Number Guessing Game in JavaScript
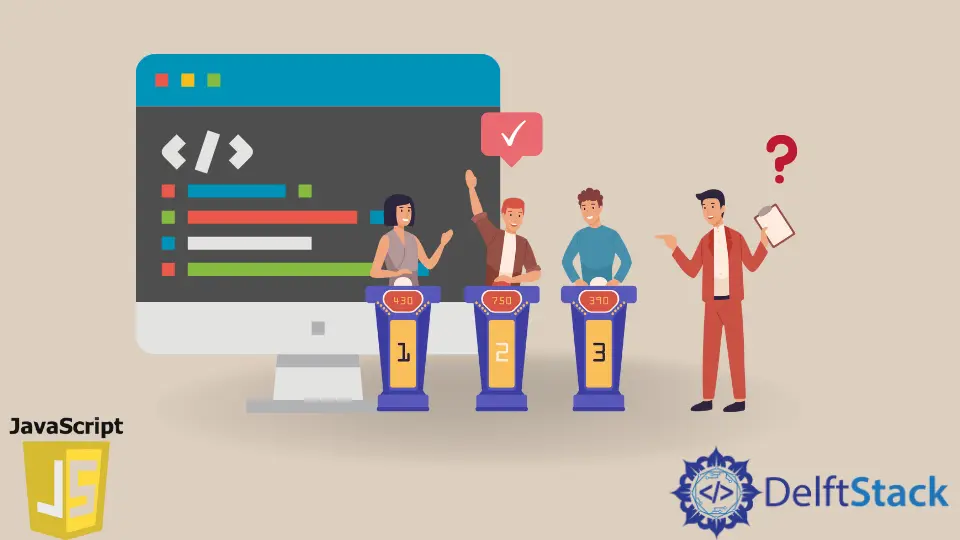
We use JavaScript to make games that we play in different browsers. The number guessing game is one of the games that we create using JavaScript.
In number guessing games, the user has to guess the correct number in the given range of numbers. The user has only limited attempts to win the game by guessing the correct number in the given range.
If he fails to guess the correct number, he loses the game.
This article will demonstrate how to create a random number guessing game in JavaScript.
Create a Random Number Guessing Game Using JavaScript
In the following example, we write a program that implements a simple guessing number game. The computer generates a random number in the game, and the user gets ten chances to guess the secret number.
On each guess, the program will inform the user whether their guess is too high, too low, or correct. When the user guesses the correct number or uses up their 10 guesses, the game will be over, and the program will no longer accept guesses.
Let’s understand the following code step by step.
As we can see in the code, first, we create an HTML page, and inside the page code, we add JavaScript. In the script, we create different variables first.
We create a randomNumber
variable in the script and assign a value 1-15. It means that the computer will generate the correct random number between 1-15.
So the user has to guess the number between 1-15, and he has only ten chances to get the random value correct.
We create a checkGuess()
function in the following code. Then, we used the if-else
condition in this function.
When a user enters the number, the function calls and checks whether the entered value is too low, too high, or correct. If the user’s entered number is less than the right random number, a message will be displayed that says the Last guess was too low
.
And if the user entered a number greater than the correct random number, then a message will be displayed that says the Last guess was too high
. This helps the user in guessing the random number.
If the user enters the number equal to the random number, then the game will be over, and a message will be displayed that says Congratulations! You got it right
.
If the user cannot guess the random number in ten chances, the game is over. A message, GAME OVER
, will be displayed.
This is how we can create a random number guessing game using a JavaScript program.
Example code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Number guessing game</title>
<style>
html {
font-family: sans-serif;
}
body {
width: 50%;
max-width: 800px;
min-width: 480px;
margin: 0 auto;
}
.lastResult {
color: white;
padding: 3px;
}
</style>
</head>
<body>
<h1>Number guessing game</h1>
<p>We selected a random number between 1 and 15. Can you guess it in the 10 chances that you got? We will let you know if your guess was too high or too low.</p>
<div class="form">
<label for="guessField">Enter your guess</label><input type="text" id="guessField" class="guessField">
<input type="submit" value="Submit guess" class="guessSubmit">
</div>
<div class="resultParas">
<p class="guesses"></p>
<p class="lastResult"></p>
<p class="lowOrHi"></p>
</div>
<script>
let randomNumber = Math.floor(Math.random() * 15) + 1;
const guesses = document.querySelector('.guesses');
const lastResult = document.querySelector('.lastResult');
const lowOrHi = document.querySelector('.lowOrHi');
const guessSubmit = document.querySelector('.guessSubmit');
const guessField = document.querySelector('.guessField');
let guessCount = 1;
let resetButton;
function checkGuess() {
const userGuess = Number(guessField.value);
if (guessCount === 1) {
guesses.textContent = 'Previous guesses: ';
}
guesses.textContent += userGuess + ' ';
if (userGuess === randomNumber) {
lastResult.textContent = 'Congratulations! You guessed it right!';
lastResult.style.backgroundColor = 'green';
lowOrHi.textContent = '';
setGameOver();
} else if (guessCount === 10) {
lastResult.textContent = '!!!GAME OVER!!!';
lowOrHi.textContent = '';
setGameOver();
} else {
lastResult.textContent = 'Wrong!';
lastResult.style.backgroundColor = 'red';
if(userGuess < randomNumber) {
lowOrHi.textContent = 'Last guess was too low!' ;
} else if(userGuess > randomNumber) {
lowOrHi.textContent = 'Last guess was too high!';
}
}
guessCount++;
guessField.value = '';
guessField.focus();
}
guessSubmit.addEventListener('click', checkGuess);
function setGameOver() {
guessField.disabled = true;
guessSubmit.disabled = true;
resetButton = document.createElement('button');
resetButton.textContent = 'Start new game';
document.body.appendChild(resetButton);
resetButton.addEventListener('click', resetGame);
}
function resetGame() {
guessCount = 1;
const resetParas = document.querySelectorAll('.resultParas p');
for (const resetPara of resetParas) {
resetPara.textContent = '';
}
resetButton.parentNode.removeChild(resetButton);
guessField.disabled = false;
guessSubmit.disabled = false;
guessField.value = '';
guessField.focus();
lastResult.style.backgroundColor = 'white';
randomNumber = Math.floor(Math.random() * 15) + 1;
}
</script>
</body>
</html>
Output:
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn