How to Get the Value of the Selected Option From the Drop-Down List in JavaScript
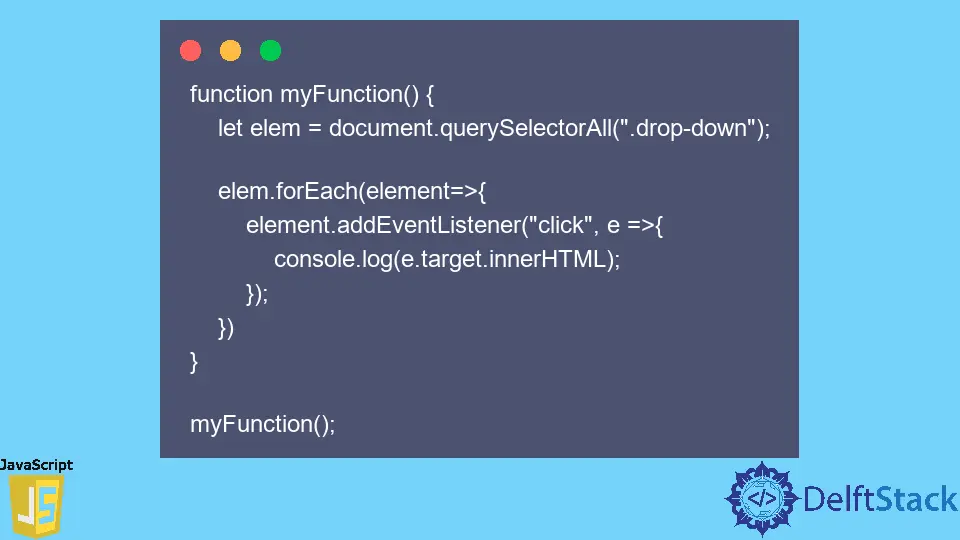
In JavaScript, working with or keeping track of user interactions like mouse clicks or keyboard keypresses is challenging. Thanks to the JavaScript events, it is easier for us to keep track of the user’s interactions and get the updated values after the users on the webpage make some changes.
For example, if there is a drop-down menu and you have to select an option from the list of all the other choices, here’s what would happen: you can easily get the value of the option which you have selected from the drop-down list with the help of mouse click
events.
You will see how to implement this in detail as you read this article.
Get the Selected Option From a List Using Mouse Events in JavaScript
Events in JavaScript are a great way to keep track of the user’s interactions on the webpage, like mouse clicks or keyboard click events. There are various events available in JavaScript, a full list of which can be found here. Out of all these events, we will only focus on discussing the mouse click
event.
Whenever users click on any of the options present inside the drop-down list, our mouse click
event will be triggered. With this feature, we can easily get the value of the selected option from the drop-down.
Let’s now see how it works during execution.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<select id="list">
<option class="drop-down">test1</option>
<option class="drop-down" selected="selected">test2</option>
<option class="drop-down">test3</option>
</select>
<script src="./script.js"></script>
</body>
</html>
The following is the JavaScript code.
function myFunction() {
let elem = document.querySelectorAll('.drop-down');
elem.forEach(element => {
element.addEventListener('click', e => {
console.log(e.target.innerHTML);
});
})
}
myFunction();
Output:
test2
test3
test1
Note that the output will vary depending on which option has been selected by the user from the drop-down.
Inside our HTML, we have a select
tag with an id
of list
, which will be used to create a drop-down list. In this case, there are three options inside the select
tag, each of which has a class name of drop-down
. And at the end, we are linking the JavaScript code at the end of the body
tag since it is present in a separate file.
Inside the JavaScript, we have a function named myFunction()
. Here, we first get all the option elements of the drop-down list using the class name drop-down
using the querySelectorAll
property. This process will return an array containing all the options of the drop-down list. Then, we will store this array inside a variable called elem
.
Now that we have all the options stored in the variable elem
, we will use a for each
loop on that variable. The for each
loop will help us get the individual elements of the array, so we can now add event listeners to each of these option elements of the array.
The individual elements inside the array can be accessed with the variable element
, passed as an argument to the for each
loop. To add an event to element
, we have to use a method provided by JavaScript called addEventListener()
, which takes two arguments.
The first argument is the click
event, and the second argument is the callback
function. The click
event tells the browser to execute the callback
function only when a user clicks (left mouse button) on any of the options from the drop-down. The callback
function itself takes an argument called e
or event which will help us get the element that the user selects.
In the callback
function, you can write whatever code you want. Since we want to get the value of the option selected in this case, we will use e.target
. This command will tell which HTML element is currently being selected.
Finally, to get the value of that option selected, we can use e.target.innerHTML
and pass this inside the console.log()
method to see the output in the console window.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn