JavaScript Pointers
- Pass by Value for Primitive Object in JavaScript
- Pass by Reference of Composite Objects in JavaScript
-
Use the
Spread Operator
for Deep Copy in JavaScript -
Use the
JSON.parse()
andJSON.stringify()
for the Deep Copy of an Object -
Use the
Object.assign()
for Deep Copy of Objects in JavaScript -
Use the
Object.slice(0)
for Deep Copy of Arrays in JavaScript -
Use the
lodash
to Make a Deep Cloning in JavaScript
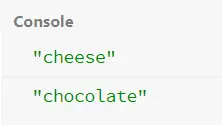
In a programming language like C++, we often initialize the pointer to get the content location. But in the case of JavaScript, we do not get conventions of pointing an address directly.
In JavaScript, we have 2 kinds of data types, which are primitive data types, and the other is composite data types.
Usually, the primitive data types follow to maintain the pass by value
. And the composite data types allow pass by reference
; here is where the shallow copy
works.
A shallow copy of an object takes the target object’s reference, and whatever modification is made affects the original and the copy.
Here, we will see how work works pass by value
and pass by reference
. We will also try to make deep copies
of composite objects.
Specifically, we will cover the spread operator
, using lodash
, JSON.parse and JSON.stringify
, slice()
, Object.assign()
functionalities.
Pass by Value for Primitive Object in JavaScript
When dealing with a primitive object, a general copy of targeted objects is maintained. And in this drive, the original object is not affected even if any modification is made to the copy.
This process is named after the pass by value
and performs the deep copy
by default.
var x = 7;
var y = x;
x = 42;
console.log(x);
console.log(y);
Output:
The modification of the object y
does not impact the x
object.
Pass by Reference of Composite Objects in JavaScript
In the case of composite objects, the reference is passed as a copy. As a result, any modification made to the copy object impacts the original object.
This method is also known as shallow copy
or the pass by reference
.
var x = {num: 42};
var y = x;
y.num = 7;
console.log(x.num);
console.log(y.num);
Output:
Here, we have an object y
that takes a copy of x
. But the inner picture only takes the object x
reference.
So, when we alter the contents in the copy, the effect will be on the original object because both objects here point to the same reference.
To solve this case of shallow copy, we have some propositions and works regardless of the object type (either array or object) or need individual attention.
For example, the spread operator
works for deep copy
in both the array and an object. Let’s follow the following portion for a clear view.
Use the Spread Operator
for Deep Copy in JavaScript
The Spread Operator is a convention that spreads an object to another object. This copy method is accurate, and any modification keeps the objects intact or as they are supposed to be altered.
Again, the spread operator works for an array and an object. Let’s check the following instance.
var x = [1, 2, 3];
var y = [...x];
y[2] = 5;
console.log(x[2]);
console.log(y[2]);
Output:
Using a spread operator for a nested object will be tedious as you will have to access the keys
of every level. In this case, the easier solution is to use the upcoming method.
Use the JSON.parse()
and JSON.stringify()
for the Deep Copy of an Object
Whenever we work with an object, we access the contents triggering the keys
. The JSON.parse(JSON.stringify(Object))
makes the tough work a lot better.
It makes a deep copy of the targeted object, and we can also modify the contents.
var json = {num: {val: 7}};
var collect = JSON.parse(JSON.stringify(json));
collect.num.val = 42;
console.log(json.num.val);
console.log(collect.num.val);
Output:
Use the Object.assign()
for Deep Copy of Objects in JavaScript
The Object.assign()
method makes an accurate copy of the original object.
In this way of solving the problem, we need 2 parameters; one is a pair of blank curly brace {}
that is followed by the targeted object. Let’s check the instance for a better understanding.
var x = {'food': 'cheese', 'sport': 'badminton'};
var y = Object.assign({}, x);
y.food = 'chocolate';
console.log(x.food);
console.log(y.food);
Output:
Use the Object.slice(0)
for Deep Copy of Arrays in JavaScript
The method slice()
initiating without any argument or zero can make a deep copy. This is one of the familiar and most used methods for dealing with arrays.
Other than this, you can also use the map
to copy an array. Check this documentation for a further query.
The examples will be more explanatory.
var x = [1, 3, 4];
var y = x.slice(0);
y[2] = 5;
console.log(x);
console.log(y);
Output:
Use the lodash
to Make a Deep Cloning in JavaScript
For using this solution, you will require to load the lodash 4.x
library. We have examined the example in the jsbin editor.
Here, you will find the lodash 4.x
in the library section.
var x = [1, 2, 3];
var y = _.cloneDeep(x);
y[1] = 4;
console.log(y[1]);
console.log(x[1]);
Output: