How to Create Keyboard Shortcuts Using JavaScript
- Use Plain JavaScript to Create Keyboard Shortcuts in JavaScript
-
Use
hotkeys.js
Library to Create Keyboard Shortcuts in JavaScript - Use jQuery Library to Create Keyboard Shortcuts in JavaScript
-
Use
mousetrap.js
Library to Create Keyboard Shortcuts in JavaScript
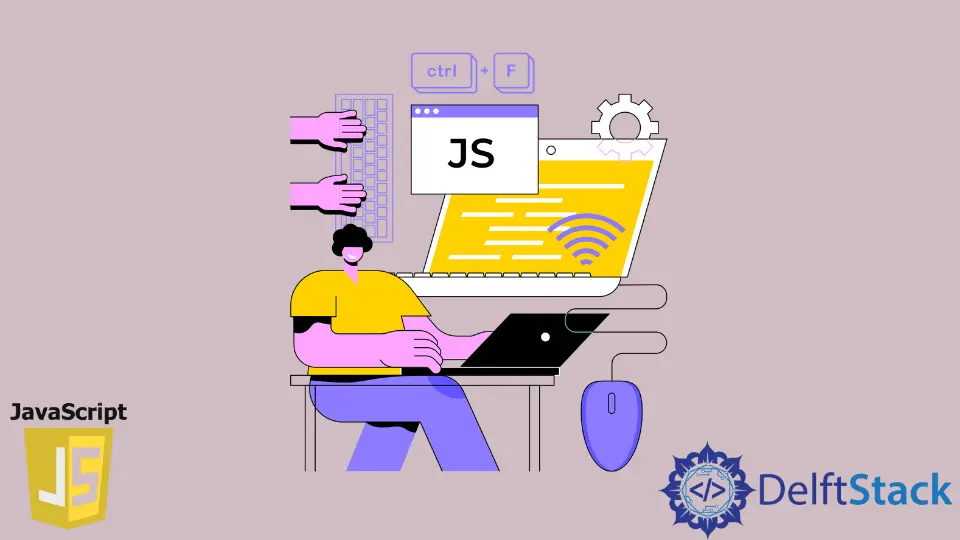
Do you want to spice up your blog or website using keyboard shortcuts?
This tutorial teaches you how to create keyboard shortcuts using JavaScript.
One or multiple properties, including shiftKey
, altKey
, ctrlKey
, key
, can be used by a user to get the pressed key’s value.
We can use the approaches that are listed below.
- Plain JavaScript
- Use
hotkeys.js
library - Use the jQuery library
- Use
mousetrap.js
library
Let’s start with plain JavaScript.
Use Plain JavaScript to Create Keyboard Shortcuts in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Pure JS to Create Keyboard Shortcuts</title>
<script src="./script.js"></script>
</head>
<body>
<p>Press Alt+C</p>
</body>
</html>
JavaScript Code:
document.addEventListener('keydown', function(e) {
if (e.altKey && e.code === 'KeyC') {
alert('Alt + C is pressed!');
e.preventDefault();
}
});
This example uses addEventListener()
to listen to the event named keydown
. To implement the Alt+C keyboard event shortcut, we use the KeyboardEvent.altKey
and the KeyboardEvent.code
.
The read-only property named KeyboardEvent.altKey
is a Boolean result that tells whether the Alt is pressed or not. It returns true
on pressing the key, otherwise false
.
KeyboardEvent.code
shows the physical key of the keyboard.
Finally, the program shows a message using alert()
if the expected keypress is detected, and the preventDefault()
function stops the event (it can only cancel or stop the cancelable events). We can also use the KeyboardEvent.key
instead of the KeyboardEvent.code
; see the following example.
JavaScript Code:
document.addEventListener('keydown', function(e) {
if (e.altKey && (e.key === 'c' || e.key === 'C')) {
alert('Alt + C is pressed!');
e.preventDefault();
}
});
Use hotkeys.js
Library to Create Keyboard Shortcuts in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Pure JS to Create Keyboard Shortcuts</title>
<script src="https://unpkg.com/hotkeys-js/dist/hotkeys.min.js">
</script>
<script src="./script.js"></script>
</head>
<body>
<p>Press Alt+C</p>
</body>
</html>
JavaScript Code:
hotkeys('alt+c', function(event, handler) {
alert('Alt + c is pressed!');
event.preventDefault();
});
We are using the hotkeys.js
library to create a keyboard shortcut for Alt+C. To use it, you must have Node.js installed on your machine or include it via the <script>
tag.
We can also use it for multiple keyboard shortcuts as follows.
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Pure JS to Create Keyboard Shortcuts</title>
<script src="https://unpkg.com/hotkeys-js/dist/hotkeys.min.js">
</script>
<script src="./script.js"></script>
</head>
<body>
<h1>You Can Press the Following Keyboard Shortcuts</h1>
<ul>
<li>Press Ctrl+A</li>
<li>Press Ctrl+B</li>
<li>Press A</li>
<li>Press B</li>
</ul>
</body>
</html>
JavaScript Code:
hotkeys('ctrl+a,ctrl+b,a,b', function(event, handler) {
switch (handler.key) {
case 'ctrl+a':
alert('You pressed Ctrl+A');
break;
case 'ctrl+b':
alert('You pressed Ctrl+B');
break;
case 'a':
alert('You pressed A!');
break;
case 'b':
alert('You pressed B!');
break;
default:
alert(event);
}
});
Use jQuery Library to Create Keyboard Shortcuts in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Pure JS to Create Keyboard Shortcuts</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<script src="./script.js"></script>
</head>
<body>
<p>Press Alt+X</p>
</body>
</html>
JavaScript Code:
$(document).keydown(function(e) {
if (e.altKey && e.which === 88) {
alert('You pressed Alt + X');
e.preventDefault();
}
});
We use the jQuery library’s keyboard()
function, which triggers the keydown
event when keyboard keys are pressed. The KeyboardEvent.which
has the numeric value and denotes which mouse button or keyboard key is pressed.
Remember, you may have to choose anyone from the event.which
or event.keyCode
depends on what is supported by your browser.
Use mousetrap.js
Library to Create Keyboard Shortcuts in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Pure JS to Create Keyboard Shortcuts</title>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/mousetrap/1.6.3/mousetrap.min.js">
</script>
<script src="./script.js"></script>
</head>
<body>
<p>Press Alt+C</p>
</body>
</html>
JavaScript Code:
Mousetrap.bind(['alt+c'], function() {
alert('You pressed Alt+C');
return false;
})
Now, we are using the mousetrap.js
library to handle keyboard shortcuts. We can use it if you have Node.js or manually add it via the <script>
element.
You can dig in detail here.