Introduction to JavaScript Profiler
- What is a JavaScript Profiler?
- Importance of Using a JavaScript Profiler
- How to Use JavaScript Profiler in Chrome DevTools
- Analyzing Profiling Results
- Best Practices for JavaScript Profiling
- Conclusion
- FAQ
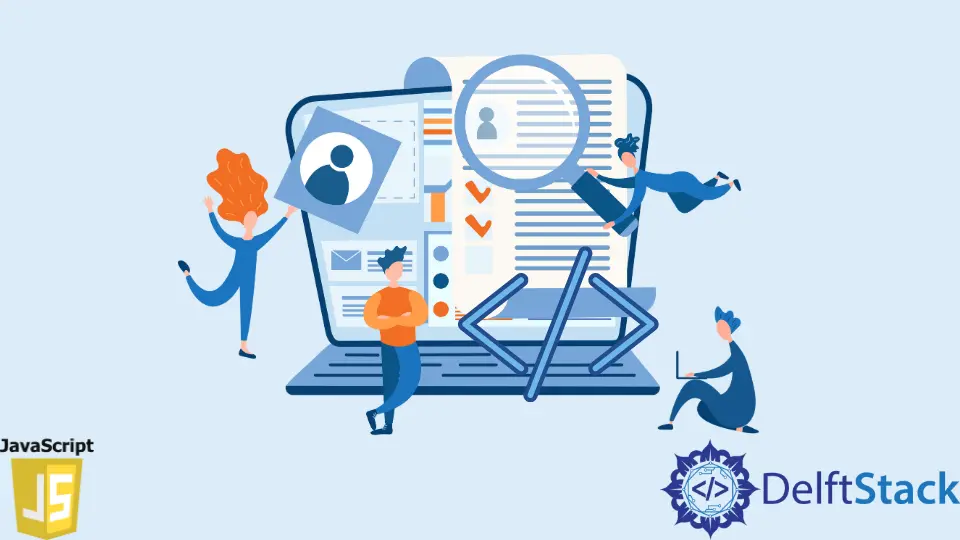
JavaScript profiling is a crucial aspect of web development that helps developers understand the performance of their applications. Whether you’re building complex web applications or simple websites, knowing how to optimize your JavaScript code can lead to a smoother user experience.
In this tutorial, we will delve into the JavaScript profiler, exploring its importance and practical usage. We will discuss how profiling can help identify bottlenecks, improve load times, and enhance overall application performance. By the end of this article, you will have a solid understanding of what a JavaScript profiler is, why it matters, and how to effectively use it to optimize your code.
What is a JavaScript Profiler?
A JavaScript profiler is a tool that helps developers analyze the performance of their JavaScript code. It provides insights into how long functions take to execute, how many times they are called, and how memory is being used. By leveraging this data, developers can identify slow-running code, memory leaks, and other performance issues that may affect user experience.
Using a profiler can be likened to having a magnifying glass that reveals the hidden inefficiencies in your code. It enables you to see the bigger picture and make informed decisions on where to focus your optimization efforts. Most modern browsers come equipped with built-in profiling tools, such as Chrome’s DevTools, making it easier than ever to get started with performance analysis.
Importance of Using a JavaScript Profiler
The importance of using a JavaScript profiler cannot be overstated. Here are some reasons why profiling is essential for developers:
-
Performance Optimization: Profilers help you identify which parts of your code are slowing down your application. By pinpointing these bottlenecks, you can optimize your code for better performance.
-
Memory Management: Memory leaks can severely impact application performance. Profiling tools can help you track memory usage and find leaks, ensuring that your application runs smoothly.
-
User Experience: A faster application leads to a better user experience. By optimizing your JavaScript code with the help of a profiler, you can ensure that users enjoy a seamless experience on your website.
-
Debugging: Profilers can also assist in debugging by providing detailed information about function calls and execution times. This information can be invaluable when trying to track down performance-related issues.
How to Use JavaScript Profiler in Chrome DevTools
Using the JavaScript profiler in Chrome DevTools is straightforward. Follow these steps to get started:
- Open Chrome and navigate to the webpage you want to analyze.
- Right-click on the page and select “Inspect” to open DevTools.
- Go to the “Performance” tab.
- Click on the record button (circle) to start profiling your application.
- Interact with your webpage as you normally would to generate data.
- Stop the recording by clicking the button again.
Once you stop the recording, you’ll see a detailed breakdown of your application’s performance. This includes CPU usage, memory consumption, and a flame graph that visualizes the execution time of your JavaScript functions.
Analyzing Profiling Results
After recording your profiling session, the next step is to analyze the results. Here’s how you can interpret the data:
-
Flame Graph: This visual representation shows the call stack of your functions. The wider the bar, the longer the function took to execute. Look for functions with wide bars, as these are your potential bottlenecks.
-
Call Tree: This section provides a hierarchical view of function calls. You can see which functions called others and how much time each function consumed. This is particularly useful for understanding complex interactions between functions.
-
Stack Chart: This chart displays CPU usage over time. Spikes in CPU usage can indicate performance issues that need to be addressed.
By carefully analyzing these results, you can identify areas for optimization, such as reducing function execution times or refactoring inefficient code.
Best Practices for JavaScript Profiling
To make the most of your JavaScript profiling efforts, consider the following best practices:
-
Profile Regularly: Make profiling a regular part of your development process. This will help you catch performance issues early and maintain a high-quality user experience.
-
Focus on Critical Paths: Identify the most critical parts of your application that impact user experience and prioritize profiling these areas.
-
Use Multiple Tools: While Chrome DevTools is powerful, consider using other profiling tools like Firefox’s Developer Edition or third-party tools for a more comprehensive analysis.
-
Test on Real Devices: Performance can vary across devices and browsers. Test your application on real devices to get accurate profiling data.
-
Iterate and Optimize: Profiling is an ongoing process. After making optimizations, re-profile your application to see the impact of your changes.
Conclusion
JavaScript profiling is an invaluable skill for developers looking to optimize their web applications. By understanding how to use profiling tools effectively, you can identify performance bottlenecks, manage memory usage, and enhance the overall user experience. Regular profiling, combined with best practices, will ensure your applications run smoothly and efficiently. As you continue to explore the world of JavaScript, remember that performance matters, and a well-optimized application can make all the difference.
FAQ
-
What is a JavaScript profiler?
A JavaScript profiler is a tool used to analyze the performance of JavaScript code, helping developers identify slow functions and memory leaks. -
How do I access the JavaScript profiler in Chrome?
You can access the JavaScript profiler in Chrome by opening DevTools, navigating to the “Performance” tab, and starting a recording session. -
Why is profiling important for web development?
Profiling is important because it helps optimize application performance, manage memory usage, and enhance user experience. -
Can I use profiling tools other than Chrome DevTools?
Yes, there are other profiling tools available, such as Firefox’s Developer Edition and third-party options that provide additional insights. -
How often should I profile my JavaScript code?
It is advisable to profile your code regularly, especially during the development phase, to catch performance issues early.