How to Open Popup Window in JavaScript
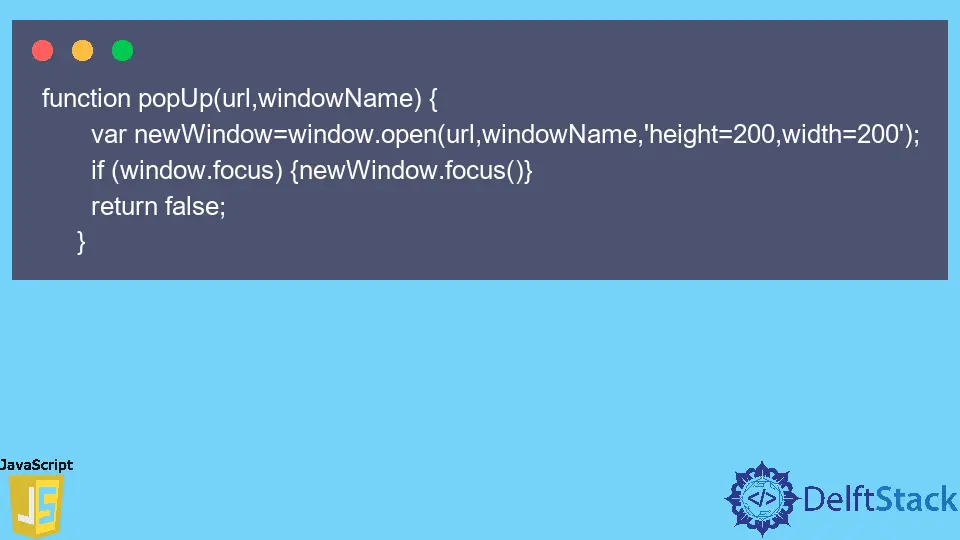
This article will introduce a method to open a popup window using JavaScript.
Open a Popup Window Using the Window open()
Method in JavaScript
A popup window is another window that shows the user the extra information while keeping the original window open. Sometimes, a popup window must be used while interacting with the user on a website.
One of the popular scenarios is while performing an OAuth authorization. For example, whenever you try to log in to a SAAS application using your Gmail account, a new popup window opens and shows the list of Gmail account to choose from to log in to the application.
The important concept to note about the popup window is that it has a JavaScript environment, making it safe to run third-party applications and untrusted sites. Now, let’s see how we can create such popup windows.
The open()
method provided by the window
object opens up a new window. The syntax of the open()
method is shown below.
window.open(url, name, parameter)
The url
option is the URL of the page that is to be opened as a popup window. The name
option is the window’s name to be opened.
window.name
defines the window’s name in the browser. The parameter
option is a notation for the configuration option while opening a window.
It contains several configurations like height/width, menu bar, toolbar, etc.
For example, create a JavaScript function popUp()
that takes two parameters, url
and windowName
. Inside the function, create a variable newWindow
and call the open()
method using the window
object in the variable.
In the open()
method, supply the parameters url
, windowName
, height
, and width
. Set the height and width of your choice.
Next, check the condition window.focus
, and if it is true
, set the focus to the new window you just created as newWindow.focus()
. Finally, return false
.
In HTML, create an anchor tag <a> </a>
. Set some URLs of your choice in the href
attribute.
Then, inside the anchor tag, create an onclick
event. Call the popUp()
function with the same URL as in the href
attribute and the window name as the parameters.
Between the anchor tags, write some text to create a clickable link.
Example Code:
function popUp(url, windowName) {
var newWindow = window.open(url, windowName, 'height=200,width=200');
if (window.focus) {
newWindow.focus()
}
return false;
}
<a href="https://www.youtube.com/" onclick="return popUp('https://www.youtube.com/', 'youtube')">Youtube Popup</a>
In the example above, we have used Youtube’s URL https://www.youtube.com/
to create a new popup window. Whenever the onClick
event occurs, a new window is created.
Then, the focus is shifted to the newly created window to display it as a popup. We have returned false
in the function so that the browser won’t be redirected to the URL set in the href
attribute.
Thus, you learned how to open a new popup window using the window.open
method in JavaScript.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn