How to Add Style to an Element Using jQuery
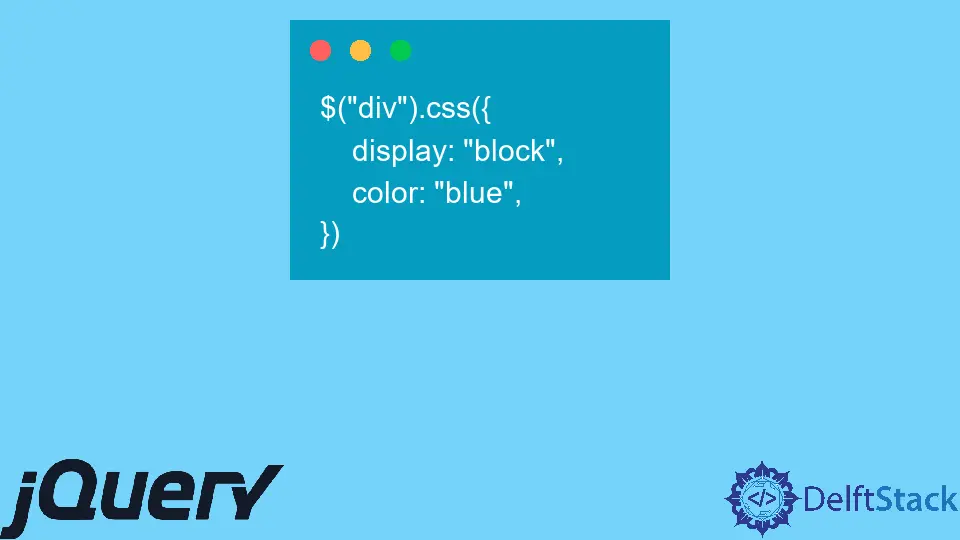
Today’s post will discuss adding style to an element using jQuery.
Use .css()
to Add Style to an Element Using jQuery
jQuery provides a simple way to add style to an element using .css()
to set one or more CSS properties for a set of matched elements. This approach can take a property name and value as separate parameters or a single object of key-value pairs.
Syntax:
.css(propertyName, value)
Where:
propertyName
is a string that represents the CSS property name.value
is a string or number representing the value to set for the property.
jQuery can interpret CSS and DOM formatting of multi-word properties equally. For example, jQuery understands the correct value for:
.css({'background-color': '#fefefe', 'border-right': '2px solid #eee'})
and
.css({backgroundColor: '#fefefe', borderRight: 'solid 2px #eee'})
In DOM notation, citation marks around property names are optionally available; and in CSS notation, they are required due to the hyphen inside the name. While .css()
is used as a setter, jQuery modifies the style property of an element.
Updating/setting the value of a style property to an empty string, e.g., $( "#div-1" ).css( "color", "" )
, removes the property from an element if it has already been applied directly, either in the HTML style attribute, via jQuery’s .css()
method, or by direct DOM manipulation of the style property.
As a result, the element’s style for that property reverts to the applied value. Therefore, this method can cancel any style changes you have previously made. Moreover, .css()
doesn’t support important declarations.
The setter .css()
automatically takes care of prefixing the property name. You can find more information about the .css()
documentation for .css()
.
Let’s understand it with the following example.
Example code (HTML):
<div>Hello World!</div>
Example code (JavaScript):
$('div').css({
display: 'block',
color: 'blue',
})
We have described a div
tag in the above example code without any styling applied. Through jQuery, we are setting the color of the div
as blue
and display as block
.
Attempt to run the above code snippet in any browser that supports jQuery. It’s going to display the below result.
Before adding a CSS property, we have the following output:
After adding a CSS property, this becomes:
You can view the live demo of the above example code here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn