How to Execute the JavaScript Code After the Webpage Loads
-
Add the
script
Tag at the End of thebody
Tag in JavaScript -
Using the
onload
Event in JavaScript -
Use
onload
Event on thebody
Tag in JavaScript -
Use the
onload
Event on thewindow
Object Inside a JavaScript File
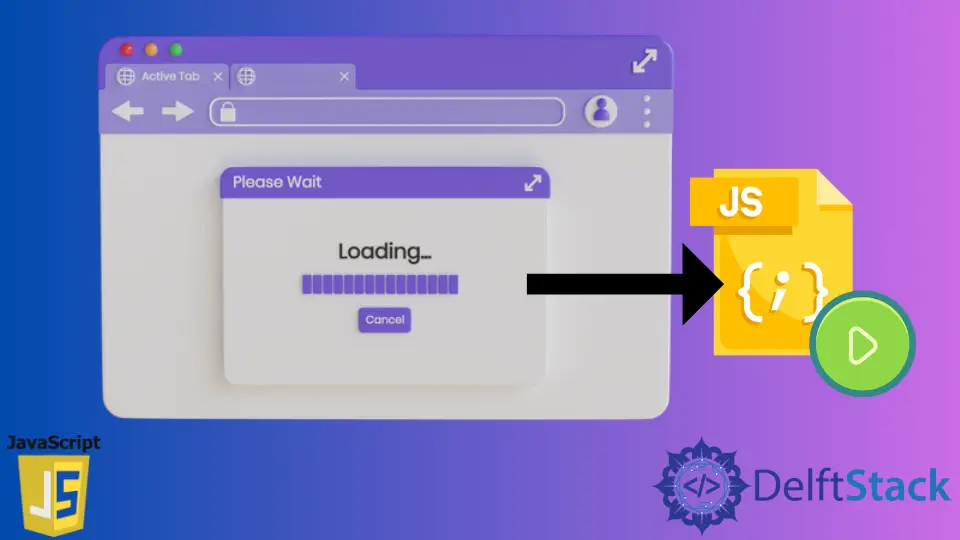
Before executing any JavaScript code, we always have to ensure that all the HTML elements are rendered on the browser first. Since we will be referring to these HTML elements inside our JavaScript code, those elements need to be loaded before the webpage.
Writing the script
tag inside the head
tag is not a good idea (this only make sense when you are loading third party scripts into your code) because the HTML document is executed from top to bottom and the head
tag is present before the body
tag. So, it will be executed first and then the body
tag.
Since all of our webpage contents are present inside the body
tag and the body
tag is present after the head
tag, adding the JavaScript code inside head
isn’t needed as it won’t be able to find the HTML elements. Take note that up to this point, the elements have not been created yet.
There are various ways to load the JavaScript after all the webpage’s contents are completely rendered on the screen. Below are some well-known ways which you can follow to execute the JavaScript code after the webpage is fully loaded.
Add the script
Tag at the End of the body
Tag in JavaScript
Adding a script
tag at the end of the body
tag is a common method of loading the JavaScript code. Up to this point, all the contents of the webpage are rendered properly on the screen. So, we can easily find or refer to these HTML elements inside our JavaScript code while not facing any error in the process.
The following program below shows how it is done.
<body>
<script></script>
</body>
Using the onload
Event in JavaScript
Another way to execute the JavaScript after the page is loaded is by using the onload
method. The onload
method waits until the page gets loaded. As soon as it does, this process then executes the JavaScript code.
There are two ways of writing a JavaScript code. One way is to write the JavaScript code inside the script
tag below the body
tag and then call the function inside the onload
method. You can also create a separate file for writing the JavaScript code, link that file inside your HTML at the end of the body
tag, and then call that function inside the onload
method.
Use onload
Event on the body
Tag in JavaScript
The onload
method requires a target
variable. In this case, we will be using the onload
method on the body
tag so that it will be the target
.
Inside the onload
method, we just have to pass in a function. Now, this function will only execute after all the contents of the webpage are fully loaded.
<body onload="myFunction()">
<h1>This is an example of onload.</h1>
<script>
function myFunction() {
console.log("function called...");
}
</script>
</body>
Output:
function called...
In the body
tag, we just have an h1
tag and a script
tag. In the script
tag, we have a myFunction()
function that prints a message to the function called...
console window. To execute this function after the page is loaded, we just have to pass it inside the onload
method.
Use the onload
Event on the window
Object Inside a JavaScript File
Another way of using the onload
method is on the window
object. The window object represents the entire browser window. After the elements inside the browser’s window are completely executed, we can execute our JavaScript code using the onload
method.
<body>
<h1>This is an example of onload.</h1>
<script>
window.onload = function () {
console.log("function called...");
}
</script>
</body>
Here, we have the same code as the previous one. The only difference here is that we are using the onload
method on the window
object, which is now the target. The program will eventually call the function and the message function called...
will be printed on the console window.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn