How to isset Equivalent in JavaScript
-
isset
Equivalent in JavaScript -
Check if Value Is Set or Not Using the
in
Operator in JavaScript -
Check if Value Is Set or Not Using
Object.hasOwnProperty()
in JavaScript
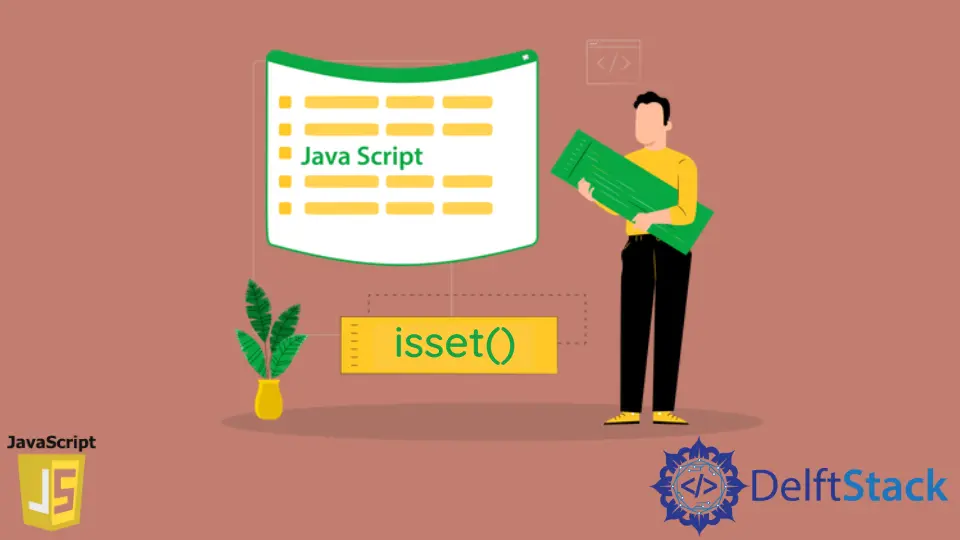
PHP provides a function isset
that determines if a variable is set; this means if a variable is declared and assigned a value other than null. When a variable is assigned to null, isset()
will return false. In JavaScript, we need to determine whether a variable is set or not.
In this post, we will learn how to check if a variable is set or not.
isset
Equivalent in JavaScript
JavaScript provides the typeof
operator. It returns a string indicating the operand type that wasn’t evaluated. This operator is useful when the operand type is unknown, and depending on the type, you can perform the next task.
If the operand type is a number, add the numbers, then it will concatenate the strings.
Syntax:
typeof operand typeof (operand)
It requires an expression representing the primitive or object whose type must be returned. There are eight results depending on the expression passed to it. It can either be undefined
, string
, symbol
, function
, object
, boolean
, number
, or object
.
For more information, read the documentation of typeof
.
const inputObject = {
id: 42,
name: 'John Doe'
};
let phone
console.log(typeof inputObject.id !== 'undefined');
if ((typeof phone === 'undefined')) {
phone = '7878787878';
}
console.log(phone);
In the example, we defined two properties of an object id
and name
. When you check typeof inputObject.id
, it will check if the id property exists in inputObject
. To check the opposite condition, add !
before the condition, then it will negate the check.
For a single variable, you can check if it’s set or not. You can add an assigned value to phone
if it doesn’t exist.
The output will look like this.
true
"7878787878"
Check if Value Is Set or Not Using the in
Operator in JavaScript
The in
operator is a built-in method to verify that the specified property belongs to an object or its prototype chain. It repeats the object and returns the Boolean value according to the result.
Syntax:
prop in object
This function takes prop
as input in the string format, and it’s a required parameter. This method checks whether the specified property or the array index exists in Object
or prototype chain. Since the array is derived from an object, this method can also be called for an array.
For more information, read the documentation of the in
operator method.
const inputObject = {
id: 42,
name: 'John Doe'
};
console.log('name' in inputObject);
if (!('country' in inputObject)) {
inputObject.country = 'India';
}
console.log(inputObject);
In the example, we defined two properties of an object id
and name
. If you pass name
in inputObject
, it checks whether the name property exists in inputObject
or not. Add !
in front of the condition to verify the opposite condition, refusing verification.
You can add a new country
property if it doesn’t exist within an object. The output will look like this.
true
{
id: 42,
name: "John Doe",
country: "India"
}
Check if Value Is Set or Not Using Object.hasOwnProperty()
in JavaScript
hasOwnProperty
is also a built-in method that checks whether the specified property exists in an Object
or not. It repeats the object and returns the Boolean value according to the result.
Syntax:
Object.prototype.hasOwnProperty(prop);
This function takes prop
as input in the format string
and is a required parameter. This method checks whether the specified property exists or not regardless of the value in Object.
Even if the property’s value is null
or undefined
, it will return true
. This method can also be called for an Array
, since the array is derived from an object.
For more information, read the documentation of hasOwnProperty()
method.
const inputObject = {
name: 'John Doe'
};
console.log(inputObject.hasOwnProperty('name'));
if (!inputObject.hasOwnProperty('email')) {
inputObject.email = 'Johndoe@gmail.com';
}
console.log(inputObject);
In the example, we defined two properties of an object id
and name
. If you pass inputObject.hasOwnProperty ('name')
, it will check whether the name property in inputObject
exists or not. Add !
in front of the condition to verify the opposite condition, refusing verification.
We can add a new property if it doesn’t exist in an object. The output is shown below.
true
{
email: "Johndoe@gmail.com",
name: "John Doe"
}
The difference between hasOwnProperty
and in
operator is that hasOwnProperty
returns false if the property is inherited within the object or not declared. In contrast, the in operator
doesn’t validate the object in the specified property - prototype string.
The base class Object
has hasOwnProperty
as its function, so it returns false
, while in operator
returns true
.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn