How to Replace New Line Using JavaScript
-
Use
replaceAll()
to Replace Newline With the<br/>
in JavaScript -
Use
replace()
to Replace Newline With the<br/>
in JavaScript -
Difference Between
replaceAll()
andreplace()
in JavaScript
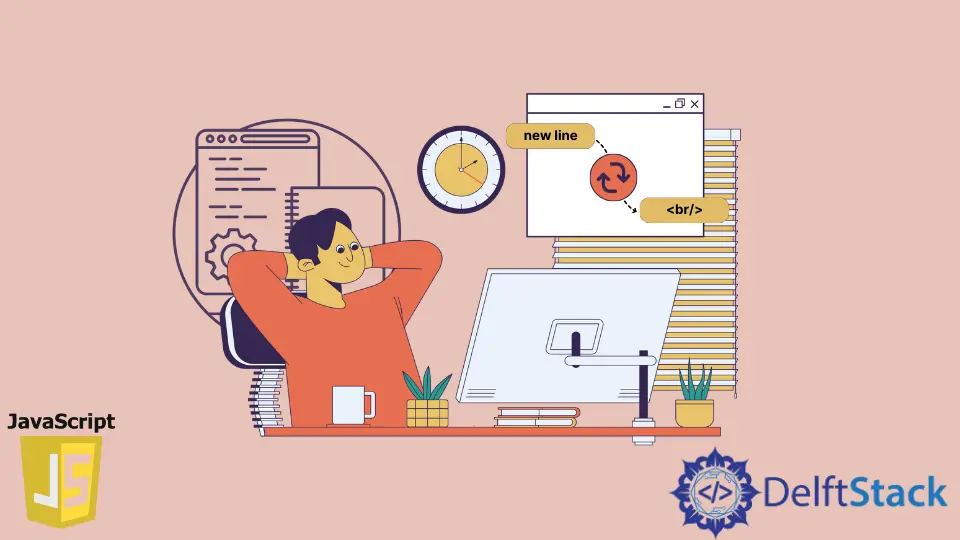
JavaScript provides two functions to replace a new line with HTML <br/>
in the string. In today’s post, we will learn both the functions to replace newline (\n
) with an HTML break tag (<br/>
).
Use replaceAll()
to Replace Newline With the <br/>
in JavaScript
The replaceAll()
is an in-built method provided by JavaScript which takes the two input parameters and returns a new String in which all matches of a pattern are replaced with a replacement. The first input parameter is a pattern which is usually a string
or RegExp
.
Depending on the first input params, the replacement can be a string or a function that needs to be called for each match.
Syntax:
replaceAll(regexp | substr, newSubstr | replacerFunction)
The RegEx
or pattern
is an object or literal with the global flag. All the matches are replaced with either a new substring or the value returned by the specified replacement function.
RegExp provided must contain the global flag g
without generating a TypeError: replaceAll must be called with a regular expression
.
If a string is passed instead of a RegEx, substr
is a string that should be replaced with newSubstr
. It is not interpreted as a regular expression and is treated as a literal string.
The second parameter, replacerFunction
or newSubstr
, is the string that replaces the specified substring (original string) with the specified regexp
or substr
parameter. Several special replacement patterns are allowed.
The replacement
or replacerFunction
function is called to create the new substring. This function replaces (one or all) matches with the specified RegEx or substring.
The output of the replaceAll()
is a new string that contains all matches of a pattern replaced by a replacement.
Find more information in the documentation for the method replaceAll()
.
<div style="white-space: pre-line" id="para">Hello World.
Welcome to my blog post.
Find out all the programming-related articles in one place.
</div>
const p = document.getElementById('para').innerText;
console.log(p.replaceAll('\n', '<br/>'));
const regex = /(?:\r\n|\r|\n)/g;
console.log(p.replaceAll(regex, '<br/>'));
In the example above, we replaced the new line with the string and applied <br/>
to the declaration as a new string. RegEx can be used if you want to replace a complex string.
This automatically finds the appropriate pattern and replaces it with the replace
function or the replace
string.
Output:
"Hello World.<br/>Welcome to my blog post.<br/>Find out all the programming-related articles in one place.<br/>"
"Hello World.<br/>Welcome to my blog post.<br/>Find out all the programming-related articles in one place.<br/>"
Use replace()
to Replace Newline With the <br/>
in JavaScript
The replace()
is an in-built method provided by JavaScript which takes the two input parameters and returns a new String in which all or first matches of a pattern are replaced with a replacement.
The first input parameter is a pattern which is usually a string
or RegExp
. Depending on the first input params, the replacement can be a string or a function that needs to be called for each match.
The pattern is the string; it only replaces the first match.
Syntax:
replace(regexp | substr, newSubstr | replacerFunction)
The RegEx
or pattern
is an object or literal with the global flag. All the matches are replaced with either a new substring or the value returned by the specified replacement function.
RegExp provided must contain the global flag g
without generating a TypeError: replaceAll must be called with a regular expression
.
If a string is passed instead of a RegEx, substr
is a string that should be replaced with newSubstr
. It’s not interpreted as a regular expression and is treated as a literal string.
The second parameter, replacerFunction
or newSubstr
, is the string that replaces the specified substring (original string) with the specified regexp
or substr
parameter. Several special replacement patterns are allowed.
The replacement
or replacerFunction
function is called to create the new substring. This function replaces (one or all) matches with the specified RegEx or substring.
The replace()
output is a new string containing all matches of a pattern replaced by a replacement.
Find more information in the documentation for the method replace()
.
<div style="white-space: pre-line" id="para">Hello World.
Welcome to my blog post.
Find out all the programming-related articles in one place.
</div>
const p = document.getElementById('para').innerText;
console.log(p.replace('\n', '<br/>'));
const regex = /(?:\r\n|\r|\n)/g;
console.log(p.replace(regex, '<br/>'));
In the example above, we replaced the new line with the string and applied <br/>
to the declaration as a new string. RegEx can be used if you want to replace a complex string.
This automatically finds the appropriate pattern and replaces it with the replace
function or the replace
string.
Output:
"Hello World.<br/>Welcome to my blog post.
Find out all the programming-related articles in one place.
"
"Hello World.<br/>Welcome to my blog post.<br/>Find out all the programming-related articles in one place.<br/>"
Difference Between replaceAll()
and replace()
in JavaScript
The sole difference between replaceAll
and replace
is that if the search argument is a string, the prior method only replaces the first occurrence, while the replaceAll()
method replaces all matching occurrences instead of the first with the replacement value or function.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn