JavaScript Tail Recursion
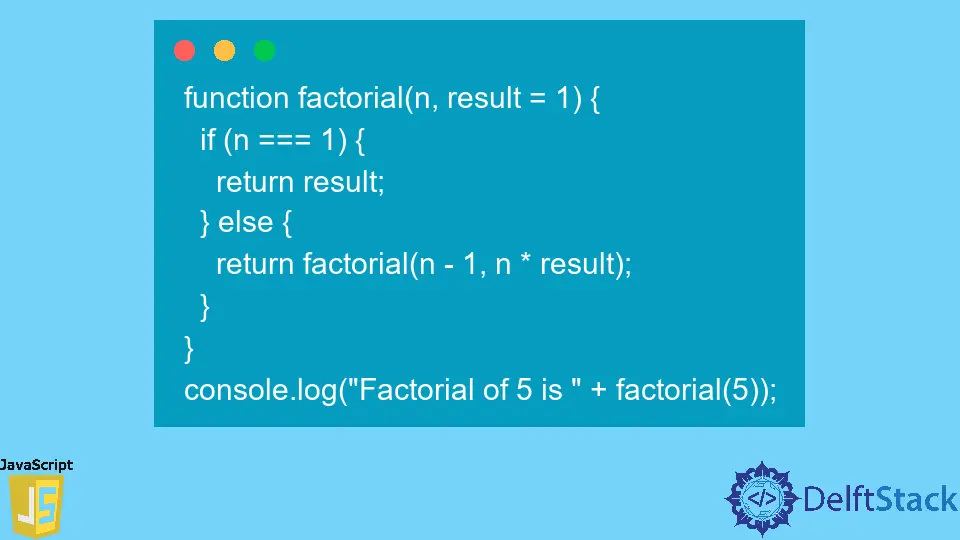
Tail recursion is a programming concept involving a function calling itself the last action it performs. This can be a useful technique for optimizing certain algorithms, as it allows the function to reuse its stack frame rather than create a new one each time it is called.
This can help reduce the amount of memory the program uses and improve its performance.
In JavaScript, tail recursion is not natively supported, but there are a few ways to achieve it using various techniques. Let’s take a closer look at what tail recursion is, how it works, and how to implement it in JavaScript.
Use of Tail Recursion
There are a few reasons why tail recursion can be useful in certain situations:
-
Memory efficiency: When a function calls itself recursively, it creates a new stack frame for each call. This can use a lot of memory, especially for deeply nested recursive calls.
Tail recursion allows the function to reuse its stack frame, reducing the amount of memory used by the program.
-
Performance: Tail recursion can also be more efficient in terms of performance, as it allows the function to avoid the overhead of creating and destroying multiple stack frames. This can make the function run faster, especially for large input values.
-
Code simplicity: Tail recursion can also make the code easier to understand, as it eliminates the need for an explicit loop or iterative structure. This can make the code more concise and easier to read.
How to Implement Tail Recursion in JavaScript
As mentioned earlier, JavaScript does not natively support tail recursion. However, there are a few ways to achieve it using various techniques.
Example 1
One approach is to use the ES6 syntax for function arguments, which allows you to specify default values for function parameters. This can be used to implement a simple wrapper function that handles the tail recursion for you.
function factorial(n, result = 1) {
if (n === 1) {
return result;
} else {
return factorial(n - 1, n * result);
}
}
console.log('Factorial of 5 is ' + factorial(5));
Output:
Factorial of 5 is 120
In this example, the result
parameter is given a default value of 1
, so if it is not specified when the function is called, it will default to 1
. This allows us to create a simple wrapper function that calls factorial
with the result
parameter set to 1
, which handles the tail recursion for us.
Example 2
Another approach is to use the arguments.callee
property, which refers to the currently executing function. This can be used to implement a recursive function that calls itself as the last action it performs, like below.
function factorial(n) {
if (n === 1) {
return 1;
} else {
return n * arguments.callee(n - 1);
}
}
console.log('Factorial of 5 is ' + factorial(5));
Output:
Factorial of 5 is 120
This approach works, but it has a few drawbacks. First, the arguments.callee
property is deprecated in strict mode, so using it can cause issues if you use strict mode in your code.
Second, the arguments.callee
property can be overwritten by other code, which can cause unexpected behavior.
Example 3
A third approach is to use a trampoline
function, a higher-order function that wraps a recursive function and allows it to be called in a tail-recursive manner. To implement a trampoline function, you can use the following pattern:
function trampoline(f) {
while (f && f instanceof Function) {
f = f();
}
return f;
}
function factorial(n, result = 1) {
if (n === 1) {
return result;
} else {
return () => factorial(n - 1, n * result);
}
}
console.log('Factorial of 5 is ' + trampoline(factorial(5)));
Output:
Factorial of 5 is 120
In this example, the factorial
function is written in a tail-recursive manner, with the recursive call being the last action performed before the function returns. The trampoline
function then wraps the factorial
function and handles the recursive calls for us, allowing us to call factorial
in a tail-recursive manner.
Using a trampoline
function can be a little more complex than the other approaches, but it has the advantage of being more efficient and less prone to errors.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn