment identifier” of the target element. Most modern HTML code uses our target element’s id
attribute.
We use this hash
to select our target element.
The rest of the code is the same as the jQuery animate to scroll to
code above. We pass the animate()
method and set the scrollTop
property to the target element’s offset().top
value.
jQuery Scroll to Element Inside a Container
Sometimes, our target element is located inside a scrollable container. We can use the following code to jQuery to an element positioned like this.
let container = $('#container');
let scrollToMe = $('#scrollToMe');
$('button').on('click', function() {
container.animate(
{
scrollTop: scrollToMe.offset().top - container.offset().top +
container.scrollTop()
},
500)
});
Here, #container
is an outer div
element that acts as our container.
#scrollToMe
is the target image we want to scroll to. It is nested inside the outer div
container.
Also, the outer div
container has other nested div
and p
elements. This outer div
container is scrollable and has a vertical scroll bar.
We add the click
event handler to the triggering button. The callback runs the animate
method on the container element.
It sets the container’s scrollTop
property to a new value. We calculate this new value with the following formula.
scrollToMe.offset().top - container.offset().top + container.scrollTop()
scrollToMe.offset().top
is the number of pixels from the top of the document to the target image element.container.offset().top
is the number of pixels from the top of the page to the containerdiv
element’s top.
But, there is a little catch. The container div
element is scrollable; if it is scrolled initially, it has moved down by that many pixels.
So, we have to add that factor of container.scrollTop()
into the parameter we pass to the scrollTop
method.
Tips to Keep in Mind
We have two important tips for scrolling to an element using the jQuery above methods.
Pro Tip 1:
The offset
and position
methods return a float
value, and if you expect a lot of zooms on your page, it might cause errors. So, you could use the parseInt
function to first convert the returned value into an int
value.
$('#clickButton').on('click', function() {
$([
document.documentElement, document.body
]).scrollTop($('#scrollToMe').parseInt(offset().top));
});
Pro Tip 2:
The animation()
also takes an easing function as the argument. This function decides how the animation executes.
The default value of this function is swing
, which provides a smooth and even animation effect. So, we can use this default value to get the smooth scrolling jQuery itself.
Two Quick Short-Cuts for jQuery ScrollTop
Finally, we have two quick short-cuts for two common use cases of jQuery scroll to an element.
jQuery Scroll to Top of Document Short-Cut
If you have a long web page with lots of content, you would want to provide a "scroll to top"
link at the bottom for easy navigation.
The short-cut code for such an anchor link is:
// this code scrolls to the top of the document with a click on the anchor
// element
$('#top').on('click', function() {
$(document.documentElement, document.body).animate({scrollTop: 0}, 500);
});
We set the target scrollTop
value of the animate
function to 0
- which scrolls us to the top of the page.
Add Hash/Fragment Identifier to the URL in jQuery Scroll to Element
If you want to add the fragment identifier of your target element to the URL in the address bar, you need to add just one line to our scroll to an anchor link code above.
// this code adds the fragment identifier/hash of the target
// element to the URL
// this is a reusable module to jQuery scrollTop from any internal link
$('a[href^="#"]').on('click', function(e) {
e.preventDefault();
let targetHash = this.hash;
let targetEle = $(targetHash);
$([
document.documentElement, document.body
]).animate({scrollTop: targetEle.offset().top}, 500, function() {
window.location.hash = targetHash;
});
})
The line window.location.hash = targetHash
is the code that provides this functionality.
You can see the element hash/fragment identifier scrollToMe
gets added at the end of the URL.
Be careful to include this code snippet as a callback to the animate
function to ensure it executes after the animation - in sync with JavaScript best practices.