How to Set Style of an HTML Form Element in JavaScript
- Add Style to an HTML Element in JavaScript
- Apply Style to Elements With the Class Name in JavaScript
- Apply Style to Tags in JavaScript
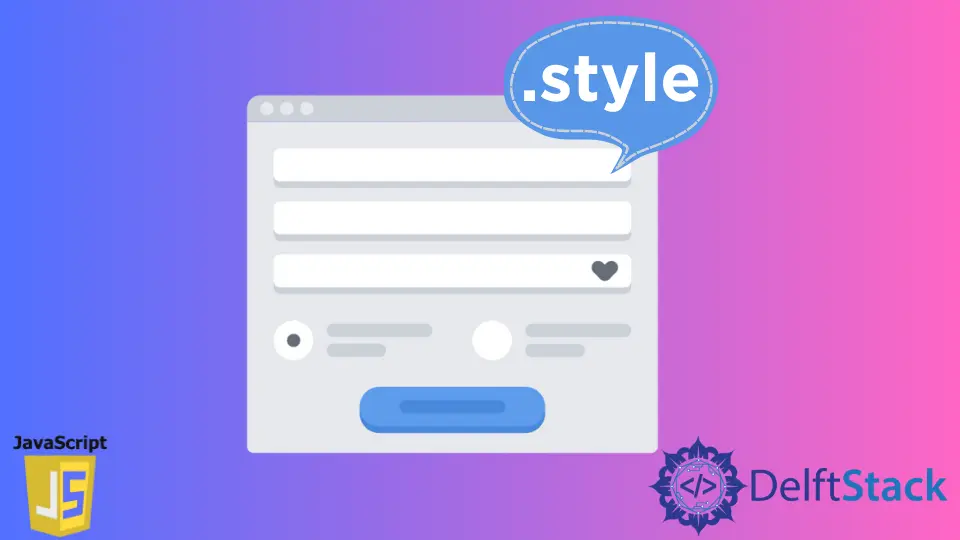
One of the use cases for setting custom style to an HTML element at run time is while performing validations in forms. We will need to highlight the element in red and display a validation text like Username field cannot be left empty
. There are various ways to set style parameters to an HTML element at run time with JavaScript. We can add new styles and change the existing style too. Following are a few ways in which we can set CSS style to an HTML element using JavaScript.
Add Style to an HTML Element in JavaScript
We can change the style of an element with the JavaScript .style
attribute. Before it, we need to query the HTML element and set the .style
attribute. There are various ways to query an HTML element in JavaScript, which are as follows, but the most commonly used is the document.getElementById()
method.
getElementById()
: of the document interface in JavaScript is used to query an HTML element based on its id. Usually, while designing a webpage, the developers assign a unique id to the HTML elements. ThegetElementById()
returns the element that has the id as specified in the parameters of thegetElementById()
function. If there are more elements with the same id, then the first element with the specified id is returned.getElementsByClassName()
: Another way to query an HTML element is with its assigned class name. These are CSS class names usually used in decorating the element. As more than one HTML element can have the same CSS class names, the function returns an array of HTML elements. We can choose to alter or add the specific style to an element or all the elements returned bygetElementsByClassName()
function.getElementsByName()
: Similar to the way we select an HTML element with its CSS class name, thegetElementsByName()
function uses the name attribute to filter the expected HTML element. In this case, there may be more than one HTML element with the same names. Hence, it returns an array of HTML elements having thename
attribute the same as the one passed in its parameters.getElementsByTagName()
: is used in cases we need to select particular HTML tags like the<div>
,<p>
,<li>
tags etc. It makes it convenient to apply a style or set of styles to all the<div>
elements in one go. ThegetElementsByTagName()
function returns an array of elements having tag name passed in the parameter to the function. There may be more than one HTML tag whose styles may need altering in a single shot.querySelector()
: is like a master method of retrieving an element. To understand the usage of parameters accepted by this JavaScript function, think of using selectors for querying HTML elements in CSS. We can use combinations of tag names likedocument.querySelector("div span")
(to select all the span inside the divs),document.querySelector("#elementID")
(to query an element based on its id),document.querySelector(".sample-css")
(to query for elements that have the CSS class namesample-css
) etc. It returns the first element that satisfies the criteria passed as a parameter to the function.querySelectorAll
: is similar toquerySelector()
in all aspects except the return type. Instead of returning just the first element that satisfies the criteria as in thequerySelector()
function,querySelectorAll()
function returns all the HTML elements that meet the conditions mentioned in parameters to the method. Once an HTML element is selected, we use the.style
attribute, followed by the style name that we wish to change or add. For instance, in the following code, we try to change the background colour of an element having id asusername
.
<div id="username">
<label>User Name: </label>
<input type="text" id="username" name="UserName">
</div>
window.onload = function() {
document.getElementById('username').style.backgroundColor = 'green';
}
If the selected HTML element has the style attribute (for example, the background colour) already set, then the following code will change it. And if it does not have any style attribute, then a new style is added at run time by the JavaScript code.
Apply Style to Elements With the Class Name in JavaScript
We can query for an element using the class name with the JavaScript function getElementsByClassName()
. Once the element(s) are selected, we can add or change the style of the elements with the .style
attribute. This method is applied when we need to have additional CSS attributes in that particular CSS class of the element. The following code depicts the usage.
<div id="username" class="blue-bg">
<label>User Name: </label>
<input type="text" id="username" name="UserName">
</div>
window.onload = function() {
document.getElementsByClassName('blue-bg')[0].style.backgroundColor = 'green';
}
getElementsByClassName("blue-bg")
function returns an array of elements. Hence, it is necessary to select the index of the element on which we intend to apply the styles. If the style attribute is already set by some CSS class or by inline style, the .style
attribute will overwrite it. If not set already, it will add a new attribute to set the style of the targetted HTML element.Apply Style to Tags in JavaScript
In case, we must add style to all the div
or all the span
, then we can make use of the .getElementsByTagName()
function. It queries the DOM for the tag names. It returns a collection of HTML elements that satisfy the tag name passed as a parameter. Once the function returned the HTML elements, we can apply the style attribute to that element by using its index. The following code elaborates it.
<div id="output">Hello World!!</div>
<form name="LoginForm">
<div id="username" class="blue-bg">
<label>User Name: </label>
<input type="text" id="username" name="UserName">
</div>
<div>
<label>Password: </label>
<input type="password" name="password">
</div>
<div>
<label>Description: </label>
<textarea name="description"></textarea>
</div>
<div>
<label>Occupation: </label>
<select id="occupationSelect" autofocus>
<option>Student</option>
<option>Teacher</option>
<option>Engineer</option>
</select>
</div>
</form>
window.onload = function() {
document.getElementsByTagName('div')[0].style.backgroundColor = 'green';
}
On executing the above code, the first div
(<div id="output">Hello World!!</div>
) gets its background color set as green. Note that as we are targetting the first div
, we use the index as 0
(document.getElementsByTagName("div")[0]
). Similarly, we can assign style atributes to the body tag too with document.getElementsByTagName("body")[0].style.backgroundColor = "green"
. As only one body tag is allowed in an HTML document, we can directly query the body element and apply style attributes to it with .body
attribute of the document
interface. Hence the code will look like document.body.style.backgroundColor = "green"
.