How to Empty Input Field With JQuery
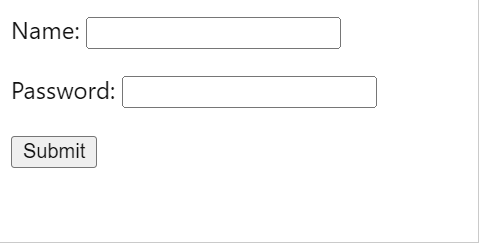
After validation of a form, we often need to refresh the page when the data is posted or saved. This is necessary to ensure safety, as the following user might grab the credentials of the previous one.
In a well-organized webpage and form, we ensure that the input fields are reset after the proper submission. Unfortunately, even this convention is followed so strictly that, after a limited amount of session time, input fields get invalid, and a refresh is required.
This article will use a code example to learn a widely used approach to clear an input field. The plus point is that it is easy to implement.
Clear Input Field With Defined class
& input[type]
Here, we will set up two input fields; one with the text
type and the other with the password
type. So, what we will do is, make a button that will ensure a submission.
Whenever the button is clicked, the specific input field will get cleared. So let’s jump up to the code fence.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<title>array loop through</title>
</head>
<body>
<form method="" action="">
<label for="name">Name: <input type="text" name="name" class="input"/></label>
<p></p>
<label for="pass">Password: <input type="password" name="pass"></label>
<p></p>
<input type="submit" value="Submit" class="button"/>
</form>
</body>
<script>
$(document).ready(function() {
$("input[type='text']").val("");
$(".button").on("click", function(event) {
event.preventDefault();
$(".input").val("");
});
});
</script>
</html>
Output:
As it can be seen, in the script, the jQuery("input[type='text']")
was initiated and it was followed by the .val("")
.
This depicts that the input field will be blank in the first place, and after the submission, it will be reset. In this code, we have used the class="input"
to complement the later clearance of the input field text.
Clear Input Field With input[type]
as a Selector
Here, we will just replace $("input[type="password"]")
with $(:password)
. And this selector
will perform the same task as before. Let’s jump to the code snippet.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<title>array loop through</title>
</head>
<body>
<form method="" action="">
<label for="name">Name: <input type="text" name="name" class="input"/></label>
<p></p>
<label for="pass">Password: <input type="password" name="pass"></label>
<p></p>
<input type="submit" value="Submit" class="button"/>
</form>
</body>
<script>
$(document).ready(function() {
$("input[type='text']").val("");
$(".button").on("click", function(event) {
event.preventDefault();
$(".input").val("");
});
});
$(document).ready(function() {
$(":password").val("");
$(".button").on("click", function(event) {
event.preventDefault();
$(":password").val("");
});
});
</script>
</html>
Output: