How to Send Emails in JavaScript
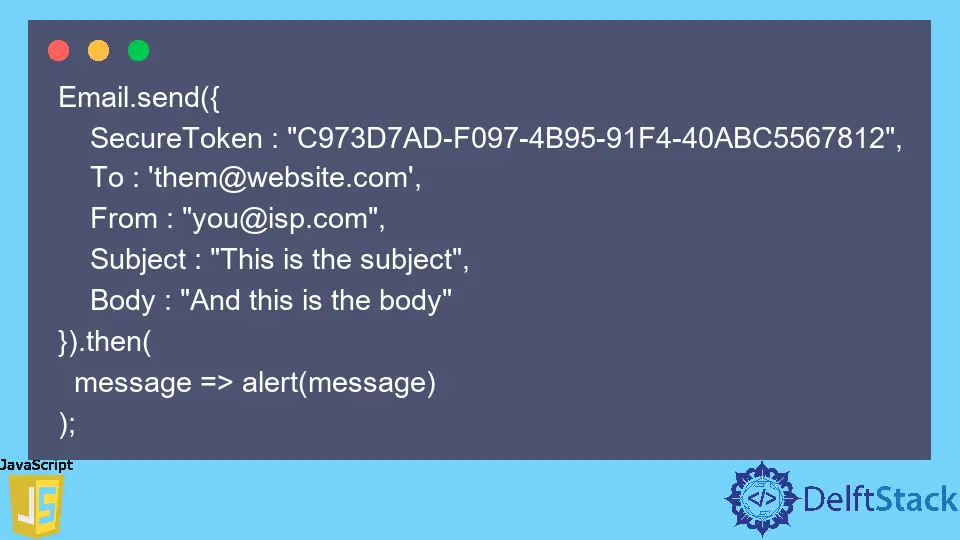
The standard method of sending emails using a web application requires that the server-side configuration of a specific web app is set up to send emails to the SMTP server. SMTP, also known as Simple Mail Transfer Protocol, can be used to transfer emails from the internet browser’s Client side makes a request to send an email to the server side, and the backend creates an email and then sends it to the SMTP server.
There is an approach to sending emails from the client side without the help of the server side, using JavaScript.
Send Emails With JavaScript
It is a common fact that only using JavaScript code cannot send direct emails because of less support for server sockets. But for the process of Email sending through the browser, we can use SMTPJS; a third-party free library offered in JavaScript.
This library offers the Email.send()
method to send an email from JavaScript. Usually, contact forums do send emails through the browser.
As an instance, we can create a contact form using the following HTML and CSS code. After that, we can add JavaScript code to send emails.
Code Example:
<!DOCTYPE html>
<html>
<header>
<meta charset="UTF-8">
<meta name="viewport" content="width=divce-width, initial-scale=1">
</header>
<body>
<style>
form{
display: flex;
flex-direction: column;
width: 50%;
margin: auto;
}
#Name,#email,#message,#btn{
padding: 20px;
margin-bottom: 20px;
border: none;
outline: none;
background-color: #ddd;
}
#btn{
width: 25%;
}
h1{
text-align: center;
}
</style>
<h1>Contact Us</h1>
<form method="post">
<input type="text" id="Name" placeholder="Your Name"><br>
<input type="email" id="email" placeholder="Your email"><br>
<input type="text" id="message" placeholder="Your Message"><br>
<input type="button" value="Send Email"
onclick="sendEmail()" />
</form>
</body>
</html>
We can create a form to help with the above code. Next, we should include the following JavaScript code in our HTML file.
<script src="https://smtpjs.com/v3/smtp.js"></script>
Including the above code, we just added SMTPJS libraries to our code that are available on smtpJS.com
. The further procedure of sending Email through the browser must have its server set up and running.
In the next step, we should create an SMTP server to send the emails to the receiver. We can create an SMTP server with Elastic Email, which provides free server service.
smtpJS.com
will direct to the Elastic Email web application. We can create an account and an SMTP server by setting up details in “create SMTP credentials”.
Once creating credentials, there will be a popup window including credentials of the new server. It’s better to copy the password and save it to use later.
Set Up Server Credentials to JavaScript Code
After creating the server go back to smtpJS.com
. It is available via the Email.send()
function as follows.
Email
.send({
Host: 'smtp.elasticemail.com',
Username: 'username',
Password: 'password',
To: 'them@website.com',
From: 'you@isp.com',
Subject: 'This is the subject',
Body: 'And this is the body'
})
.then(message => alert(message));
This piece of code should include our HTML code with replace of relevant details. The username
is an email we created, an Elastic Email account, and a password.
The To
is the receiver’s email address, and the From
should replace the sender’s email address. Also, the send button should trigger the JavaScript function as follows.
<button type="button" value="send email" onclick="sendEmail()">Send</button>
We can build the complete code in the following code, including the Email.send()
method.
This code will not work until it includes credentials given by Elastic Email when creating an SMTP server. Include a username and password to execute the code.
<!DOCTYPE html>
<html>
<head>
<title>Send Mail</title>
<script src=
"https://smtpjs.com/v3/smtp.js">
</script>
<script type="text/javascript">
function sendEmail() {
Email.send({
Host: "smtp.elasticemail.com",
Username: "sender@email_address.com",
Password: "Enter your password",
To: 'receiver@email_address.com',
From: "sender@email_address.com",
Subject: "Sending Email using javascript",
Body: "",
})
.then(function (message) {
alert("mail sent successfully")
});
}
</script>
</head>
<body>
<style>
form{
display: flex;
flex-direction: column;
width: 50%;
margin: auto;
}
#Name,#email,#message,#btn{
padding: 20px;
margin-bottom: 20px;
border: none;
outline: none;
background-color: #ddd;
}
#btn{
width: 25%;
}
h1{
text-align: center;
}
</style>
<h1>Contact Us</h1>
<form method="post">
<input type="text" id="Name" placeholder="Your Name"><br>
<input type="email" id="email" placeholder="Your email"><br>
<input type="text" id="message" placeholder="Your Message"><br>
<input type="button" value="Send Email"
onclick="sendEmail()" />
</form>
</body>
</html>
We can set To
with an array of email addresses if we have multiple receivers to receive emails.
Output:
The important thing is we can encrypt our SMTP credentials preventing visible user names and passwords from the client-side server. smtpJS.com
offer a feature called “Encrypt your SMTP credentials”.
With this feature, we can encrypt our server credentials, filling required fields. Afterward, we can include the below JS code in the HTML code to configure credentials.
Email
.send({
SecureToken: 'C973D7AD-F097-4B95-91F4-40ABC5567812',
To: 'them@website.com',
From: 'you@isp.com',
Subject: 'This is the subject',
Body: 'And this is the body'
})
.then(message => alert(message));
We can add a token created for our credentials in place of the Secure token of our HTML code. We can include it in our code to encrypt our credentials.
Using this method, we can send emails using JavaScript.
Conclusion
Sending emails from the front end of the websites is very useful for building user-interactive web applications. JavaScript offers a method by which we can send emails from frontend help with SMTPJs libraries.
We can send emails without refreshing the page and also without server-side coding.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.