Whether JavaScript Is a Pass by Reference or a Pass by Value Type
- Primitive Data Types in JavaScript Are Pass by Value
- Non-Primitive Values in JavaScript Are Pass by Reference
- JavaScript Is Both Pass by Value and Pass by Reference
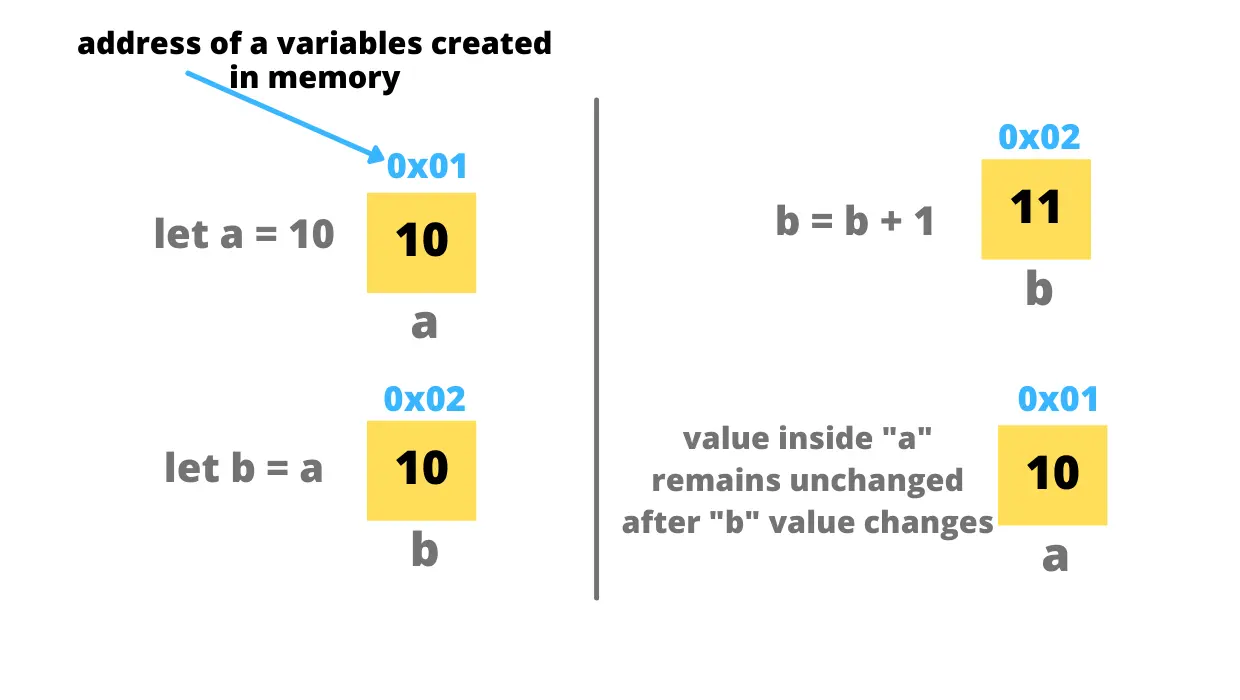
Before diving deep into whether the JavaScript programming language is a pass by reference type or not, let’s first see the difference between pass by value and pass by reference.
These things usually come into the picture when we are doing assignment operations or dealing with the functions themselves. Since most of the time, we perform assignment operations, create various functions, and pass multiple variables to these functions, knowing how these things internally work would give us a deeper understanding of the programming language.
Whenever we assign or pass the value of a variable to another variable or function, it’s known as pass by value. When we assign or pass the address of a variable to another variable or function, it’s known as pass by reference.
Now that we’ve seen the basic definition of the pass by value and pass by reference types, let’s further understand how they are used in the following examples below.
Primitive Data Types in JavaScript Are Pass by Value
Take note that there are two kinds of data types in JavaScript: the primitive data type and the non-primitive data type. The primitive data types consist of numbers, boolean, strings, undefined, and null.
These data types do not have any pre-defined methods as we have in objects or arrays. The primitive data types are immutable; this means that whenever you store or assign the value of one primitive variable to another and make some modifications to the value of the second variable, the value of the first variable will not change. It will remain as it is until and unless you change the value of that variable itself. Only then will the second variable be altered.
You can find more information related to primitive data types on MDN.
// Example 1
let a = 10;
let b = a;
b = b + 1;
console.log(a);
console.log(b);
// Example 2
let name = 'Google';
let greet =
(val) => {
val = 'Hi ' + name + '!';
return val;
}
console.log(name);
console.log(greet(name));
Output:
10
11
Google
Hi Google!
The code above can be better understood with the help of the illustration below. Note that it only explains the first code example; the second example will work mostly the same way as that of example one.
In our first example, we have two variables: a
and b
. We have assigned a numerical value of 10
to our variable a
; this will allocate a space for variable a
in the memory, represented by the yellow color having an address 0x01
(which is just an arbitrary address taken for this example).
Then, we assign the variable a
to the variable b
. This process will allocate the new memory space for variable b
. Here, it will store the value of variable a
, which is 10
.
From the example above, we can clearly see that the address of both the variables a
and b
is different, which means that they have assigned different spaces in memory. This result implies that whenever you change or modify the value of variable b
as b = b + 1
, the value inside variable a
will be not be changed. Only the value of variable b
will change, and it will become 11
since we are incrementing it by one.
In our second example, we have a variable name
having a value Google
inside. We also have a function called greet()
, which takes a string value as an argument and returns a new string. As an argument to this function, we pass the variable a
(notice that passing a variable means passing its value and not its address). The Google
value will be stored inside the function’s local variable named val
.
Currently, the variable val
holds the value Google
. In the function, we are changing this value to Hi Google!
and then returning this value. Now, if you output both the name
variables value and the functions, along with the returned value, they will both be different. The name
variables remain unchanged because both variables name
and val
are stored at different locations, and we are only passing the value Google
to the function and the address.
Previously, we have seen the definition of pass by value, which said we only pass the value of the variable during an assignment or while passing it to a function. The two examples above demonstrated it in practice.
Non-Primitive Values in JavaScript Are Pass by Reference
The non-primitive data types consist of arrays, objects, or classes. These data types do have pre-defined built-in functions that allow us to manipulate the data inside the arrays or the objects.
The primitive data types are mutable; this means that the data types which can be modified later are known as mutable. Additionally, the non-primitive data types are called by reference. Let’s understand this with the example below.
let a = [1, 2];
let b = a;
b.push(3);
console.log(a)
console.log(b)
Output:
[ 1, 2, 3 ]
[ 1, 2, 3 ]
The image for the example above is as shown below.
In this example, we created an array a
with two elements: [1,2]
. Since an array is a non-primitive data type, the variable a
will not store all the array elements. Instead, the values [1,2]
will be stored at another location in the memory. In this case, the starting address of that array, in this case, 0x01
, will be stored in variable a
, as shown in the diagram above. Since the variable a
has the address where the actual array elements are stored, it will point to that location.
When we assign variable a
to variable b
, we are storing the address present inside a
into variable b
. Now, variable b
will also point to the same memory location, 0x011
as that of variable a
, since both of them have the same memory address. So, if you change the elements of an array pointed by b
, then a
will also get affected because both are pointing to the same location.
Here, we are pushing a new element into the array using variable b
such as b.push(3)
. Now, variable b
will traverse the entire array starting from the first element, 1
, until the last element, 2
. After the last element, it will insert the new element 3
into the array. If you print both the variables a
and b
, you will see that they will both show the same value as [1,2,3]
because of referencing.
This is known as pass by reference because we are passing the memory address itself and not the value.
JavaScript Is Both Pass by Value and Pass by Reference
The JavaScript programming language supports both pass by value and pass by reference. Primitive values such as numbers, boolean, strings, undefined, and null are all passed by values. Non-primitive types, such as arrays, objects, and classes, are passed by reference.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn