How to Find Element With Data Attribute in jQuery
-
Find a Data Attribute Using jQuery
.find()
Method - Find a Data Attribute Using a CSS Attribute Selector
-
Find a Data Attribute Using the
.filter()
Method
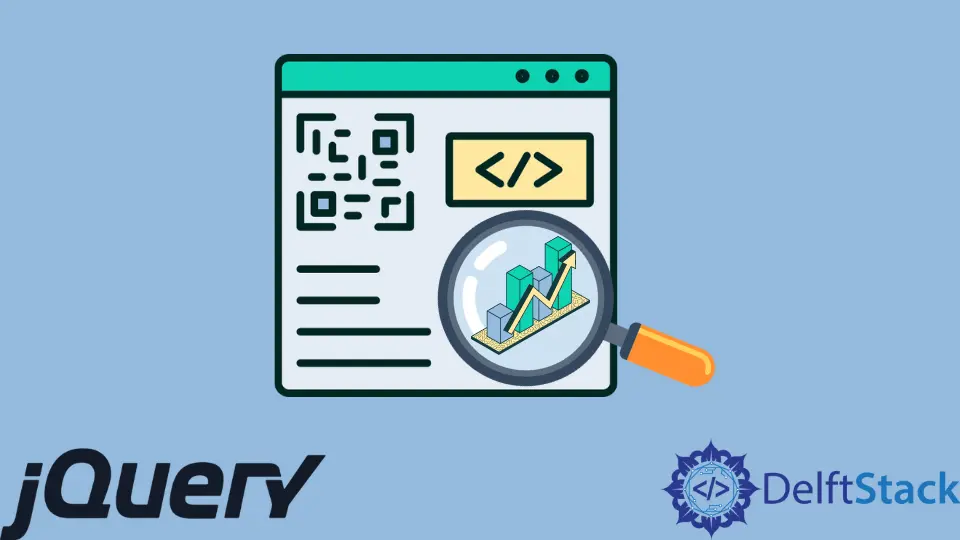
To search for an element with a data attribute using jQuery, you can use the .find()
method, the .filter()
method, or a CSS attribute selector.
This article teaches you how you can use them all using practical code examples.
Find a Data Attribute Using jQuery .find()
Method
In jQuery, the .find()
method allows you to search for an element on a web page using a CSS selector. Here, you can use it to find a data attribute if you write the right CSS selector.
From this, you can do what you want with elements that match the CSS selector. In the example below, we searched for data-name=Edison
and changed its font size to 3em
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-find-data-attr</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<ul>
<li data-name="Edison">Thomas Edison</li>
<li data-name="Einstein">Albert Einstein</li>
<li data-name="Rutherford">Ernest Rutherford</li>
</ul>
</main>
<script>
$(document).ready(function() {
$("ul").find("[data-name='Edison']").css('font-size', '3em');
});
</script>
</body>
</html>
Output:
Find a Data Attribute Using a CSS Attribute Selector
You can find a data attribute using a CSS attribute selector. But there are two ways to do this, depending on your HTML markup.
In the first example below, the list items have data attributes. So, you can use a CSS descendant selector, which consists of a tag selector and a CSS attribute selector.
The result is a pinpoint match of the required element, and you can apply CSS styles afterward.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-find-data-attr</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
body {
font-family: "Trebuchet MS", Verdana, sans-serif;
font-size: 1.2em;
}
</style>
</head>
<body>
<main>
<ul>
<li data-iso="MRT">Mauritania</li>
<li data-iso="LUX">Luxembourg</li>
<li data-iso="HRV">Croatia</li>
</ul>
</main>
<script>
$(document).ready(function() {
$("ul [data-iso='MRT'").css('color', 'magenta');
});
</script>
</body>
</html>
Output:
The second example below has a different HTML and jQuery code. First, the parent <ul>
element has the data attribute in the HTML.
This means the previous selector in the last example will not work. To solve this, you can remove the space between the <ul>
element and the CSS attribute selector.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02_01-jQuery-find-data-attr</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<ul data-viruses="computer-viruses">
<li>Mydoom</li>
<li>ILOVEYOU</li>
<li>WannaCry</li>
</ul>
</main>
<script>
$(document).ready(function() {
$("ul[data-viruses='computer-viruses']").css('font-style', 'italic');
});
</script>
</body>
</html>
Output:
Find a Data Attribute Using the .filter()
Method
The .filter()
method will match an HTML element using a CSS selector. With this, you can pass the CSS selector of an element and work with the result.
That’s what we’ve done in the code example below. Unlike previous examples, we add a CSS class using the jQuery .addClass()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-find-data-attr</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
.style-chef {
background-color: #1a1a1a;
color: #ffffff;
padding: 0.2em;
width: 40%;
}
</style>
</head>
<body>
<main>
<ul>
<li data-esolang="Befunge">Befunge</li>
<li data-esolang="Chef">Chef</li>
<li data-esolang="Whitespace">Whitespace</li>
</ul>
</main>
<script>
$(document).ready(function() {
$("li").filter('[data-esolang="Chef"]').addClass('style-chef');
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn