How to Capitalize First Letter in jQuery
-
Use
toUpperCase()
With thereplace()
Method to Capitalize the First Letter in jQuery -
Use
toUpperCase()
With thesubstring()
Method to Capitalize the First Letter in jQuery -
Use the
css()
Method to Capitalize the First Letter in jQuery
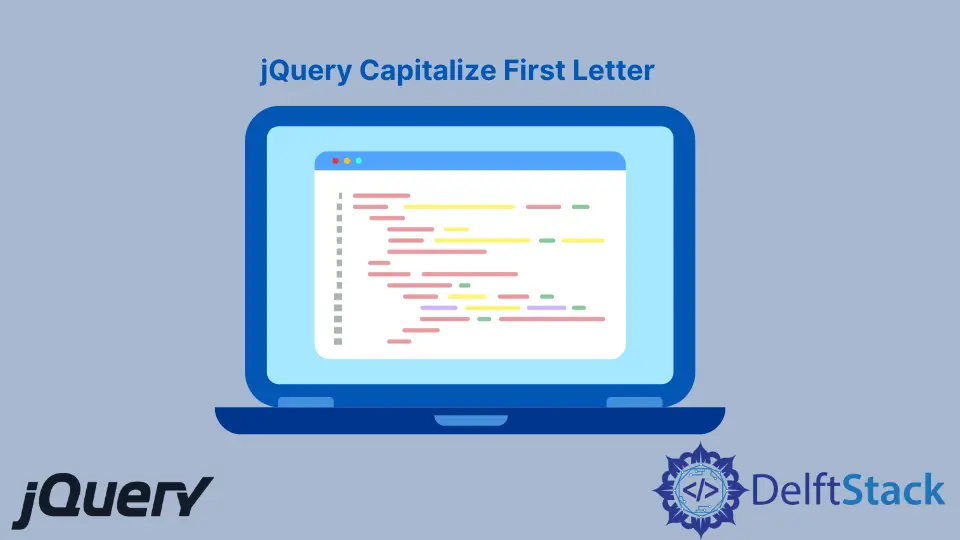
This tutorial demonstrates how to capitalize the first letter of a string using jQuery.
We can capitalize the first letter of a string in jQuery by using the toUpperCase()
method with helper methods which help to break the string or replace the first letter. This tutorial demonstrates different methods to capitalize the first letter of a string in jQuery.
Use toUpperCase()
With the replace()
Method to Capitalize the First Letter in jQuery
The toUpperCase()
method can capitalize any text, but to capitalize the first letter of a string, we need another method to help the toUpperCase
method. We use the replace method of Javascript, which will help us to capitalize the first letter of the given string; the syntax for these methods is:
String.toUpperCase()
- It will convert the given whole string to upper case.String.replace(/\b[a-z]/g, function(NewString){}
- This method will take two parameters, one is the pattern to identify the first letter, and the second is a method with the string to be replaced.
Let’s try an example to capitalize the first letter of a given string using jQuery:
<!doctype html>
<html lang="en">
<head>
<title>jQuery Capitalize First Letter</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('#DemoButton').click(function(){
var OriginalString = $('#DemoString').text();
CapitalizedString = OriginalString.replace(/\b[a-z]/g, function(DemoStr) {
return DemoStr.toUpperCase();
});
$("#output").text(CapitalizedString);
});
});
</script>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
</head>
<body>
<div id = "Main">
<h2>jQuery Uppercase First Letter of Text</h2>
<p id="DemoString">hello! this is delftstack.com</p>
<h3 id= "output"></h3>
<button type="button" id="DemoButton">Click to Uppercase the First Letter</button>
<div>
</body>
</html>
The code above will capitalize the first letter of each word in the string. See the output:
Use toUpperCase()
With the substring()
Method to Capitalize the First Letter in jQuery
As we can see, the toUpperCase()
method with the replace()
method will capitalize each word of the string, but to capitalize only the first letter of the first word, we can use the substring()
method instead of the replace()
method. The syntax using the substring()
is:
OriginalString.substring(0, 1).toUpperCase() + OriginalString.substring(1);
Where the substring(0,1)
will break the string and the substring(1)
will rejoin the string after capitalization of the first letter of the string. Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<title>jQuery Capitalize First Letter</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('#DemoButton').click(function(){
var OriginalString = $('#DemoString').text();
var CapitalizedString = OriginalString.substring(0, 1).toUpperCase() + OriginalString.substring(1);
$('#output').text(CapitalizedString);
});
});
</script>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
</head>
<body>
<div id = "Main">
<h2>Uppercase First Letter</h2>
<p id="DemoString">hello! this is delftstack.com</p>
<h3 id= "output"></h3>
<button type="button" id="DemoButton">Click to Uppercase the First Letter</button>
<div>
</body>
</html>
The code above will capitalize only the first letter for the first word of a given string. See the output:
Use the css()
Method to Capitalize the First Letter in jQuery
We can also use the jQuery built-in method css()
to capitalize the first letter of the word. We can use the CSS property text-transform: capitalize;
to keep a particular element to the first letter capital style.
<!doctype html>
<html lang="en">
<head>
<title>jQuery Capitalize First Letter</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('#DemoButton').click(function(){
$('#DemoString').css("text-transform", "capitalize");
});
});
</script>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
</head>
<body>
<div id = "Main">
<h2>Uppercase First Letter</h2>
<p id="DemoString">hello! this is delftstack.com</p>
<button type="button" id="DemoButton">Click to Uppercase the First Letter</button>
<div>
</body>
</html>
The code above capitalizes the first letter of each word in the string. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook