How to Implement Singleton in JavaScript
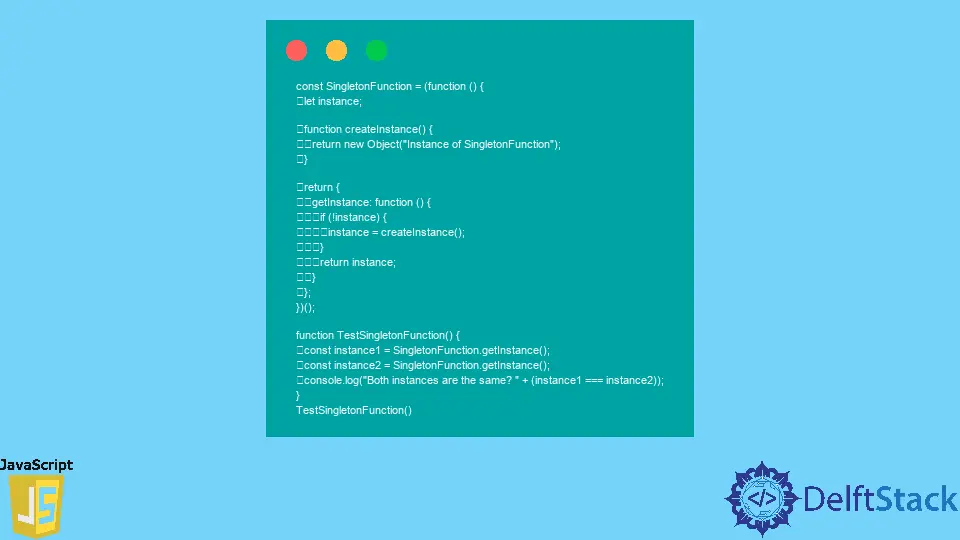
In today’s post, we’ll learn how to implement singleton classes in JavaScript using the simplest way.
Singleton Implementation in JavaScript
In programming languages like Java, one object can have multiple instances. But when we want to restrict only a single instance of an object, a singleton pattern comes into the picture.
This pattern limits the number of instances of a particular object to just one at any given point in time. The name of this single instance is a singleton.
This concept is useful when coordinating system-level actions from a central location. One of the best examples is a database connection pool.
The database connection is important for any application, and the pool manages the creation, destruction, and duration of all database connections for the entire application, ensuring that no connection is lost.
Let’s take an example.
const SingletonFunction = (function() {
let instance;
function createInstance() {
return new Object('Instance of SingletonFunction');
}
return {
getInstance: function() {
if (!instance) {
instance = createInstance();
}
return instance;
}
};
})();
function TestSingletonFunction() {
const instance1 = SingletonFunction.getInstance();
const instance2 = SingletonFunction.getInstance();
console.log('Both instances are the same? ' + (instance1 === instance2));
}
TestSingletonFunction()
In the above example, the getInstance
method acts as the Singleton gatekeeper. It returns the only instance of the object while maintaining a private reference to it that is not accessible to the outside world.
The getInstance
method illustrates another design pattern called Lazy Load
. Lazy Load checks to see if an instance has already been created; otherwise, it creates one and stores it for future reference.
All subsequent calls will receive the archived instance. Lazy loading is a memory and CPU-saving technique that creates objects only when necessary.
When you create two instances of the singleton method, both instances are the same in terms of datatype and value. When you run the above code in any browser, you will see that both instances are the same instance.
Output:
"Both instances are the same? true"
Advantages of Singleton in JavaScript
Global variables are an important part of JavaScript, but singletons reduce the need for global variables. Several other models, such as Factory
, Prototype
, and Façade
, are often implemented as Singleton when only one instance is needed.
This object is implemented as an immediate, anonymous function. The function is executed immediately by enclosing it in parentheses, followed by two more parentheses.
Because it has no name, it is called anonymous.
Singleton is a manifestation of a common JavaScript pattern: the Module.Module
pattern is the basis for all major JavaScript libraries and frameworks (jQuery, Backbone, Ember, etc.).
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn