How to Create Unique ID With JavaScript
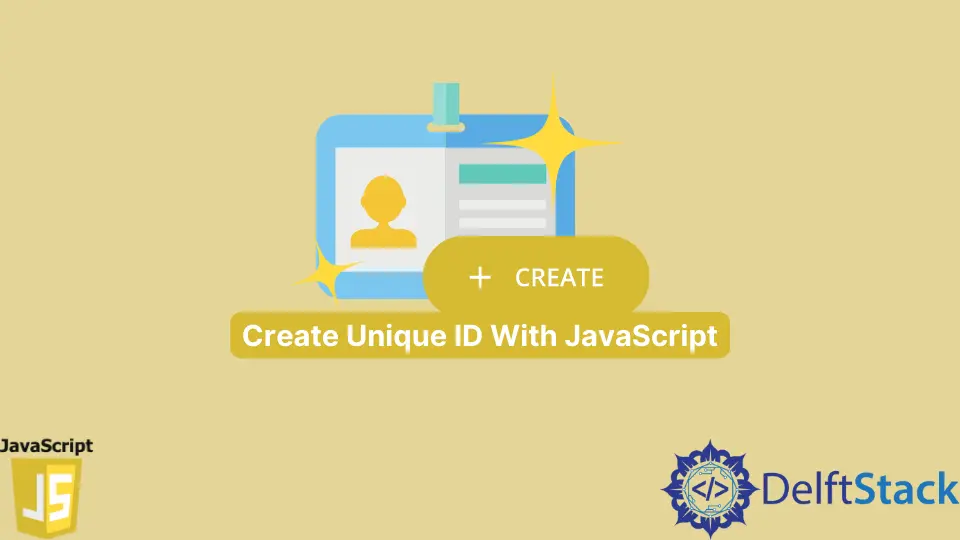
A unique identifier (UID) is an identifier whose uniqueness is guaranteed among all the identifiers used for these objects and a specific purpose. The concept was formalized early in information technology and information systems development.
Unique identification is important in all the areas related to technology (such as in relational databases) to identify the unique records; certain attributes of an entity that serve as unique identifiers are called primary keys.
Today’s post will teach us how to create a unique id with JavaScript.
Use Math.random
to Create a Unique ID in JavaScript
The Math.random()
is built-in function provided by JavaScript. This function returns a pseudo-random floating-point number between 0 (including 0) and less than 1 (excluding 1) with roughly equal distribution over that range, which you can then set to a random scale to the desired range.
The implementation selects the starting seed for the random number generation algorithm. It is completely random and cannot be chosen or reset by the user.
Syntax:
Math.random()
This method does not take any input from the users. This is one of the popular functions used to generate 4/6 digit OTP.
You can find more information about Math.random
in the documentation for the method Math.random
.
console.log(Math.ceil(Math.random() * 1000000000))
The code above uses the Math.ceil
function to round the number since the random
function returns the floating-point value. You can multiply the output with 10*n
to generate an n
digit number.
The above code’s output will vary each time you run the code.
Output:
626963298
Use getTime
to Create a Unique ID in JavaScript
The getTime()
is built-in method provided by JavaScript. This method returns the number of milliseconds since the ECMAScript epoch.
You can use this method to assign a date and time to another Date
object. It is equivalent to the valueOf()
method.
Syntax:
getTime()
This method does not take any input from the user. This method returns a number representing the milliseconds that elapsed between January 1, 1970 00:00:00 UTC
and the specified date.
You can find more information about getTime
in the documentation for the method getTime
.
Example:
console.log(new Date().getTime())
console.log(new Date().getTime() * Math.random() * 100000)
In the above code, we print the milliseconds elapsed between 1 January 1970 00:00:00 UTC
and the current date. We can use the Math.random
function to generate the unique id.
The above code’s output will vary each time you run the code.
Output:
1647189474700
9404572545500480
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn