How to Change the Background Color in JavaScript
- Understanding the backgroundColor Property
- Changing Background Color on Page Load
- Changing Background Color on Button Click
- Changing Background Color with Random Colors
- Conclusion
- FAQ
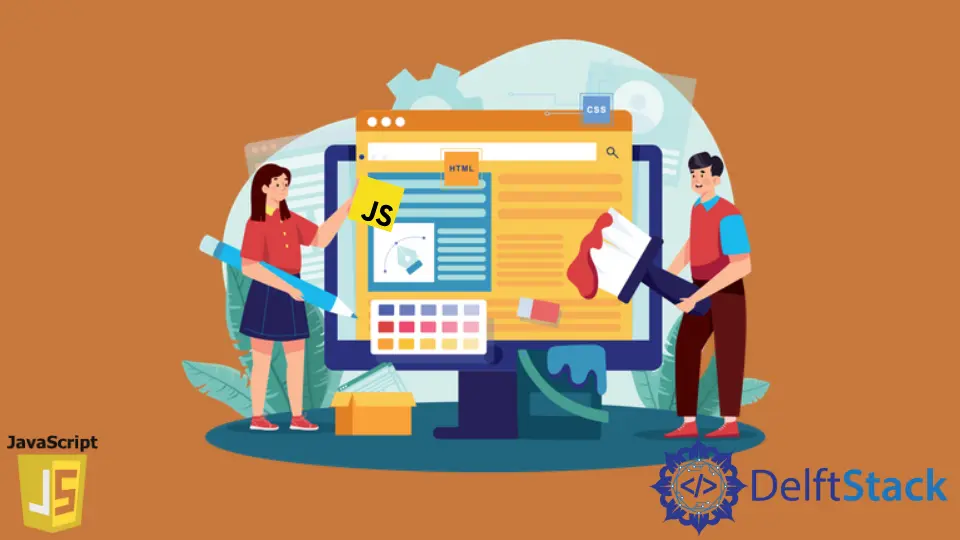
Changing the background color of a webpage can enhance user experience and improve visual appeal. In JavaScript, this is a straightforward process thanks to the backgroundColor
property. Whether you want to create a dynamic effect based on user interactions or simply set a color when the page loads, JavaScript provides the tools you need.
In this article, we will explore various methods to change the background color using JavaScript, complete with code examples and detailed explanations. By the end, you’ll have a solid understanding of how to manipulate the background color effectively.
Understanding the backgroundColor Property
The backgroundColor
property is a part of the Document Object Model (DOM) in JavaScript. It allows you to change the background color of HTML elements dynamically. This property is particularly useful when you want to create interactive web applications. You can set it to any valid CSS color value, including named colors, HEX codes, RGB, and RGBA values.
To get started, you need to select the HTML element whose background color you want to change. This can be done using methods like getElementById
, getElementsByClassName
, or querySelector
. Once you have the element, you can assign a new color to its style.backgroundColor
property.
Changing Background Color on Page Load
One of the simplest ways to change the background color is to do it when the page loads. This can be achieved by placing your JavaScript code inside a window.onload
function. Here’s an example of how to change the background color of the entire body of the webpage.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Change Background Color</title>
</head>
<body>
<script>
window.onload = function() {
document.body.style.backgroundColor = "lightblue";
};
</script>
</body>
</html>
Output:
The background color of the webpage will change to light blue when the page loads.
In this example, the window.onload
event ensures that the JavaScript code runs after the entire page has loaded. The document.body.style.backgroundColor
line modifies the background color of the body element to light blue. This method is effective for setting a default color when users first visit your website.
Changing Background Color on Button Click
Another engaging way to change the background color is through user interaction, such as clicking a button. This method makes your webpage more dynamic and interactive. Here’s how you can implement this functionality:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Change Background Color on Click</title>
</head>
<body>
<button id="colorButton">Change Background Color</button>
<script>
document.getElementById("colorButton").onclick = function() {
document.body.style.backgroundColor = "yellow";
};
</script>
</body>
</html>
Output:
The background color of the webpage will change to yellow when the button is clicked.
In this example, we create a button with the ID colorButton
. When the button is clicked, the onclick
event triggers a function that changes the background color to yellow. This approach not only enhances user interaction but also makes your website feel more responsive and lively.
Changing Background Color with Random Colors
For a more advanced and fun approach, you can change the background color to a random color each time a button is clicked. This method adds an element of surprise and can be visually stimulating for users. Here’s how to implement this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Random Background Color</title>
</head>
<body>
<button id="randomColorButton">Change to Random Color</button>
<script>
function getRandomColor() {
const letters = '0123456789ABCDEF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
document.getElementById("randomColorButton").onclick = function() {
document.body.style.backgroundColor = getRandomColor();
};
</script>
</body>
</html>
Output:
The background color of the webpage will change to a random color each time the button is clicked.
In this code, we define a function getRandomColor()
that generates a random HEX color code. When the button with the ID randomColorButton
is clicked, the onclick
event calls this function, and the background color is updated accordingly. This method not only showcases JavaScript’s capabilities but also keeps users engaged with unexpected color changes.
Conclusion
Changing the background color using JavaScript is a simple yet powerful way to enhance your website’s aesthetics and user experience. Whether you’re setting a default color on page load, responding to user actions, or generating random colors, JavaScript provides a variety of methods to achieve your goals. By mastering these techniques, you can create more interactive and visually appealing web applications. So go ahead and experiment with these methods to see how they can elevate your projects!
FAQ
-
How can I change the background color of a specific element?
You can change the background color of a specific element by selecting it usingdocument.getElementById
or other DOM selection methods and then setting itsstyle.backgroundColor
property. -
Can I use CSS color names with the backgroundColor property?
Yes, you can use CSS color names, HEX codes, RGB, and RGBA values with thebackgroundColor
property. -
Is it possible to animate the background color change?
Yes, you can use CSS transitions or animations to create smooth transitions when changing the background color. -
How do I reset the background color to its original state?
You can reset the background color by setting it back to its original value or using theinitial
keyword in thestyle.backgroundColor
property. -
Can I change the background color based on user input?
Yes, you can change the background color based on user input by capturing the input value and applying it to thestyle.backgroundColor
property.