How to Make a Div Clickable Using JavaScript
-
Using the
onclick
Event Handler to Make a Div Clickable - Adding a Conditional Click to a Div
- Good to Have Feature in a Clickable Div
- Achieving Anchor Element Functionality With a Clickable Div
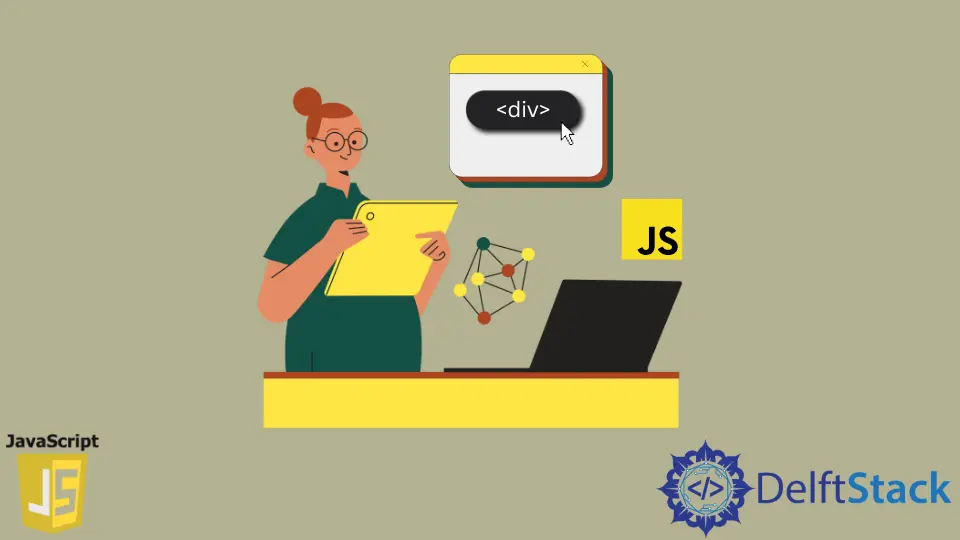
The <a>
tag (or the anchor element) in HTML is used commonly for navigation controls. It is a clickable tag that functions without any javascript code working behind the scenes. It is not a block element. Can we use a <div>
tag for routing to a path just like the anchor tag? The answer is definitely yes, but you will need to write javascript code for it. We can use a click handler
on the div
element to make it clickable.
Using the onclick
Event Handler to Make a Div Clickable
The anchor element is a displayed inline. And this feature may not always work in our favour while designing the Graphical User Interface. div
s are easy to use while designing GUIs as they are stand-alone block elements. A div
is a content division element and is not clickable by default. We may have to use the onclick
event handler to process the events emitted by the element when a user clicks on it. The onclick
event handler accepts a function or a JavaScript expression preceding the function. When a user clicks on the div
, javascript executes the associated functions or the expressions at the run time. There are a couple of ways to add the onclick
event handler to a div
element.
Using Both HTML and JavaScript Code Combined to Make a Div Clickable
The most widely used approach in javascript and various frameworks of javascript is to link a function call to the onclick
event handler in the HTML code. Then we write the function definition associated with it in the javascript code. Once a user clicks the div
, the onclick
event handler will execute the function passed as an argument to the onclick
of the div
element. Refer to the following code.
<div id="title-div" onclick="sayHello()"></div>
function sayHello() {
console.log('Hello there !!');
}
Once the user clicks the title-div
, the associate function sayHello()
gets executed. And we will have Hello there !!
logged in the web console. This approach is widely used and makes it easy to debug as compared to the pure javascript approach. The HTML explicitly shows the binding.
Using Pure JavaScript to Make a div
Clickable
Another approach is purely javascript based. We first find the intended HTML element using any of the methods like document.getElementById()
, document.getElementsByClassName()
, document.querySelectorAll()
etc. The most commonly used method to find the HTML element is the document.getElementById()
as it uniquely identifies the HTML elemnt based on the id
we assign to it in the HTML. Once we find the element, the div
element, we attach the onclick
event handler and link the function to be executed on click
of the div
. The following code elleborates the approach.
<div id="title-div">Click here</div>
window.onload =
function() {
var el = document.getElementById('title-div');
el.onclick = sayHello;
}
function sayHello() {
console.log('Hello');
}
In the above code, we bind the onclick
event handler purely in JavaScript. There is no trace of any onclick
mapping in the HTML. The HTML is kept simple with a div
element and an associated id
. Also, note that we assign the sayHello
function object to the onclick
event handler. If we link sayHello()
(with parenthesis) instead of sayHello
, it will cause the JavaScript to execute the function on the window
load itself. And nothing will happen when a user clicks on the div
element.
Adding a Conditional Click to a Div
We can trigger the click event conditionally. Using HTML and JavaScript approach, we can add expression coupled with the &&
or the ||
sign based on the requirement. JavaScript executes the click
event based on how the boolean expression evaluates. If it evaluates to true, JavaScript executes the associated function.
<div onclick="isEvenClick() && sayHello()">Click Here !!!</div>
function sayHello() {
console.log('Hello');
}
function isEvenClick() {
clickCounter++;
return (clickCounter % 2 === 0)
}
The above code will log Hello
only on an even number of clicks. We can also write the same code with expression instead of the isEvenClick()
function as elaborated in the following code.
<div onclick="(++clickCounter % 2 === 0) && sayHello()" id="title-div">Click here</div>
The expression is evaluated at run time for the value of clickCounter
. If the clickCounter
is even, the expression (++clickCounter % 2 === 0)
evaluates to true
and the sayHello()
function gets executed.
Good to Have Feature in a Clickable Div
Once we add a click
listener to a div
, it will still be a div
. The user will not understand whether that HTML element has any user interaction associated with it. For that purpose, we can add features using the CSS styles. One such thing is to make the cursor a hand icon. We can do it by using cursor: pointer
. It is a good UI/UX practice to hint to the end-user that this HTML element is actionable. We can do it in the following way.
<div onclick="sayHello()" style="cursor: pointer;">Click Here !!!</div>
We can achieve the same using pure JavaScript by adding styles to the element at run time. We first select the element using document.getElementById()
and then add the cursor style using el.style.cursor
and assign it the string value pointer
. The following code explains it.
<div onclick="sayHello()">Click Here !!!</div>
window.onload =
function() {
var el = document.getElementById('title-div');
el.style.cursor = 'pointer';
el.onclick = sayHello;
}
function sayHello() {
console.log('Hello');
}
Achieving Anchor Element Functionality With a Clickable Div
We can use a div
element to mimic an anchor
element. The <a>
tag has an attribute called href
that creates a hyperlink. We can mimic the same behavior using the onclick
of a div. We link a function to capture the click
event in the HTML code. In the javascript code, we use the window.location.href
attribute to link a URL to the div
. Observe the functioning in the following code.
<div onclick="navToGoogle()" style="cursor: pointer;">Take me to Google</div>
function navToGoogle() {
window.location.href = 'https://www.google.com';
}