JavaScript List Comprehension
- The Syntactic Explanation for JavaScript List Comprehensions
- JavaScript List Comprehension with Map
-
Utilize the
for..of
Syntax to Iterate Through a List - Conclusion
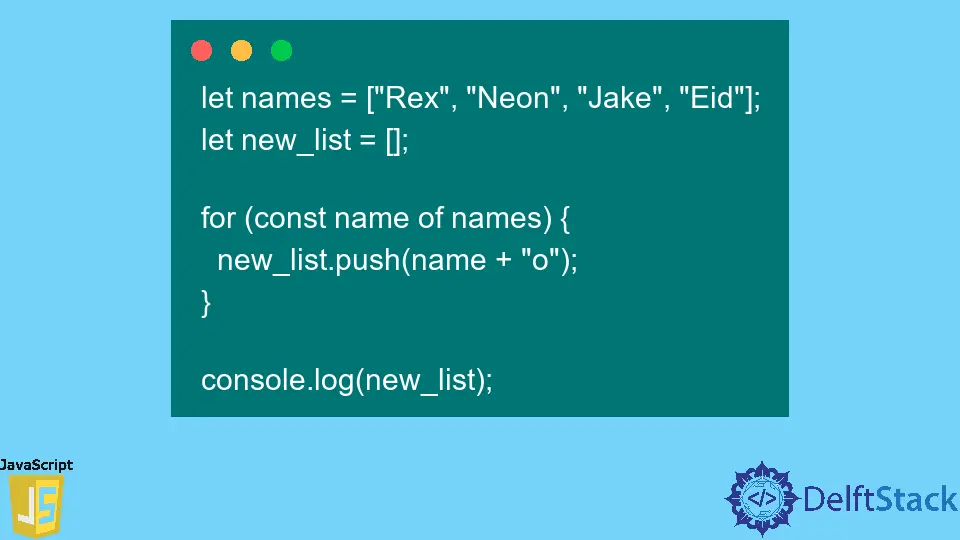
Many programming languages support a specific language syntax known as a list comprehension. We can use this list comprehension to derive a new list from a subsisting list.
Using the list comprehension syntax, a JavaScript expression, you might easily create a new list based on an existing one. The standard and Firefox’s implementation, however, no longer include it.
The standardization of list comprehension in ECMAScript 2016 was originally suggested because it offers a practical shortcut for building a new list based on the contents of an existing one.
List comprehension was formerly a proposed addition to JavaScript by the JavaScript Committee TC39, but it was abandoned in favor of other JavaScript list techniques like filter()
and map()
.
The filter()
or map()
method can be used with a list as an alternative to the list comprehension syntax. The list comprehension syntax in JavaScript is another name for this.
The Syntactic Explanation for JavaScript List Comprehensions
Applying a certain filter to an existing list using the filter()
method allows you to produce a new list from the original one.
The filter is a JavaScript expression that returns an element of a list upon passing the test function you define in the function body.
Example:
Let’s take a list as names = ["Rex", "Neon", "Jake", "Eid"]
to demonstrate the filter()
method in JavaScript and create a new list.
Code:
let names = ['Rex', 'Neon', 'Jake', 'Eid'];
let new_list = names.filter(function(name) {
return name.includes('e');
});
console.log(new_list);
Here we can get an output, as shown below.
The filter builds a new list to prevent changes to the original list.
As you can see, a good replacement for the list comprehension filter is the filter()
method.
The arrow function syntax also allows you to condense the method body into a single line.
Code:
let names = ['Rex', 'Neon', 'Jake', 'Eid'];
let new_list = names.filter(name => name.includes('e'));
console.log(new_list);
As the output, we can receive the same output from the previous example as [ 'Rex', 'Neon', 'Jake' ]
.
Note: One of the new functions in the ES6 edition of JavaScript is the arrow Function. Compared to conventional functions, it enables you to create functions more clearly.
For example:
If this is the original function:
let testFunction = function(m, n) {
return m * n;
}
By using the arrow function, the following can be written as a simpler version of this function:
let testFunction = (m, n) => m * n;
Let’s see how the map()
method can loop across a list next.
JavaScript List Comprehension with Map
List comprehension can also be used repeatedly on each list element as you go through it.
The map()
method enables you to build a new list from an existing one, just like the filter()
method.
However, the map()
method iterates across the existing list without filtering, allowing you to run code freely inside the callback function.
Example:
Let’s consider the list as names = ["Rex", "Neon", "Jake", "Eid"]
to demonstrate the map()
method in JavaScript and create a new list by adding the letter "o"
to each element of the list.
Code:
let names = ['Rex', 'Neon', 'Jake', 'Eid'];
let new_list = names.map(function(name) {
return name + 'o';
});
console.log(new_list);
Here, as shown below, we will receive the result.
The map()
function can be condensed to a single line using the arrow function.
Code:
let names = ['Rex', 'Neon', 'Jake', 'Eid'];
let new_list = names.map(name => name + 'o');
console.log(new_list);
As a result, we can receive the same output from the previous example as [ 'Rexo', 'Neono', 'Jakeo', 'Eido' ]
.
You can iterate through any JavaScript list and run a given callback function using the map()
method.
Utilize the for..of
Syntax to Iterate Through a List
As an alternative, you can also iterate through a list using the operator for..of
, as shown below.
let names = ['Rex', 'Neon', 'Jake', 'Eid'];
let new_list = [];
for (const name of names) {
new_list.push(name + 'o');
}
console.log(new_list);
Here we can receive the output as follows.
With the above example in mind, each iteration creates a new name
variable that holds the element stored in the list.
Inside the method for..of
, push each name
element into the new_list
. For each element, we have the freedom to choose the desired operator.
Conclusion
This article expresses the methods used in list comprehension in JavaScript by examining the functions such as the filter
function, map
function and for..of
iteration.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.