How to Build A Stopwatch in JavaScript
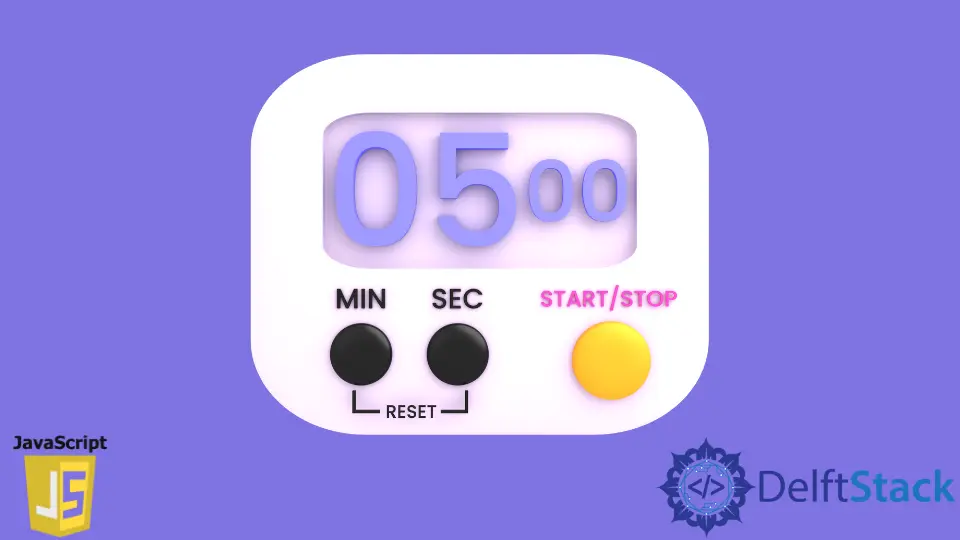
A stopwatch is used to measure the amount of time between its activation and deactivation. It helps to measure the time taken for a specific activity. We will build our stopwatch using the JavaScript timing methods setTimeout()
and clearTimeout()
that helps in time-interval-related implementations. The stopwatch has a display and three buttons. The display shows the time and the three buttons to start, stop and reset the stopwatch.
This tutorial teaches how to build a stopwatch in JavaScript.
JavaScript Stopwatch
HTML Code
<h1><time>00:00:00</time></h1>
<button id="strt">start</button>
<button id="stp">stop</button>
<button id="rst">reset</button>
In the above code, we use HTML to display the time of the stopwatch and create the three required buttons start
, stop
and reset
.
JavaScript Code
var h1 = document.getElementsByTagName('h1')[0];
var start = document.getElementById('strt');
var stop = document.getElementById('stp');
var reset = document.getElementById('rst');
var sec = 0;
var min = 0;
var hrs = 0;
var t;
function tick() {
sec++;
if (sec >= 60) {
sec = 0;
min++;
if (min >= 60) {
min = 0;
hrs++;
}
}
}
function add() {
tick();
h1.textContent = (hrs > 9 ? hrs : '0' + hrs) + ':' +
(min > 9 ? min : '0' + min) + ':' + (sec > 9 ? sec : '0' + sec);
timer();
}
function timer() {
t = setTimeout(add, 1000);
}
timer();
start.onclick = timer;
stop.onclick = function() {
clearTimeout(t);
} reset.onclick = function() {
h1.textContent = '00:00:00';
seconds = 0;
minutes = 0;
hours = 0;
}
-
We first select all the HTML elements by using selectors so that we can manipulate them using JavaScript and initialize
3
variableshrs
,min
, andsec
to store the current time. We also declare a variablet
to storesetTimeout()
and clear it by callingclearTimeout()
. -
We have attached the timer function to the
start
button. Whenever we click thestart
button, thetimer()
function is called, which in turn calls the add function at an interval of1
second. Theadd()
function calls thetick()
function to increment seconds by1
and adjust minutes and hours accordingly. It resets the content of the display to display the new time and then recalls itself by again calling thetimer()
function. -
We have attached the
clearTimeout()
to thestop
button to stop calling the add function periodically. -
We have attached a function resetting the time as
00:00:00
and reset thesec
,min
, andhrs
to0
toreset
button.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn