JavaScript Readable Stream
-
Install
ReadableStream
in JavaScript -
Example of
ReadableStream
Using.getReader()
-
Example of
ReadableStream
UsingFetch
API
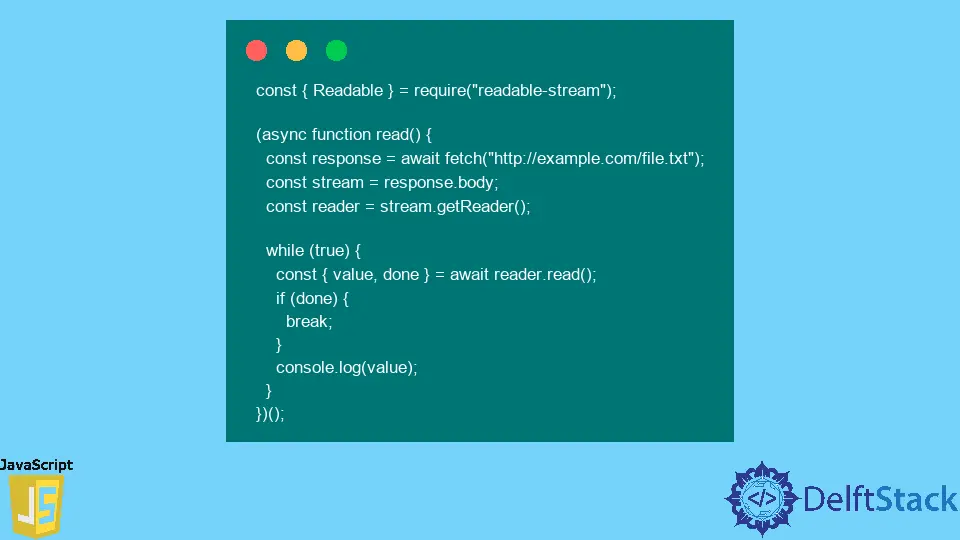
A ReadableStream
is a JavaScript object that allows you to read data from a source in a stream-like fashion. It is often used to read large amounts of data, such as a file or network stream, and can be used in conjunction with other APIs, such as the Fetch
API or the Response
object in the browser.
To create a ReadableStream
, you can use the new ReadableStream()
constructor, which takes in a source and some options as arguments. You can then use the stream’s .getReader()
method to get a reader
object, which you can use to read the data from the stream using methods like .read()
and .cancel()
.
One of the key benefits of using a ReadableStream
is that it allows you to process the data as it is being read rather than wait for the entire stream to be fully loaded. This can be particularly useful when working with large data sets or reading data from a slow source.
Overall, the ReadableStream
is a powerful tool for working with streaming data in JavaScript and can be a useful addition to your toolkit when developing web applications.
Install ReadableStream
in JavaScript
The ReadableStream
constructor is not a part of the JavaScript core API, but libraries are available that provide this functionality. One popular library is the readable-stream
package.
To use the readable-stream
package in your Node.js project, you can install it using npm
by running the following command:
npm install readable-stream
Then in your code, you can import the Readable
class and use it to create a new ReadableStream
object.
const {Readable} = require('readable-stream');
Example of ReadableStream
Using .getReader()
Here is an example of creating a ReadableStream
and reading data using the .getReader()
method.
In this example, the start
method uses a setTimeout
function to asynchronously push new values into the stream every 100 milliseconds.
This code creates a ReadableStream
that generates a series of numbers, starting at 0
and ending at 99
. The stream is created using the start
method, which is called every time the stream is ready to start reading data.
The .getReader()
method is used to get a reader
object, which is then used in an async
function to read the data from the stream. The .read()
method is called repeatedly in a loop, and the loop is terminated when the done
property of the returned object is true
, indicating that the stream has been closed.
// const { Readable } = require("readable-stream");
const stream = new ReadableStream({
start(controller) {
let i = 0;
function push() {
controller.enqueue(i++);
if (i < 100) {
setTimeout(push, 100);
} else {
controller.close();
}
}
push();
},
});
const reader = stream.getReader();
(async function read() {
while (true) {
const {value, done} = await reader.read();
if (done) {
break;
}
console.log(value);
}
})();
Output:
Example of ReadableStream
Using Fetch
API
Here is another example of using a ReadableStream
, this time using the Fetch
API to read data from a remote resource.
In this example, we use the fetch
function to request a remote resource and get a Response
object. The Response
object has a body
property that is a ReadableStream
, which we can use to read the data from the response.
We then use the .getReader()
method to get a reader
object and read the data from the stream using the same async
function as in the previous example. This time, the data being read is the contents of the file.txt
file on the server.
const {Readable} = require('readable-stream');
(async function read() {
const response = await fetch("http://example.com/file.txt");
const stream = response.body;
const reader = stream.getReader();
while (true) {
const { value, done } = await reader.read();
if (done) {
break;
}
console.log(value);
}
})();
Output:
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn