How to Call a JavaScript Function Using onclick Event
-
Calling JavaScript Function Using the
onclick
Event Handler - Attaching a JavaScript Function to an HTML Element Using Pure JavaScript
-
Defining a Function on the
onclick
Event Handler in the HTML Element -
Calling a JavaScript Function Using
addEventListener
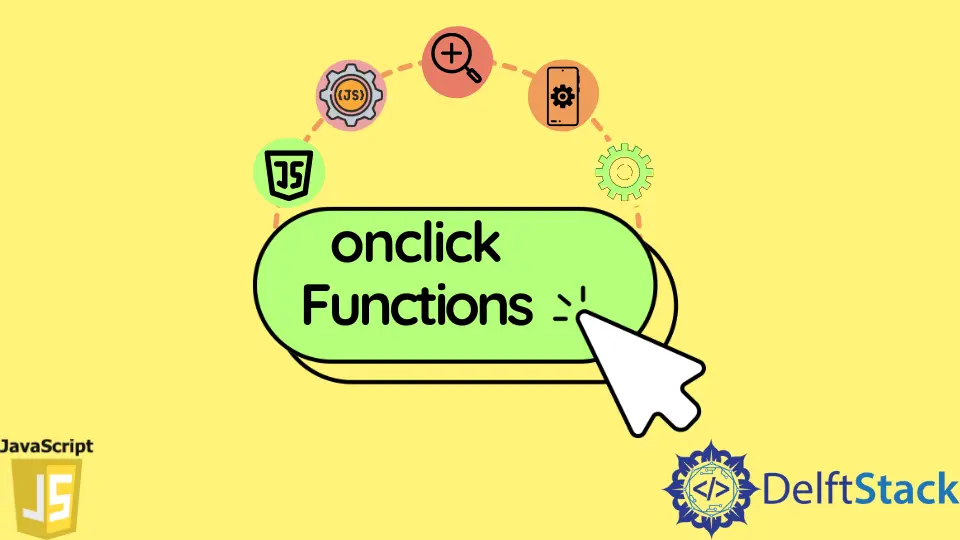
Even if we design static HTML content for a webpage, we may want to make it user interactive. HTML elements, by default, are dumb. The <a>
tag is user interactive but is mainly used to load a specific path. HTML elements like div
can be made user interactive by adding an event handler to them. We will be discussing one such event handler called the onclick
event handler. There are a couple of ways in which we can add it to an HTML element.
Calling JavaScript Function Using the onclick
Event Handler
We can bind a JavaScript function to a div
using the onclick
event handler in the HTML or attaching the event handler in JavaScript. Let us refer to the following code in which we attach the event handler to a div
element.
<div id="interactive-div" onclick="changeMyColor('interactive-div')" style="cursor: pointer;">Click me</div>
function changeMyColor(id) {
var el = document.getElementById(id);
el.style.color = 'blue';
}
The div
element does not accept any click events by default. In the code, we bind a JavaScript function changeMyColor()
to the onclick
event handler of the div
. It now makes the div
clickable. Even though we define an onclick
event handler to a div
, still the user will not know if the div
accepts a click or not unless he clicks. It is a good UI/UX to have the cursor marked differently once the user hovers on our target div
. For this purpose, we assign an inline style style="cursor: pointer;"
to the div
element. Once the user hovers the div
, the cursor will show a hand sign indicating it as clickable.
function changeMyColor(id) {
var el = document.getElementById(id);
el.style.color = 'blue';
}
In the JavaScript code, we define the function changeMyColor()
passed to the onclick
event of the div
. The function takes the id of the div
as a parameter. It selects the element using the document.getElementById(id)
and changes the font color of the element to blue
using the line el.style.color = "blue";
. Hence, we assigned a JavaScript function to the div
and made it clickable.
Attaching a JavaScript Function to an HTML Element Using Pure JavaScript
In the last approach, we declared the onclick
event handler in the HTML. There is a way to keep the HTML clean and attach a JavaScript function to the div
or the corresponding HTML element without specifying any onclick
event handler in the HTML. Here, we assign the click
event in JavaScript rather than using HTML. Hence, this approach is purely JavaScript-based and keeps the HTML clean. Such a style of programming is called Unobtrusive JavaScript. Refer to the following code to understand the concept better.
<div id="interactive-div" style="cursor: pointer;">Click me</div>
window.onload =
function() {
var el = document.getElementById('interactive-div');
el.onclick = changeMyColor;
}
function changeMyColor() {
var el = document.getElementById('interactive-div');
el.style.color = 'blue';
}
Referring to the above code, we have kept the HTML similar to the previous code sample. We did not add any onclick
event handler in the HTML. Instead, we defined the onclick
event handler in JavaScript and attached the same function changeMyColor
in the JavaScript code. There are a couple of things to note here:
- We attach the
onclick
event listener inside thewindow.onload
. If we do not use thewindow.onload
method, it will throw an error sayingUncaught TypeError: Cannot set property 'onclick' of null
. This happens because, thedocument.getElementById("interactive-div")
tries to query for the element withid
interactive-div
while the HTML is loading. As the<script>
tag comes in the<head>
section, the JavaScript code executes the<script>
tag contents before actually loading thediv
that resides in the<body>
tag. Hence, thegetElementById()
function will not find the element and returnsnull
. Hence it spills out an error saying that it cannot setonclick
of null. - Another point to note is that we assign the function to the
onclick
event handler without the parenthesis()
. If we writeel.onclick = changeMyColor();
instead ofel.onclick = changeMyColor;
, it will execute the function on load of the HTML which is not what we want. Hence, we pass the function reference ofchangeMyColor
to theonclick
event handler. Once the user triggers theonclick
event by clicking on thediv
, the function callchangeMyColor
happens. And thediv
turns blue.
Defining a Function on the onclick
Event Handler in the HTML Element
There are a few more ways to call a JavaScript function on the onclick
event. We can define the method in HTML. But it is not a recommended way as it makes the HTML untidy. Refer to the following code to understand better.
<div onclick="(function() { alert('hello there'); })()" style="cursor: pointer;">click me!</div>
On clicking the div
, the above code will popup the hello there
text. Here, we code the function definition inline in the HTML. It is an anonymous inline method. And we cannot use the same function elsewhere as it does not have an associated name. Lengthier function definitions will be cumbersome and make the code readability poor.
Calling a JavaScript Function Using addEventListener
One way to add the onclick
event handler was to use the .onclick()
function. There is another way that is more generic and can be used to add many more event listeners. For that we use .addEventListener()
function of JavaScript. This function can be used on an element
, document
interface or even a window
object. We can use the .addEventListener()
function to set up a method invocation when a particular event
is triggered.
- Parameters: It takes a couple of mandatory parameters: the type of an event, for example,
click
etc., and thelistener
function. Thelistener
function gets executed when the event triggers. - Usage: Refer to the following code.
<div style="cursor: pointer;" id="interactive-div">click me!</div>
document.addEventListener('DOMContentLoaded', function() {
document.getElementById('interactive-div')
.addEventListener('click', function() {
alert('hello');
});
});
This coding style is purely JavaScript-based unobtrusive JavaScript programming. Here, we have added the click
listener using the .addEventListener()
function and for this to work, we will have to make sure the .getElementById("interactive-div")
returns the element. Hence we wrap the code in the DOMContentLoaded
event listener in the document
interface. Else, the code will throw an error saying that it cannot add event
to a null
object.