JavaScript POST
- Understanding the JavaScript POST Method
- Using the Fetch API to Send POST Data
- Using XMLHttpRequest for POST Requests
- Conclusion
- FAQ
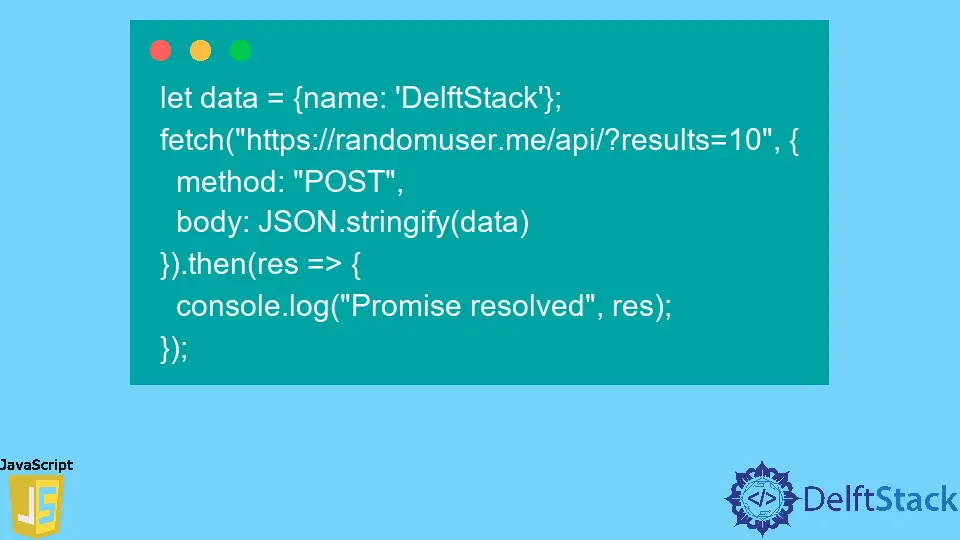
In the world of web development, JavaScript plays a crucial role in creating dynamic and interactive user experiences. One common task developers face is sending data to a server using the POST method. While many are familiar with submitting forms, there are times when you might want to send POST data without a traditional form.
This tutorial will guide you through the various ways to achieve this using JavaScript. Whether you’re looking to update a database, send user input, or communicate with an API, you’ll find practical examples and explanations to help you get started. Let’s dive into the world of JavaScript POST requests and discover how to send data seamlessly.
Understanding the JavaScript POST Method
Before we get into the nitty-gritty of sending POST requests, let’s clarify what a POST request is. In HTTP, the POST method is used to send data to the server. This data can be anything from user inputs to file uploads. Unlike GET requests, which append data to the URL, POST requests encapsulate the data within the body of the request. This makes them ideal for sending larger amounts of data securely.
In JavaScript, you can use the fetch
API or XMLHttpRequest
to send POST requests. The fetch
API is modern and promises-based, making it easier to work with asynchronous requests. Let’s explore how to use both methods to send POST data without a form.
Using the Fetch API to Send POST Data
The fetch
API simplifies the process of making network requests. Here’s how you can use it to send POST data:
const data = {
name: "John Doe",
email: "john@example.com"
};
fetch('https://example.com/api/user', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => {
console.log('Success:', data);
})
.catch((error) => {
console.error('Error:', error);
});
Output:
Success: { id: 1, name: "John Doe", email: "john@example.com" }
In this example, we create a JavaScript object called data
containing user information. The fetch
function is called with the URL where we want to send the data. We specify the HTTP method as POST
, set the Content-Type
header to application/json
, and convert our data object to a JSON string using JSON.stringify()
. The response is then processed and logged to the console. If there’s an error, it will be caught and displayed.
Using the fetch
API is a straightforward way to send POST requests, and it integrates well with modern JavaScript features like promises and async/await.
Using XMLHttpRequest for POST Requests
While the fetch
API is more modern, the XMLHttpRequest
object is still widely used and supported in all browsers. Here’s how you can achieve the same result using XMLHttpRequest
:
const data = {
name: "John Doe",
email: "john@example.com"
};
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://example.com/api/user', true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log('Success:', JSON.parse(xhr.responseText));
} else if (xhr.readyState === 4) {
console.error('Error:', xhr.statusText);
}
};
xhr.send(JSON.stringify(data));
Output:
Success: { id: 1, name: "John Doe", email: "john@example.com" }
In this example, we create an instance of XMLHttpRequest
and open a connection to the specified URL with the POST
method. We set the Content-Type
header to application/json
to indicate that we are sending JSON data. The onreadystatechange
event is used to handle the response. When the request completes (readyState 4), we check the status code. If it’s successful (status 200), we log the response. Otherwise, we log an error message.
Using XMLHttpRequest
is a bit more verbose than the fetch
API, but it remains a reliable method for sending POST requests, especially in older codebases.
Conclusion
Sending POST data without a traditional form in JavaScript is a powerful technique that enhances your web applications’ interactivity and responsiveness. Whether you opt for the modern fetch
API or the traditional XMLHttpRequest
, both methods allow you to send data efficiently. As you explore these techniques, remember to handle responses and errors gracefully to provide a seamless user experience. With these skills in your toolkit, you can build more dynamic web applications that communicate effectively with servers.
FAQ
-
What is a POST request in JavaScript?
A POST request is an HTTP method used to send data to a server. It encapsulates the data in the request body instead of appending it to the URL. -
How do I send JSON data using JavaScript?
You can send JSON data using thefetch
API orXMLHttpRequest
by setting theContent-Type
header toapplication/json
and converting your data object to a JSON string usingJSON.stringify()
. -
What is the difference between fetch and XMLHttpRequest?
Thefetch
API is more modern and promise-based, making it easier to work with asynchronous requests.XMLHttpRequest
is older, more verbose, and widely supported across all browsers. -
Can I send form data using JavaScript without a form?
Yes, you can create a JavaScript object with the data you want to send and use either thefetch
API orXMLHttpRequest
to send it as a POST request. -
How do I handle errors in a POST request?
You can handle errors in a POST request by checking the response status and using.catch()
with thefetch
API or checking thereadyState
andstatus
properties inXMLHttpRequest
.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn