How to Set Textarea Value in JavaScript
-
Method 1: Using the
value
Property - Method 2: Using jQuery
- Method 3: Using Event Listeners
- Conclusion
- FAQ
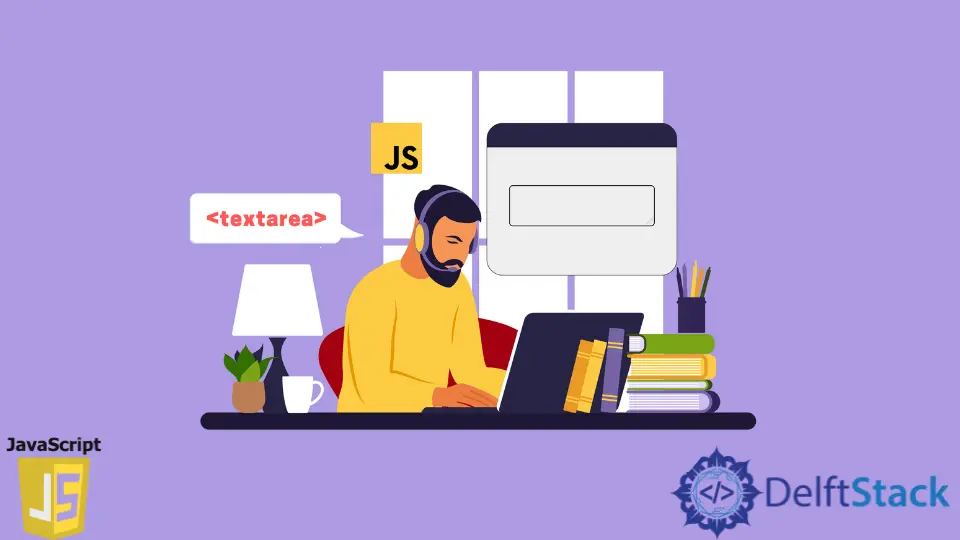
In today’s post, we’ll learn how to set textarea value in JavaScript. Textareas are essential elements in web forms, allowing users to input larger amounts of text. Understanding how to manipulate these elements using JavaScript can significantly enhance user interaction and experience. Whether you want to pre-fill a textarea with default text or dynamically update it based on user actions, knowing how to set the value is crucial.
In this article, we will explore various methods to set the value of a textarea in JavaScript, providing clear examples and explanations for each approach. Let’s dive in!
Method 1: Using the value
Property
One of the simplest ways to set the value of a textarea in JavaScript is by using the value
property. This method allows you to directly assign a string to the textarea element, making it straightforward to update its content dynamically.
Here’s an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Set Textarea Value</title>
</head>
<body>
<textarea id="myTextarea"></textarea>
<button onclick="setTextareaValue()">Set Value</button>
<script>
function setTextareaValue() {
const textarea = document.getElementById('myTextarea');
textarea.value = 'This is the new value for the textarea!';
}
</script>
</body>
</html>
Output:
This is the new value for the textarea!
In this example, we create a simple HTML structure with a textarea and a button. When the button is clicked, the setTextareaValue
function is triggered. Inside this function, we use document.getElementById
to access the textarea element by its ID. We then set its value
property to a new string. This approach is effective for both static and dynamic content, making it a go-to method for many developers.
Method 2: Using jQuery
For those who prefer using jQuery, setting the value of a textarea can be even more convenient. jQuery simplifies DOM manipulation and allows for cleaner syntax. If you are already using jQuery in your project, this method can be very effective.
Here’s how you can do it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Set Textarea Value with jQuery</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<textarea id="myTextarea"></textarea>
<button id="setValueBtn">Set Value</button>
<script>
$('#setValueBtn').click(function() {
$('#myTextarea').val('This is a new value set using jQuery!');
});
</script>
</body>
</html>
Output:
This is a new value set using jQuery!
In this example, we include the jQuery library and use the click
method to handle the button click event. When the button is pressed, the val()
method sets the textarea’s value. This method is particularly useful when dealing with complex applications where jQuery is already in use, as it provides a more concise way to manipulate elements.
Method 3: Using Event Listeners
Another effective method to set a textarea value is by utilizing event listeners. This approach allows you to set the value based on specific user interactions, providing a more interactive experience.
Here’s an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Set Textarea Value with Event Listener</title>
</head>
<body>
<textarea id="myTextarea"></textarea>
<input type="text" id="inputValue" placeholder="Type your value here"/>
<button id="setValueBtn">Set Value</button>
<script>
document.getElementById('setValueBtn').addEventListener('click', function() {
const textarea = document.getElementById('myTextarea');
const inputValue = document.getElementById('inputValue').value;
textarea.value = inputValue;
});
</script>
</body>
</html>
Output:
[User-defined input value]
In this example, we set up an input field where users can type in their desired value. When the button is clicked, an event listener captures the click event and retrieves the value from the input field. This value is then assigned to the textarea. This method is particularly beneficial when you want to allow users to specify their input dynamically, enhancing the overall functionality of your web application.
Conclusion
Setting the value of a textarea in JavaScript is a fundamental skill for web developers. Whether you choose to use the value
property, jQuery, or event listeners, each method has its advantages and can be applied based on your project needs. The flexibility of JavaScript allows you to create interactive and user-friendly forms that enhance the user experience. By mastering these techniques, you can take your web development skills to the next level and create more dynamic applications.
FAQ
- How can I set a default value for a textarea?
You can set a default value by adding thevalue
attribute directly in the HTML or by using JavaScript to set thevalue
property when the page loads.
-
Can I set the textarea value based on a user selection?
Yes, you can use event listeners to capture user selections and update the textarea value accordingly. -
Is it possible to clear the textarea value using JavaScript?
Absolutely! You can set thevalue
property to an empty string to clear the textarea. -
What is the difference between using
value
andval()
in jQuery?
Thevalue
property is a standard JavaScript method, whileval()
is a jQuery method specifically designed for retrieving or setting values of form elements. -
Can I use both JavaScript and jQuery in the same project?
Yes, you can use both JavaScript and jQuery together. Just ensure that jQuery is loaded before your custom scripts.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn