How to Try Without Catch in JavaScript
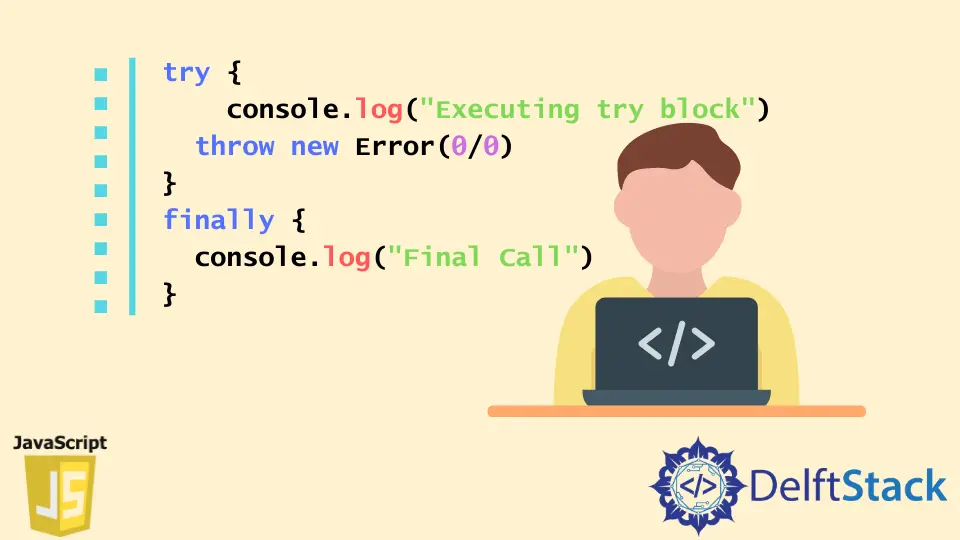
Today’s post will teach about try
statements without implementing catch
in JavaScript.
Try
Without Catch
in JavaScript
The JavaScript try
block is used to enclose code that may throw an exception. It must be used within the method.
If an exception occurs in the specific statement, it should be caught in the catch
statement.
Syntax:
try {
try_statements
/* Code to executed */
} catch (exception_var) {
catch_statements
/* Handle exception */
} finally {
finally_statements
/* Final Code/Clean variables */
}
- The
try_statements
are the statements to be executed. - The
catch_statements
are the statements to be executed when an exception is thrown in thetry
block. - An
exception_var
is an optional identifier containing an exception object for the associatedcatch
block. - The
finally_statements
are the statements executed after the test statement completes. These statements are executed regardless of whether an exception is thrown or caught.
The try
statement consists of a try
block containing one or more statements. {}
should always be used, even for single statements.
A catch
block or finally
block must be present. This gives us three combinations for the try
statement.
try...catch
try...finally
try...catch...finally
A catch
block includes statements that designate what to do simultaneously as an exception is thrown within the try
block. If a statement within the try
block (or in a function called from the try
block) throws an exception, control immediately switches to the catch
block.
The catch
block is ignored/skipped if no exception is thrown in the try
block.
The finally
block is always executed after the try
and catch
blocks have finished execution. Whether or not an exception was thrown or stuck, the finally
block is usually executed with the statements inner it.
One or more test statements can be nested. If an inner try
statement does not have a catch
block to handle the error, the catch
block of the enclosing try
statement is used instead.
The try
statement can also be used to handle JavaScript exceptions. If an exception is thrown, the statements in the finally
block will eventually execute, even if no catch
block handles the exception.
You can get more information about the try...catch
in the documentation for try...catch
.
Let’s understand it with the following example.
try {
console.log('Executing try block')
throw new Error(0 / 0)
} finally {
console.log('Final Call')
}
In the example above, we have defined a try
without catch
. We throw the error by dividing 0
with 0
.
It will throw a NaN
error which the finally
block will not catch because of the missing catch
block, and print the "Final Call"
statement inside the finally
block. You can also use these blocks to perform the required actions and clean up unused variables/resources in the finally
block.
Attempt to run the above code snippet in any browser that supports JavaScript; it will display the result below.
Executing try block
Final Call
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn