How to Change Background Color of an Element in jQuery
-
jQuery Change Background Color Using the
queue()
API -
jQuery Change Background Color Using the
hide()
API
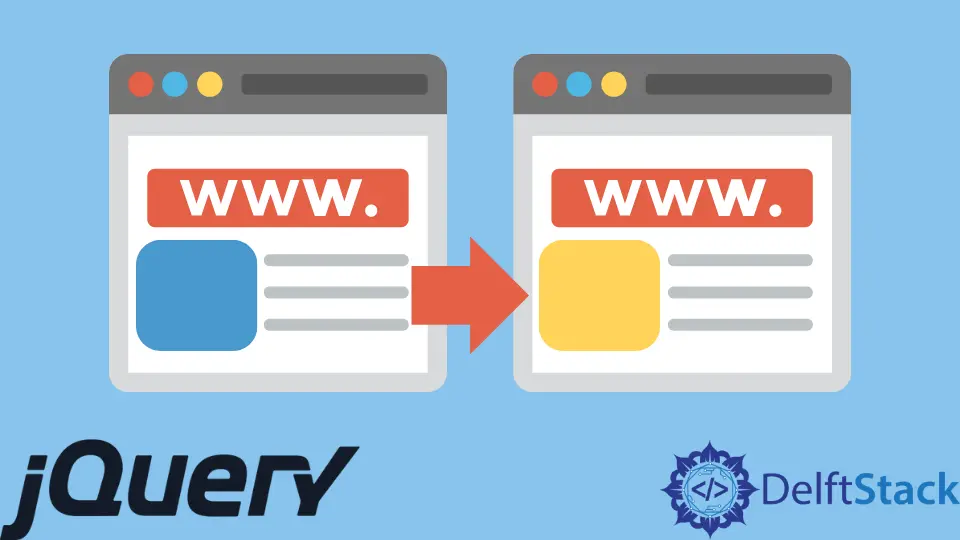
This article teaches you how to use jQuery to change the background color of an element on mouseover. We’ll achieve this using two jQuery APIs which are queue()
and hide()
.
jQuery Change Background Color Using the queue()
API
This first example will change the background color before and after animation. Here, you’ll need the queue()
API because the css()
API alone will not work as intended.
That’s because it has an instant effect on the attached element. By using the queue()
API, you can pass it a function that fires an effect after the result of the css()
API.
This means the initial color in the css()
will have an effect at the start of the animation. Afterward, you can change the initial color to another color with the queue()
API.
The following is the pseudocode of the process.
- Mouse over the button activates the initial color in the
css()
API. - The element fades away.
- The element shows again.
- Finally, its color changes to another color defined in the
queue()
API.
The following code is the implementation of the pseudocode. What follows is the result in a web browser.
In the result, you’ll observe that the animation does not occur the second time you mouse over the button. We’ll address that in the next example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-change-background-color-with-queue-API</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
main { border: 3px solid #1a1a1a; padding: 1.2em; display: grid; }
button { align-self: center; padding: 1.2em; cursor: pointer; }
p { font-size: 5em; }
</style>
</head>
<body>
<main>
<p id="random_big_text">A big random text.</p>
<button>Move your mouse here.</button>
</main>
<script>
$(document).ready(function() {
$("button").mouseover(function() {
let random_big_text = $("#random_big_text");
random_big_text.css("background-color", "#ffff00");
random_big_text.hide(1500).show(1500);
random_big_text.queue(function() {
random_big_text.css("background-color", "#ff0000");
});
});
});
</script>
</body>
</html>
Output:
jQuery Change Background Color Using the hide()
API
Using jQuery hide()
API to change background color builds on our first example where we used the queue()
API. The result is the same with two differences; we use API chaining, and the effect works every time.
As a result, you can mouse over the button at the end of each animation, and the process will start over. The following code shows you how it’s done; what follows is the result in a web browser.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-change-background-color-with-hide-API</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
main { border: 3px solid #1a1a1a; padding: 1.2em; display: grid; }
button { align-self: center; padding: 1.2em; cursor: pointer; }
p { font-size: 5em; }
</style>
</head>
<body>
<main>
<p id="random_big_text">A big random text.</p>
<button>Move your mouse here.</button>
</main>
<script>
$(function(){
$("button").mouseover(function(){
let random_big_text = $("#random_big_text");
random_big_text.stop()
.css("background-color","#ffff00")
.hide(1500, function() {
random_big_text.css("background-color","#ff0000")
.show(1500);
});
});
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn