How to Resize Image Using HTML Canvas in JavaScript
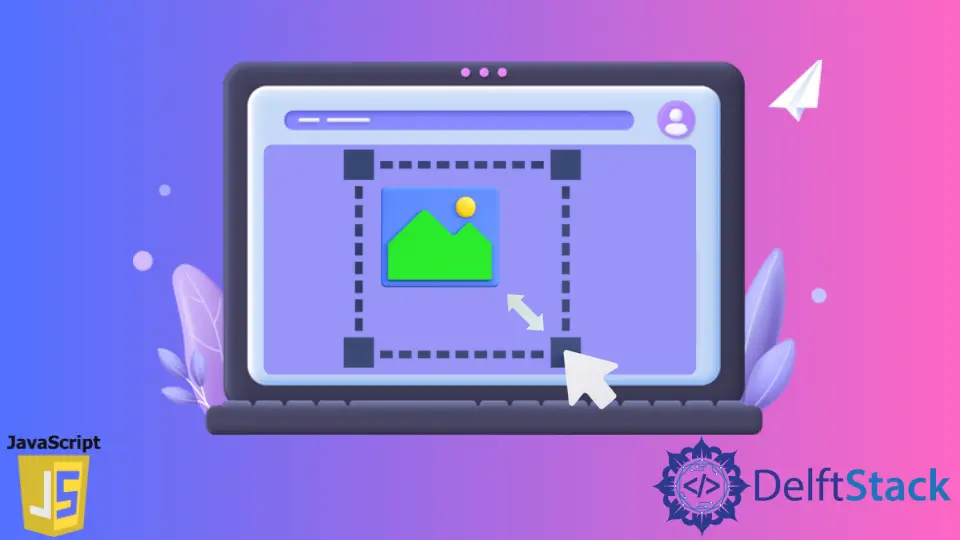
Graphics are used to describe aspects of images which is an important part of any web application. HTML offers two ways of creating graphics. The first is canvas
, and the other is SVG
. In today’s article, we will learn how to create graphics, especially how to draw a picture in HTML with canvas and JavaScript.
Resize Images by canvas
in HTML Using JavaScript
Canvas is a standard HTML element that is used for drawing graphics in web applications. It’s nothing more than a rectangular area on the page with no border or content. Users can use this rectangular area to draw graphics.
Graphics rendered on canvas are different from normal HTML and CSS styles. The entire canvas with all the graphics it contains is treated as a single DOM element.
Methods of canvas
in HTML
getContext()
: This is a built-in method provided bycanvas
that returns the drawing context on the canvas depending on thecontextType
. If the context identifier is not supported or is already configured,null
is returned. Supported context types are2d
,webgl
,webgl2
, andbitmaprenderer
.drawImage()
: This is a built-in method provided bycanvas
that helps draw the image on the canvas in various ways.
Syntax of drawImage()
context.drawImage(
$image, $sx, $sy, $sWidth, $sHeight, $dx, $dy, $dWidth, $dHeight);
Parameters
$image
: It is a mandatory parameter that specifies the image source that needs to be drawn into the canvas. Image source can be anything likeCSSImageValue
,HTMLImageElement
,SVGImageElement
, etc.$sx
: It is an optional parameter that specifies theX
or horizontal coordinate of the source image. It will be measured as a rectangle from the top left corner to draw into the destination image.$sy
: It is an optional parameter that specifies theY
or vertical coordinate of the source image. It will be measured as a rectangle from the top left corner to draw into the destination image.$sWidth
: It is an optional parameter that specifies the width of the source image. It will be measured as a rectangle from the top left corner to draw into the destination image.$sHeight
: This is an optional parameter that specifies the height of the source image. It will be measured as a rectangle from the top left corner to draw into the destination image.$dx
: It is a mandatory parameter that specifies theX
or horizontal coordinate of the destination image. It will be measured as a rectangle from the top left corner where the source image is placed.$dy
: It is a mandatory parameter that specifies theY
or vertical coordinate of the destination image. It will be measured as a rectangle from the top left corner where the source image is placed.$dWidth
: This is an optional parameter that specifies the width of the destination image. It will be measured as a rectangle from the top left corner. If no value is specified, the default width is the width of the source image.$dHeight
: It is an optional parameter that specifies the height of the destination image. It will be measured as a rectangle from the top left corner. If not specified, the default height is the height of the source image.
Steps to Resize Image Using JavaScript Canvas
-
Get the context of the Canvas.
-
Set height of the Canvas.
-
Create mew canvas element for performing resize & get context of it.
-
Set the width and height of the newly created Canvas.
-
Draw the image on new Canvas.
-
Draw the image on final Canvas using newly created Canvas.
Example Code:
<img src="https://images.pexels.com/photos/3408744/pexels-photo-3408744.jpeg?auto=compress&cs=tinysrgb&dpr=2" width="500" height="400">
<canvas id="canvas" width=1000></canvas>
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const img = new Image();
img.src =
'https://images.pexels.com/photos/3408744/pexels-photo-3408744.jpeg?auto=compress&cs=tinysrgb&dpr=2';
img.onload = function() {
// set height proportional to destination image
canvas.height = canvas.width * (img.height / img.width);
// step 1 - resize to 75%
const oc = document.createElement('canvas');
const octx = oc.getContext('2d');
// Set the width & height to 75% of image
oc.width = img.width * 0.75;
oc.height = img.height * 0.75;
// step 2, resize to temporary size
octx.drawImage(img, 0, 0, oc.width, oc.height);
// step 3, resize to final size
ctx.drawImage(
oc, 0, 0, oc.width * 0.75, oc.height * 0.75, 0, 0, canvas.width,
canvas.height);
}
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn