How to Ping Server in JavaScript
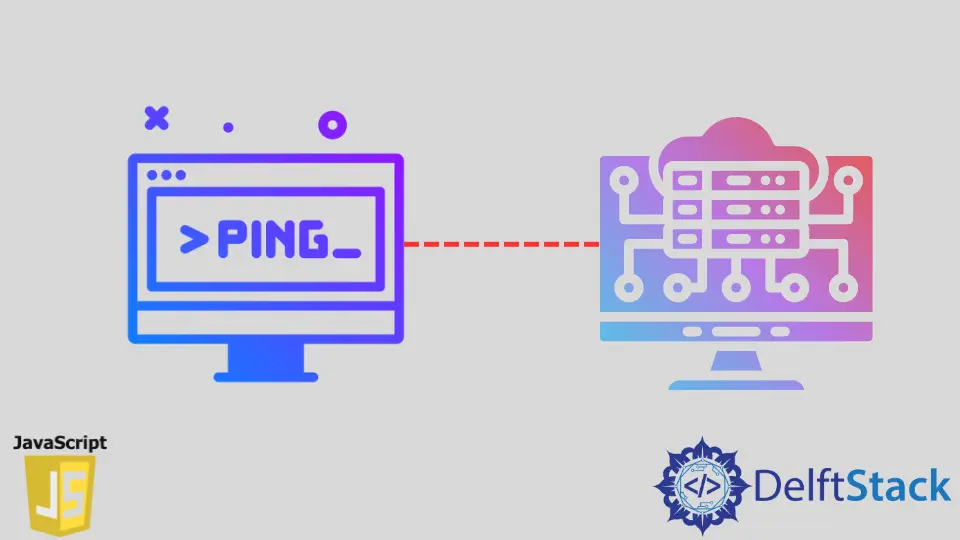
When it comes to web development, checking the availability of a server or an endpoint is essential. One common method to achieve this is by pinging the server.
In this article, we will discuss how to ping a server or an URL using JavaScript. Whether you are a beginner or an experienced developer, understanding this process can enhance your ability to create more responsive applications. We will explore various methods to implement this functionality, including using the Fetch API and XMLHttpRequest. By the end of this article, you will have a clear understanding of how to effectively ping a server using JavaScript.
Using the Fetch API to Ping a Server
The Fetch API is a modern way to make network requests in JavaScript. It provides a simple interface for fetching resources across the network. To ping a server, you can use the Fetch API to send a request to the desired URL. Here’s a straightforward example:
fetch('https://example.com')
.then(response => {
if (response.ok) {
console.log('Server is reachable');
} else {
console.log('Server responded with an error');
}
})
.catch(error => {
console.log('Server is not reachable', error);
});
In this code, we initiate a fetch request to https://example.com
. If the server responds successfully, we log a message indicating that the server is reachable. If the response indicates an error, we log that as well. If there’s a network issue or the server is down, the catch block will handle the error, letting us know that the server is not reachable.
Output:
Server is reachable
The Fetch API is promise-based, making it easy to handle asynchronous operations. This means you can chain multiple .then()
methods to handle different scenarios, such as processing the response data or handling errors. It’s also worth noting that the Fetch API supports CORS, which is crucial for web applications that need to access resources on different domains.
Using XMLHttpRequest to Ping a Server
Another method to ping a server in JavaScript is by using the older XMLHttpRequest object. While less modern than the Fetch API, it’s still widely used and supported in all browsers. Here’s how you can implement it:
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com', true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
console.log('Server is reachable');
} else {
console.log('Server responded with an error');
}
}
};
xhr.onerror = function() {
console.log('Server is not reachable');
};
xhr.send();
In this example, we create a new XMLHttpRequest object and open a GET request to https://example.com
. The onreadystatechange
event is triggered whenever the ready state changes. When the request is complete (readyState 4), we check the status code. A status code of 200 indicates a successful response, while any other code indicates an error. The onerror
event handles any network errors, informing us if the server is unreachable.
Output:
Server is reachable
Using XMLHttpRequest gives you more control over the request process, such as tracking progress or aborting requests. However, it can be more verbose compared to the Fetch API. Despite this, it remains a powerful tool for developers who need to ensure compatibility with older browsers or specific use cases.
Conclusion
Pinging a server in JavaScript can be accomplished using either the Fetch API or XMLHttpRequest. Each method has its advantages and use cases. The Fetch API is more modern and easier to use, while XMLHttpRequest offers more control over the request lifecycle. By understanding these methods, you can create more robust web applications that can effectively check server availability. Whether you are building a simple website or a complex web application, knowing how to ping a server is a valuable skill in your development toolkit.
FAQ
- What is the purpose of pinging a server in JavaScript?
Pinging a server helps determine if the server is reachable and responding to requests, which is essential for maintaining a good user experience.
-
Can I ping any URL using these methods?
Yes, you can ping any publicly accessible URL, but be aware of CORS restrictions when making requests to different domains. -
Is the Fetch API supported in all browsers?
The Fetch API is supported in most modern browsers. However, for older browsers like Internet Explorer, you may need to use XMLHttpRequest. -
How do I handle errors when pinging a server?
Both methods include error handling; the Fetch API uses the.catch()
method, while XMLHttpRequest uses theonerror
event. -
What if I need to ping a server continuously?
You can use setInterval or setTimeout to repeatedly ping the server at specified intervals.