How to Convert String to Number in jQuery
- Convert a String to a Number in jQuery
-
Use
Number()
to Convert a String to a Number in jQuery -
Use the Unary Operator (
+
) to Convert String to Number in jQuery - Multiply by 1 to Convert String to Number in jQuery
-
Use
parseInt()
to Convert String to Number in jQuery -
Use
parseFloat()
to Convert String to Number in jQuery -
Use
Math.floor
to Convert String to Number in jQuery -
Use
Math.ceil
to Convert String to Number in jQuery
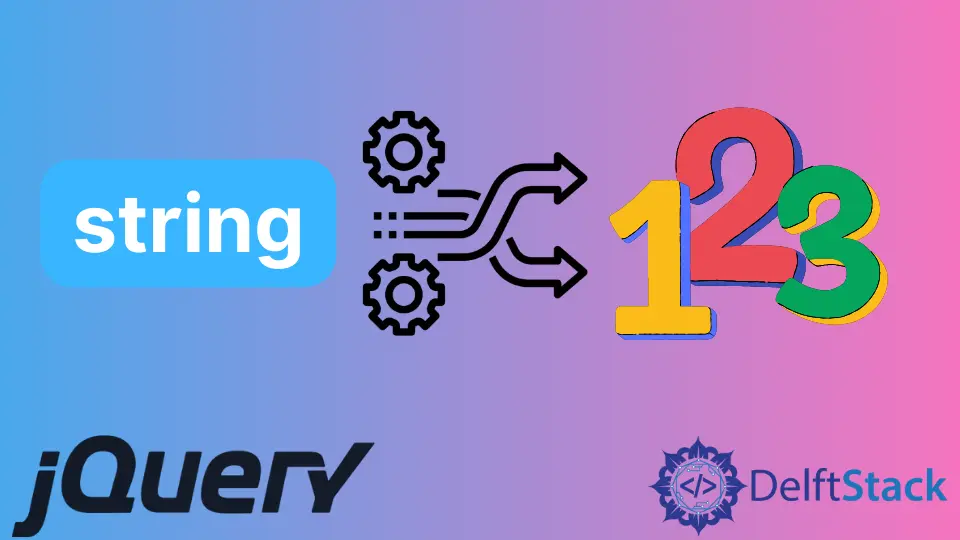
This tutorial demonstrates how to convert a string to a number using jQuery.
Convert a String to a Number in jQuery
There are several methods to convert a string to a number using JavaScript and jQuery. The JavaScript methods can be used along with jQuery code to convert a string to a number.
The methods to convert the string to a number include:
Number()
- Unary Operator (
+
) - Multiply by 1
parseInt()
parseFloat()
Math.floor()
Math.ceil()
All methods above can be used in jQuery to convert the string to a number.
Use Number()
to Convert a String to a Number in jQuery
The Number()
method is the easiest and most widely used method for converting a string to a number in jQuery/JavaScript. The Number()
method can convert strings with decimal numbers and strings without special characters or letters to numbers; otherwise, it will return NaN
.
The syntax for this method is:
var number1 = Number(StringNumber);
The StringNumber
is the number in the string that will be converted.
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Number()</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = Number('4422'); //it should return 4422
$("#Converted1").text(number1);
var number2 = Number('99,000') //it should return NaN (Not a Number)
$("#Converted2").text(number2);
var number3 = Number('13.00') //it should return 13
$("#Converted3").text(number3);
var number4 = Number('13.21') //it should return 13.21
$("#Converted4").text(number4);
var number5 = Number('1000 Delftstack') //it should return NaN (Not a Number)
$("#Converted5").text(number5);
var number6 = Number('Delftstack10') //it should return NaN (Not a Number)
$("#Converted6").text(number6);
</script>
</body>
</html>
The code above will convert the given numbers in strings to the number format using the Number()
method with jQuery. See output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: NaN
The conversion result for xx.00 format is: 13
The conversion result for xx.xx format is: 13.21
The conversion result for "xxxx Delftstack" format is: NaN
The conversion result for "Delftsackxxxx" format is: NaN
Use the Unary Operator (+
) to Convert String to Number in jQuery
The unary operator +
can be used before any string to convert it to a number which is the fastest way to convert a string to a number in jQuery or JavaScript. The unary operators operate on one operand.
The syntax for this method is:
var number = +'xx,xxx'
Where xx,xxx
is a number string and +
is a unary operator.
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Unary Operator</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.00 format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = +'4422';
$("#Converted1").text(number1);
var number2 = +'99,000'
$("#Converted2").text(number2);
var number3 = +'13.00'
$("#Converted3").text(number3);
var number4 = +'13.21'
$("#Converted4").text(number4);
var number5 = +'100.01 Delftstack'
$("#Converted5").text(number5);
var number6 = +'Delftstack10'
$("#Converted6").text(number6);
</script>
</body>
</html>
The unary operator works similarly to the Number()
method. It will accept any special character or letter in the number string for those and return NaN
.
See the output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: NaN
The conversion result for xx.00 format is: 13
The conversion result for xx.xx format is: 13.21
The conversion result for "xxxx Delftstack" format is: NaN
The conversion result for "Delftsackxxxx" format is: NaN
Multiply by 1 to Convert String to Number in jQuery
In jQuery or Javascript, when we multiply a string by a number, it will try to convert the result to a number. In this case, we can multiply any string by 1 to convert it to a number, and if it is possible, the string will be converted to a number.
The syntax for this method is:
var number = 'xx,xxx' * 1
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Multiply by One</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.00 format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = '4422' * 1;
$("#Converted1").text(number1);
var number2 = '99,000' * 1;
$("#Converted2").text(number2);
var number3 = '13.00' * 1;
$("#Converted3").text(number3);
var number4 = '13.21' * 1;
$("#Converted4").text(number4);
var number5 = '100.01 Delftstack' * 1;
$("#Converted5").text(number5);
var number6 = 'Delftstack10' * 1;
$("#Converted6").text(number6);
</script>
</body>
</html>
This method will also work similarly to the unary operator and the Number()
method. See the output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: NaN
The conversion result for xx.00 format is: 13
The conversion result for xx.xx format is: 13.21
The conversion result for "xxxx Delftstack" format is: NaN
The conversion result for "Delftsackxxxx" format is: NaN
Use parseInt()
to Convert String to Number in jQuery
The parseInt()
method can convert strings to integers using jQuery. The parseInt()
can convert a string to a whole number which means it will ignore the decimal or any other special characters.
The syntax for this method is:
var number = parseInt(StringNumber)
StringNumber
is the string that will be converted to a number.
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Parse Int</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.00 format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = parseInt('4422');
$("#Converted1").text(number1);
var number2 = parseInt('99,000')
$("#Converted2").text(number2);
var number3 = parseInt('13.00')
$("#Converted3").text(number3);
var number4 = parseInt('13.21')
$("#Converted4").text(number4);
var number5 = parseInt('1000 Delftstack')
$("#Converted5").text(number5);
var number6 = parseInt('Delftstack10')
$("#Converted6").text(number6);
</script>
</body>
</html>
The code above will ignore the decimal and other characters in the string if it finds a separate number.
See the output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: 99
The conversion result for xx.xx format is: 13
The conversion result for xx.xx format is: 13
The conversion result for "xxxx Delftstack" format is: 1000
The conversion result for "Delftsackxxxx" format is: NaN
Use parseFloat()
to Convert String to Number in jQuery
The parseFloat()
method is used to convert a string to a number with a decimal. This method works similarly to parseInt
, but it returns a number with a decimal.
The syntax for this method is:
var number = parseFloat(StringNumber)
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Parse Float</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.00 format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = parseFloat('4422');
$("#Converted1").text(number1);
var number2 = parseFloat('99,000')
$("#Converted2").text(number2);
var number3 = parseFloat('13.00')
$("#Converted3").text(number3);
var number4 = parseFloat('13.21')
$("#Converted4").text(number4);
var number5 = parseFloat('100.01 Delftstack')
$("#Converted5").text(number5);
var number6 = parseFloat('Delftstack10')
$("#Converted6").text(number6);
</script>
</body>
</html>
The code will convert the string to a number with a decimal wherever it finds a decimal in the string. See the output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: 99
The conversion result for xx.00 format is: 13
The conversion result for xx.xx format is: 13.21
The conversion result for "xxxx Delftstack" format is: 100.01
The conversion result for "Delftsackxxxx" format is: NaN
Use Math.floor
to Convert String to Number in jQuery
The Math.floor
method will return only the integer part of the number when converting a string to a number in jQuery. The method takes one parameter.
The syntax of Math.floor
is:
var number = Math.floor(StringNumber)
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Math Floor</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.00 format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = Math.floor('4422');
$("#Converted1").text(number1);
var number2 = Math.floor('99,000')
$("#Converted2").text(number2);
var number3 = Math.floor('13.00')
$("#Converted3").text(number3);
var number4 = Math.floor('13.21')
$("#Converted4").text(number4);
var number5 = Math.floor('100.01 Delftstack')
$("#Converted5").text(number5);
var number6 = Math.floor('Delftstack10')
$("#Converted6").text(number6);
</script>
</body>
</html>
The code above will only convert the strings with the correct number format. It doesn’t accept the special character or letter in the string, just like the Number()
method.
See the output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: NaN
The conversion result for xx.00 format is: 13
The conversion result for xx.xx format is: 13
The conversion result for "xxxx Delftstack" format is: NaN
The conversion result for "Delftsackxxxx" format is: NaN
Use Math.ceil
to Convert String to Number in jQuery
The Math.ceil
is similar to the Math.floor
method, but it will only round the given number to the next largest whole number.
The syntax for this method is:
var number = Math.ceil(StringNumber)
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Math Ceil</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div class="html-code-output">
<div id="div1">
<p>The conversion result for xxxx format is: <b id="Converted1"></b></p>
<p>The conversion result for xx,xxx format is: <b id="Converted2"></b></p>
<p>The conversion result for xx.00 format is: <b id="Converted3"></b></p>
<p>The conversion result for xx.xx format is: <b id="Converted4"></b></p>
<p>The conversion result for "xxxx Delftstack" format is: <b id="Converted5"></b></p>
<p>The conversion result for "Delftsackxxxx" format is: <b id="Converted6"></b></p>
</div>
</div>
<script>
var number1 = Math.ceil('4422');
$("#Converted1").text(number1);
var number2 =Math.ceil('99,000')
$("#Converted2").text(number2);
var number3 = Math.ceil('13.00')
$("#Converted3").text(number3);
var number4 = Math.ceil('13.21')
$("#Converted4").text(number4);
var number5 = Math.ceil('100.01 Delftstack')
$("#Converted5").text(number5);
var number6 = Math.ceil('Delftstack10')
$("#Converted6").text(number6);
</script>
</body>
</html>
The code above will convert the given string to a number by rounding up the decimal number. It will not accept any special characters or letters in the string. See output:
The conversion result for xxxx format is: 4422
The conversion result for xx,xxx format is: NaN
The conversion result for xx.00 format is: 13
The conversion result for xx.xx format is: 14
The conversion result for "xxxx Delftstack" format is: NaN
The conversion result for "Delftsackxxxx" format is: NaN
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook