How to Convert RGB to HEX in JavaScript
- Understanding RGB and HEX Color Formats
- Method 1: Using JavaScript to Convert RGB to HEX
- Method 2: Using a Loop for Multiple Color Conversions
- Conclusion
- FAQ
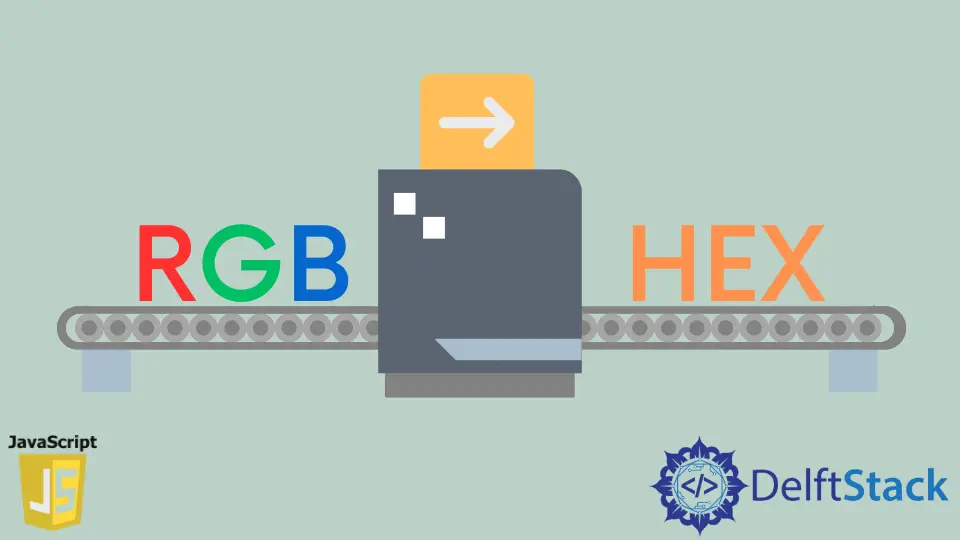
When working with colors in web development, you often encounter different color formats. One of the most common formats is RGB (Red, Green, Blue), which is widely used in CSS and JavaScript. However, sometimes you may need to convert these RGB values into HEX format, especially when dealing with design tools or libraries that prefer this representation.
In this article, we’ll explore how to convert RGB to HEX in JavaScript using the built-in toString()
function. With a few lines of code, you can effortlessly make this conversion and enhance your web projects. Let’s dive into the methods you can use!
Understanding RGB and HEX Color Formats
Before jumping into the conversion process, it’s essential to understand what RGB and HEX formats are. RGB is a color model that combines red, green, and blue light in various intensities to create a broad spectrum of colors. Each component can have a value ranging from 0 to 255, resulting in over 16 million possible color combinations.
On the other hand, HEX is a hexadecimal representation of RGB colors. It uses a six-digit code, where the first two digits represent red, the next two represent green, and the last two represent blue. For instance, the RGB value (255, 0, 0) translates to the HEX code #FF0000. Understanding these formats will help you grasp the conversion process better.
Method 1: Using JavaScript to Convert RGB to HEX
One of the simplest ways to convert RGB to HEX in JavaScript is by using the toString()
function. This method allows you to convert each color component from decimal to hexadecimal format. Below is an example code snippet demonstrating this method:
function rgbToHex(r, g, b) {
return '#' + ((1 << 24) + (r << 16) + (g << 8) + b)
.toString(16)
.slice(1)
.toUpperCase();
}
console.log(rgbToHex(255, 0, 0));
Output:
#FF0000
In this code, the rgbToHex
function takes three parameters: red, green, and blue values. It uses bitwise operations to shift the color components into their appropriate positions and combines them into a single integer. The toString(16)
method then converts this integer into a hexadecimal string. Finally, the slice(1)
method removes the leading ‘1’ added during the conversion, and toUpperCase()
ensures the HEX code is in uppercase format.
This method is efficient and straightforward, making it perfect for quick conversions. You can call the rgbToHex
function with any RGB values, and it will return the corresponding HEX code.
Method 2: Using a Loop for Multiple Color Conversions
If you need to convert multiple RGB values at once, you can enhance the previous method by using a loop. This approach allows you to handle arrays of RGB values and convert them to HEX format in a more organized manner. Here’s how you can implement it:
function rgbArrayToHex(rgbArray) {
return rgbArray.map(rgb => {
const [r, g, b] = rgb;
return '#' + ((1 << 24) + (r << 16) + (g << 8) + b)
.toString(16)
.slice(1)
.toUpperCase();
});
}
const colors = [
[255, 0, 0],
[0, 255, 0],
[0, 0, 255],
];
console.log(rgbArrayToHex(colors));
Output:
[#FF0000, #00FF00, #0000FF]
In this example, the rgbArrayToHex
function takes an array of RGB values, where each value is itself an array containing three integers (r, g, b). The map()
function iterates over each RGB array, applying the same conversion logic as in the previous method. The result is an array of HEX codes corresponding to each RGB input.
This method is particularly useful when dealing with multiple colors, making your code cleaner and more efficient. You can easily extend this to handle larger datasets or integrate it into a larger project.
Conclusion
Converting RGB to HEX in JavaScript is a straightforward process that can be accomplished using the toString()
function and some basic arithmetic. Whether you’re converting a single color or multiple colors at once, the methods discussed in this article provide efficient solutions for your web development needs. By mastering these techniques, you can enhance your ability to manipulate colors in your projects, ensuring that your designs are both beautiful and functional. So, the next time you need to convert RGB to HEX, you’ll have the tools at your disposal to do it quickly and effectively.
FAQ
- How does the RGB color model work?
The RGB color model combines red, green, and blue light in various intensities to create a wide range of colors.
-
What is the difference between RGB and HEX formats?
RGB uses decimal values for color representation, while HEX uses a six-digit hexadecimal code. -
Can I convert HEX back to RGB in JavaScript?
Yes, you can create a function to reverse the conversion from HEX to RGB by parsing the HEX string. -
Why is HEX format preferred in web design?
HEX format is concise and widely supported in CSS, making it easier to use in web development. -
Are there libraries available for color conversion in JavaScript?
Yes, libraries like Chroma.js and Color.js provide extensive color manipulation features, including conversions between RGB and HEX.