jQuery extend() Method
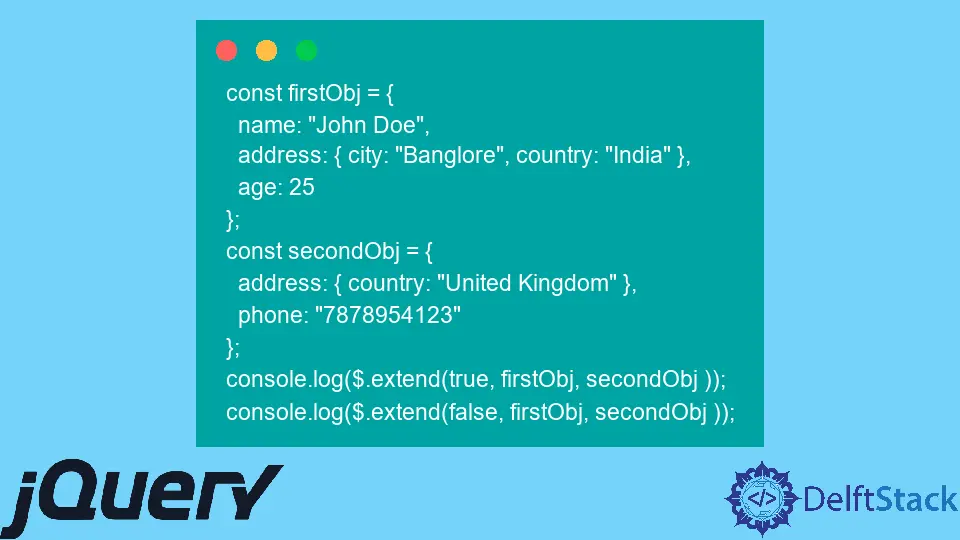
In today’s post, we’ll learn about the extend()
method in jQuery, which merges the properties of two or more objects.
extend()
in jQuery
jQuery provides an in-built method .extend()
that merges the contents of two or more objects into the target object. The properties of all objects are added to the target object if two or more objects are passed as an argument to .extend()
.
Null
or undefined
arguments are ignored while merging the objects.
Syntax:
jQuery.extend(deep, target, [object1])
- The
deep
is a Boolean parameter. The merge becomes recursive if it is specified astrue
. - The
target
is an object that will receive the new properties. - The
object1
is an object containing additional properties to merge in.
If only one argument is passed in the $.extend()
, it indicates that the target
argument is omitted. In such a case, the jQuery object itself becomes the target object.
This functionality lets you feature new capabilities/functionalities to the jQuery namespace
. This may benefit plugin authors who need to add new methods to jQuery.
By default, the $.extend()
performs a non-recursive merge. Suppose a property of the target object is itself an object or an array.
In that case, it will be completely overridden by a property with the identical key in the second or subsequent objects.
Passing true
as the 1st argument to the $.extend()
function, recursively merges the objects. However, properties inherited from the object’s prototype are copied.
Properties that are an object created via new MyCustomObject(args)
or built-in JavaScript types like Date
or RegExp
are not recreated and appear as simple objects in the resulting object or array.
Let’s try to understand it with the simple example below.
const firstObj = {
name: 'John Doe',
address: {city: 'Banglore', country: 'India'},
age: 25
};
const secondObj = {
address: {country: 'United Kingdom'},
phone: '7878954123'
};
console.log($.extend(true, firstObj, secondObj));
console.log($.extend(false, firstObj, secondObj));
In the above example, we have defined two objects with different properties. When you call the extend
method, with the first parameter as true
that is a deep
parameter, the properties of the first object recursively will be updated by the properties of the second object.
In our case, only the country will be updated by the second object, and other properties will be appended.
In the second case, when you call the extend
method with the second parameter false
, it will replace the properties of the first object with the properties of the second object.
If similar properties exist, then the properties of the first object will be replaced with the properties of the second object; otherwise, new properties of the second object will be created.
Try and run the above code snippet in any browser that supports jQuery, which will display the result below.
{
address: {
city: "Banglore",
country: "United Kingdom"
},
age: 25,
name: "John Doe",
phone: "7878954123"
}
{
address: {
country: "United Kingdom"
},
age: 25,
name: "John Doe",
phone: "7878954123"
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn