How to Set Focus of an HTML Form Element in JavaScript
- Set Focus in Input With JavaScript
- Set Focus in Textarea With JavaScript
- Set Focus in Select Box or Dropdown With JavaScript
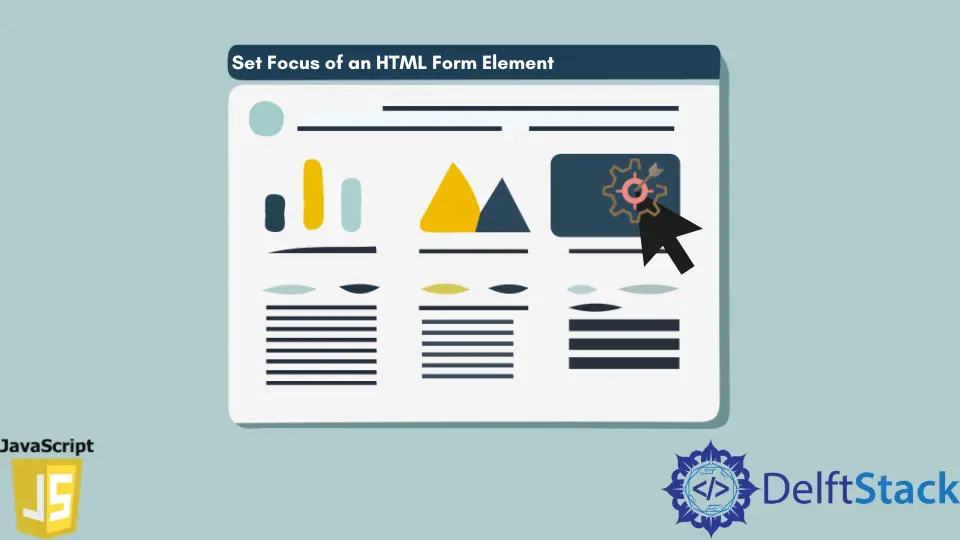
Focusing on a field for user input is a UI/UX feature that allows one to continue typing in a field without clicking on it. We may need to dynamically apply focus on a form field, especially if the form is long and has many input fields. If a form field has a validation error, it will be hard for the user to figure out the field in such a scenario. So having a focus set to the field in javascript while validating and scrolling automatically to the error field indicates the error directly to the user instead of wasting time figuring out where the error was. In JavaScript we have the .focus()
method. Let us see how we can apply these to the following.
Set Focus in Input With JavaScript
For focussing on an input field, we first need to select the field. There are various methods to do so. We can use any of the following methods.
document.getElementById()
: Queries the element based on its id. Usually ids are unique hence, thegetElementById()
function uniquely returns the element. If there are more elements with the same id, then it returns the first element with the specified id.document.getElementsByClassName()
: Selects the element based on its class name. It usually returns an array of elements qualifying the criteria.querySelector()
: JavaScript method selects the element based on its id, class name, or even with the tag of the HTML element. The function returns a single HTML element that satisfies the criteria passed as a parameter to it. In this article, we will be usinggetElementById()
function for simplicity of representation. Once we have the element selected, we can set focus on the element using the.focus()
method. Following is the syntax for.focus
.
document.getElementById('input-id').focus();
Set Focus on an Input Field
The following code sets focus on the input field on page load.
<input id="username-input" type="text" name="username">
window.onload = function() {
document.getElementById('username-input').focus();
}
Set Focus on an Input Field in an HTML Form
If the input element is part of a form, instead of querying the element using the getElementById()
, we can use the form name reference. The syntax for the usage is as follows:
document.<Form name>.<Field name>.focus();
For example, assume we have a login form with an input field. We can set the focus of the input field by applying the following code. The code will set focus on the user name input field. There are few things to take care of while using the form name and the field name for the following code to work.
<form name="LoginForm">
<div>
<label>User Name: </label>
<input type="text" name="UserName">
</div>
<div>
<label>Password: </label>
<input type="password" name="password">
</div>
</form>
window.onload = function() {
document.LoginForm.UserName.focus();
}
Remarks
- While setting the focus of an input in a form, we must assign the name attribute to the HTML form and the field we are targetting. If no name is specified, it will be hard to query for the element.
- This method does not support tag names having hyphens in this method. Hence the name assigned to the HTML form or the element should either be the camel case (like
UserName
) or lower case (like username). - For lengthier forms with many fields like the input, text areas, etc., it is good to set the scroll attribute to the element so that the browser will auto-scroll the focussed HTML element into view. It is an intended behavior expected by the user. Else he will end up wondering why the form is invalid.
document.getElementById("myButton").focus({preventScroll:false});
Set Focus in Textarea With JavaScript
We can use the .focus()
function to set focus to a text area. Usually, to focus in a text area we use document.getElementById("my-text-area").focus()
. It is similar to using the .focus()
function in an input field. If we intend to use the text area inside an HTML form tag, then we can apply the form name reference along with the text area name to focus on it. Refer to the following code.
<form name="LoginForm">
<div>
<label>User Name: </label>
<input type="text" name="UserName">
</div>
<div>
<label>Password: </label>
<input type="password" name="password">
</div>
<div>
<label>Description: </label>
<textarea name="description"></textarea>
</div>
</form>
window.onload = function() {
document.LoginForm.description.focus();
}
In this method, we must assign a name to the form and the target HTML element. The rules for naming the elements are the same as that of the input field.
Set Focus in Select Box or Dropdown With JavaScript
The .focus()
method works on select boxes or dropdown. If used within a form, then the document.FormName.SelectBoxName.focus()
can be used to set focus to the dropdown. Following is the code elaborating on the usage.
<form name="LoginForm">
<div>
<label>User Name: </label>
<input type="text" name="UserName">
</div>
<div>
<label>Password: </label>
<input type="password" name="password">
</div>
<div>
<label>Description: </label>
<textarea name="description"></textarea>
</div>
<div>
<label>Occupation: </label>
<select id="occupationSelect" autofocus>
<option>Student</option>
<option>Teacher</option>
<option>Engineer</option>
</select>
</div>
</form>
window.onload = function() {
document.LoginForm.occupationSelect.focus();
}