How to Use OpenCV JavaScript to Displaying an Image
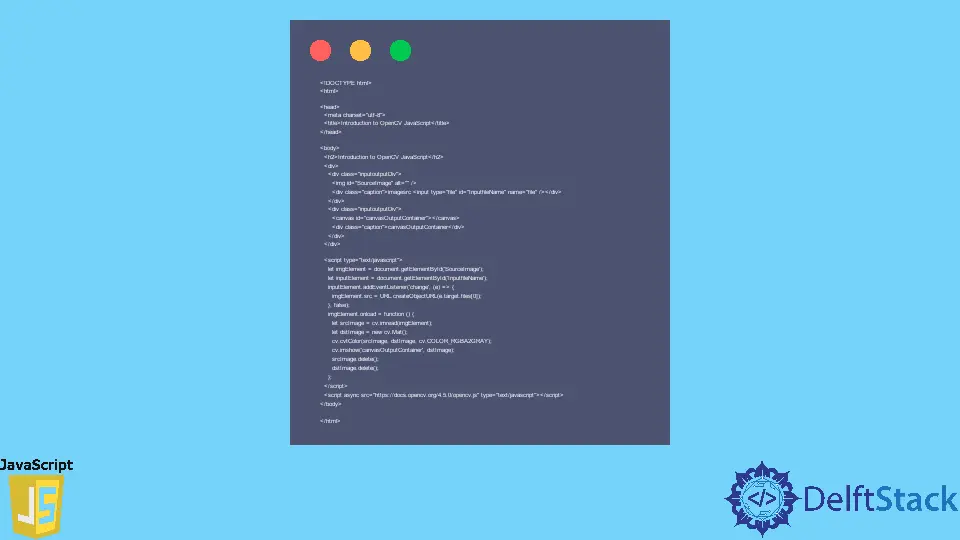
This tutorial will discuss displaying an image on a webpage using OpenCV in JavaScript.
Use OpenCV JavaScript to Display an Image
We can read an image using the imread()
function of OpenCV in JavaScript. We must pass the name
of the canvas
element, its id
or the name
of an image
element or its id
inside the imread()
function to read the image.
OpenCV JavaScript saves the image as a cv.Mat
type, which belongs to the n-dimensional array class, and it can store data to different data types like double
, float
, etc. The imread()
function returns a cv.Mat
type which contains the image with RGBA channel.
After reading the image, we can perform any image processing task on it, like changing the color space of the image, and after that, we can display it on the webpage using the imshow()
function of OpenCV.
We need a graphical container to display an image. We can use the <canvas>
tag of HTML to make a graphical container to display the image.
The imshow()
function has two input arguments. The first is the name
of the canvas
element or its id
, and the second is the variable containing the image we want to display on the webpage.
If the image passed inside the imshow()
is 8-bit unsigned, it will be displayed as it is.
If an image is a 16-bit unsigned or 32-bit integer, the pixels of the image will be divided by 256, and then it will be displayed so that the pixel values fall in the acceptable range.
If the image is a 32-bit floating point, the pixel will be multiplied by 255, map it in the range of 0 - 255, and then display the image.
After displaying the image, we can delete the image variable using the delete()
function. For example, let’s read a color image, convert it into grey, and then display it on the webpage along with the original image.
See the example code below.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Introduction to OpenCV JavaScript</title>
</head>
<body>
<h2>Introduction to OpenCV JavaScript</h2>
<div>
<div class="inputoutputDiv">
<img id="SourceImage" alt="" />
<div class="caption">imagesrc <input type="file" id="InputfileName" name="file" /></div>
</div>
<div class="inputoutputDiv">
<canvas id="canvasOutputContainer"></canvas>
<div class="caption">canvasOutputContainer</div>
</div>
</div>
<script type="text/javascript">
let imgElement = document.getElementById('SourceImage');
let inputElement = document.getElementById('InputfileName');
inputElement.addEventListener('change', (e) => {
imgElement.src = URL.createObjectURL(e.target.files[0]);
}, false);
imgElement.onload = function () {
let srcImage = cv.imread(imgElement);
let dstImage = new cv.Mat();
cv.cvtColor(srcImage, dstImage, cv.COLOR_RGBA2GRAY);
cv.imshow('canvasOutputContainer', dstImage);
srcImage.delete();
dstImage.delete();
};
</script>
<script async src="https://docs.opencv.org/4.5.0/opencv.js" type="text/javascript"></script>
</body>
</html>
Output:
In the above code, we used the cvtColor()
function of OpenCV to convert the color image to grey.
The first argument of the cvtColor()
function is a variable containing the color image, and the second argument is the variable in which we want to store the output image.
The third argument is used to specify the conversion of color spaces like cv.COLOR_RGBA2GRAY
will convert the input image’s RGBA color space to grey.
In the above code, we used the onload()
function of HTML, which will be executed when we load the input image and then the code inside it will be executed and display the image.
We have also linked the opencv.js
file to the .html
file using the file link, which will require the internet to lead the file, but we can also download it and then link it with it the .html
file.
To run the above code, we have to store it in a .html
file and then open the file with a web browser.