JavaScript Password Generator
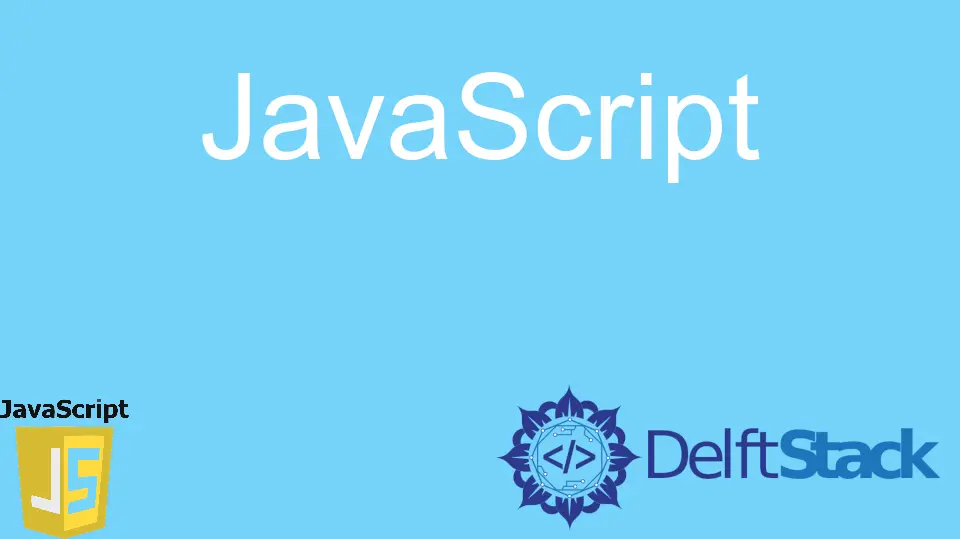
JavaScript has become very popular recently as a scripting language for web development. There are numerous things or projects we can do using JavaScript.
One of the projects that we can implement using JavaScript is a random password generator. Through this article, you will learn how to implement a random password generator using JavaScript with the help of HTML and CSS.
JavaScript Password Generator
The system that we are going to implement gives us a random password. It contains 12 characters from all the UPPERCASE and lowercase characters, numbers, and symbols.
It will display random passwords every time we click the generate button.
First, we will design a simple web page using HTML and CSS for this tutorial. Since we are creating a password generator, it needs to be more user attractive.
Then we will create a JavaScript file to activate the password generator. You can easily create a random password generator by following the below steps.
Add a Heading and a Paragraph
As the first step, we will add a heading and a small paragraph describing the web page.
Code:
<div class="headings">
<h1>Password Generator</h1>
<p>This is a password generator where you can generate random passwords</p>
</div>
Now we can add some styles to the heading (h1
) and the paragraph (p
) in the style.css
file.
Code:
body{
background-color: beige;
align-content: center;
justify-content: center;
display: block;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
.headings{
text-align: center;
}
.headings p{
margin-bottom: 40px;
font-size: 20px;
}
The output should look like this:
Add an Input Box to Display the Random Password
So far, we have added a heading and a small paragraph to our web page describing what this web page provides. Now we will add an input box where the password will be displayed.
First, add an input box using the input
tag.
<input id="showPSWD" class="inputbox" type="text" placeholder="A random password" readonly>
Here we have declared the input box ID as we use it for the getElementByID()
method in the future. Also, we have made this input box read-only since we are not modifying the content (the random password) in the input box.
Create a Button to Generate the Password
Now let us create the button to generate the password when clicked.
<button onclick="generatePSWD()">Generate</button>
Here we are invoking the generatePSWD()
function upon clicking the button. generatePSWD()
is the function we use to activate the password generating and displaying it on the input box.
Now let us style our input box and button using CSS.
.boxShape{
width: 25%;
margin: auto;
text-align: center;
}
.inputbox{
font-size: 15px;
padding: 5px;
margin-right: 5px;
}
button{
background-color: blue;
color: white;
border-radius: 6px;
border-width: 0px;
padding: 5px 20px;
margin-top: 10px;
font-size: 15px;
}
button:hover{
cursor: pointer;
}
Now the base for the password generator has been created using HTML and CSS. The complete HTML and CSS codes are shown below.
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="/style.css">
<title>Password Generator</title>
</head>
<body>
<div class="headings">
<h1>Password Generator</h1>
<p>This is a password generator where you can generate random passwords</p>
</div>
<div class="boxShape">
<input id="showPSWD" class="inputbox" type="text" placeholder="A random password" readonly>
<button onclick="generatePSWD()">Generate</button>
</div>
<script src="/activation.js"></script>
</body>
</html>
CSS Code:
body{
background-color: beige;
align-content: center;
justify-content: center;
display: block;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
.headings{
text-align: center;
}
.headings p{
margin-bottom: 40px;
font-size: 20px;
}
.boxShape{
width: 25%;
margin: auto;
text-align: center;
}
.inputbox{
font-size: 15px;
padding: 5px;
margin-right: 5px;
}
button{
background-color: blue;
color: white;
border-radius: 6px;
border-width: 0px;
padding: 5px 20px;
margin-top: 10px;
font-size: 15px;
}
button:hover{
cursor: pointer;
}
Now our web page should look like this:
Activate the Password Generator Using JavaScript
To do this, let us create a variable and assign it to the input ID: showPSWD
using the getElementByID()
method.
let pswd = document.getElementById('showPSWD');
Then we create a JavaScript function called generatePSWD()
. Inside the function, we declare three variables: randPSWD
, characters
, and lenOfPSWD
.
randPSWD
is an empty string where the randomly selected characters will store and display in the input box.
lenOfPSWD
contains 12 as the length of a random password. You can also change the length in your favor.
All the characters, numbers, and symbols will store in the characters
variable. They will be randomly selected to create the passwords.
function generatePSWD() {
let randPSWD = '';
let lenOfPSWD = 12;
let characters =
'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()';
Now we can use a for
loop to generate a random password.
for (let i = 0; i < lenOfPSWD; i++) {
let randChar = Math.floor(Math.random() * characters.length);
randPSWD += characters.substring(randChar, randChar + 1);
}
Here we have used Math.random
to select a random character from the characters string. randChar
is the randomly selected character. randChar
s in every iteration store in randPSWD
.
Finally, the randPSWD
string can be assigned as the value of the getElementByID()
method so it will display in the input box.
document.getElementById('showPSWD').value = randPSWD;
Below is the full JavaScript code:
let pswd = document.getElementById('showPSWD');
function generatePSWD() {
let randPSWD = "";
let lenOfPSWD = 12;
let characters = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()";
for (let i = 0; i < lenOfPSWD; i++) {
let randChar = Math.floor(Math.random() * characters.length);
randPSWD += characters.substring(randChar, randChar + 1);
}
document.getElementById("showPSWD").value = randPSWD;
}
A random password is displayed in the input box when we click the button. You can click the button over and over to create random passwords.
Output:
Conclusion
We just walked through a tutorial where we built a random password generator using JavaScript with HTML and CSS. There are more different methods to achieve this, but this method is another easy way of creating a random password generator.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.