Rock Paper and Scissors in JavaScript
- Understanding the Game Logic
- Setting Up the HTML Structure
- Writing the JavaScript Code
- Enhancing the User Experience
- Conclusion
- FAQ
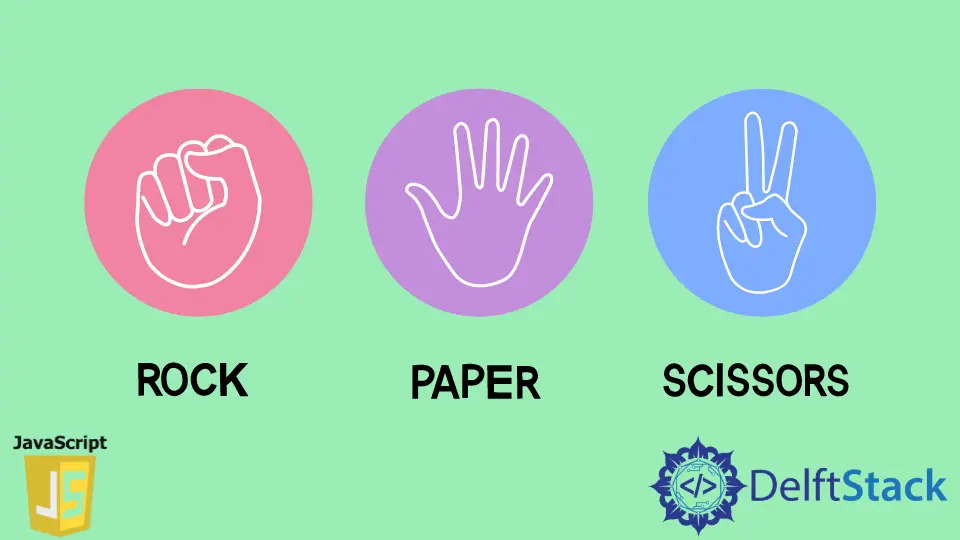
In this article, we are going to learn how to write code for the classic game Rock, Paper, Scissors using JavaScript. This simple yet engaging game is a fantastic way to practice your coding skills while having fun. Whether you’re a beginner looking to enhance your JavaScript knowledge or an experienced developer seeking a quick refresher, this tutorial will guide you through the process step-by-step. We’ll cover the essential components of the game, including user input, random choices, and game logic. By the end of this article, you’ll have a fully functional Rock, Paper, Scissors game that you can customize and expand upon. Let’s dive in!
Understanding the Game Logic
Before we jump into the code, it’s essential to understand the basic rules of Rock, Paper, Scissors. The game is played between two players who simultaneously choose one of three options: rock, paper, or scissors. The outcomes are determined as follows:
- Rock crushes scissors (rock wins)
- Scissors cuts paper (scissors win)
- Paper covers rock (paper wins)
If both players choose the same option, it’s a tie. These simple rules will guide our implementation in JavaScript, allowing us to create a program that can simulate the game effectively.
Setting Up the HTML Structure
To create our Rock, Paper, Scissors game, we first need a basic HTML structure. This will serve as the foundation for our JavaScript code. Here’s a simple layout that includes buttons for user interaction and a display area for the results.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rock Paper Scissors</title>
</head>
<body>
<h1>Rock Paper Scissors Game</h1>
<div>
<button id="rock">Rock</button>
<button id="paper">Paper</button>
<button id="scissors">Scissors</button>
</div>
<div id="result"></div>
<script src="script.js"></script>
</body>
</html>
This HTML code sets up a simple interface where users can click buttons to make their choices. The script.js
file will contain our JavaScript code, which will handle the game logic and update the results on the webpage.
Writing the JavaScript Code
Now that we have our HTML structure, it’s time to write the JavaScript code that will make our game functional.
const choices = ['rock', 'paper', 'scissors'];
document.getElementById('rock').addEventListener('click', () => playGame('rock'));
document.getElementById('paper').addEventListener('click', () => playGame('paper'));
document.getElementById('scissors').addEventListener('click', () => playGame('scissors'));
function playGame(userChoice) {
const computerChoice = choices[Math.floor(Math.random() * choices.length)];
let result = '';
if (userChoice === computerChoice) {
result = 'It\'s a tie!';
} else if (
(userChoice === 'rock' && computerChoice === 'scissors') ||
(userChoice === 'paper' && computerChoice === 'rock') ||
(userChoice === 'scissors' && computerChoice === 'paper')
) {
result = 'You win!';
} else {
result = 'You lose!';
}
document.getElementById('result').textContent = `You chose ${userChoice}. Computer chose ${computerChoice}. ${result}`;
}
In this code, we define an array of choices: rock, paper, and scissors. We then set up event listeners for each button, so when a user clicks one, it triggers the playGame
function with the user’s choice. Inside this function, we randomly select the computer’s choice and determine the outcome based on the game rules. Finally, we display the result on the webpage.
Output:
You chose rock. Computer chose scissors. You win!
This simple yet effective implementation allows players to enjoy the game while learning about event handling and random number generation in JavaScript.
Enhancing the User Experience
To make the game more engaging, we can add some enhancements. For instance, we can keep track of the score and display it to the user. This will encourage players to keep playing and improve their skills.
let userScore = 0;
let computerScore = 0;
function playGame(userChoice) {
const computerChoice = choices[Math.floor(Math.random() * choices.length)];
let result = '';
if (userChoice === computerChoice) {
result = 'It\'s a tie!';
} else if (
(userChoice === 'rock' && computerChoice === 'scissors') ||
(userChoice === 'paper' && computerChoice === 'rock') ||
(userChoice === 'scissors' && computerChoice === 'paper')
) {
result = 'You win!';
userScore++;
} else {
result = 'You lose!';
computerScore++;
}
document.getElementById('result').textContent = `You chose ${userChoice}. Computer chose ${computerChoice}. ${result}`;
document.getElementById('score').textContent = `Score - You: ${userScore}, Computer: ${computerScore}`;
}
With this modification, we introduced two variables to keep track of the user and computer scores. Each time a player wins, their score increases by one. The score is then displayed on the webpage, providing users with a sense of progress and competition.
Output:
You chose paper. Computer chose rock. You win!
Score - You: 1, Computer: 0
By enhancing the user experience with a scoring system, we make the game more interactive and enjoyable for players.
Conclusion
Creating a Rock, Paper, Scissors game in JavaScript is a fun and educational project for developers at any level. By following the steps outlined in this article, you can build a fully functional game that not only entertains but also helps you improve your coding skills. We explored the basic game logic, set up an HTML structure, and wrote JavaScript code to handle user interactions and game outcomes. With some enhancements, such as a scoring system, you can take your game to the next level. So why not give it a try? Customize your game, add new features, and enjoy the process of learning!
FAQ
-
How do I run the Rock, Paper, Scissors game?
You can run the game by opening the HTML file in a web browser. Ensure that the JavaScript file is properly linked. -
Can I customize the game?
Yes, you can customize the game by changing the styles, adding sounds, or implementing new features like a best-of-three series. -
What programming concepts can I learn from this project?
This project helps you learn about event handling, random number generation, and basic game logic in JavaScript. -
Is this game suitable for beginners?
Absolutely! This project is perfect for beginners looking to practice their JavaScript skills in a fun way. -
Can I deploy this game online?
Yes, you can deploy your game using platforms like GitHub Pages, Netlify, or any other web hosting service.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn