How to Get Textbox Value in JavaScript
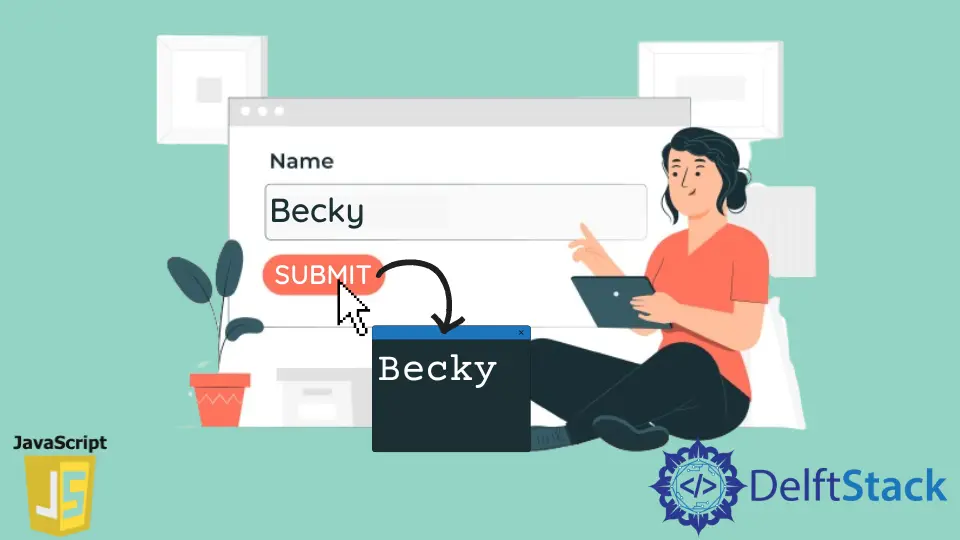
This tutorial educates about the text value property and jQuery val() function to get the value of a JavaScript textbox.
The text value property is used to return or set the value of the input field’s value attribute. The value attribute displays the input textbox’s initial value. It can have user-defined values or default values.
The jQuery val()
method is to get the form element’s value. Here, the form element means the input
, textarea
, and select
elements. It returns the undefined
if called on an empty collection. The val()
function sets or returns the value of the selected elements’ value attribute.
Using this function, we can set the value attribute’s value for all matched elements and return the value attribute’s value of the first matched element. Remember, this function is mainly used with HTML form elements.
The HTML code will remain the same for all examples (except the one using <form>
element), but JavaScript code will keep changing to practice different ways.
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title> Learning JavaScript Get Textbox Value</title>
</head>
<body>
Full Name: <input type="text" id="fullName" name="fullName" value="Mehvish Ashiq">
<button type="button" onclick="nameFunction()">Submit</button>
<p id="displayName"></p>
</body>
</html>
Different Ways to Get the Value of JavaScript Textbox
Various JavaScript code snippets are given below to get the value of the JavaScript textbox.
function nameFunction() {
var element = document.getElementById('fullName').value;
document.getElementById('displayName').innerHTML = element;
}
OUTPUT:
The first method that we are learning to get the value of textbox is document.getElementById()
. It selects the first element with the id attribute with the particular value (here, it is fullName
), gets its value by using the .value
property, and saves it into the element
variable. The document.getElementById()
is used again to modify the content of an element having id’s value displayName
. To update the content, .innerHTML
property is used.
function nameFunction() {
// first element in DOM (index 0) with name="fullName"
var element = document.getElementsByName('fullName')[0].value
document.getElementById('displayName').innerHTML = element;
}
OUTPUT:
The above JavaScript code uses document.getElementByName
to get the list of all elements having the given name (fullName
for this instance). The element’s value at index 0 is selected from the list of all elements. This can also be used if your element does not have an id attribute.
function nameFunction() {
document.getElementById('displayName').innerHTML =
document.forms[0].elements[0].value;
}
OUTPUT:
The above code modifies the content of an element whose id’s value is displayName
and assigns the value received from index 0. Remember, you must have a <form>
element in your HTML file to get value by index.
function nameFunction() {
document.getElementById('displayName').innerHTML = $('input:text').val();
}
OUTPUT:
The code snippet given above presents the jQuery method val()
, which is used to access the value of the JavaScript textbox. This method gets the value of the first input
element whose type is text
. Don’t forget to include jQuery in the <head>
tag.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>