How to Get Select Value From DropDown List With JavaScript and jQuery
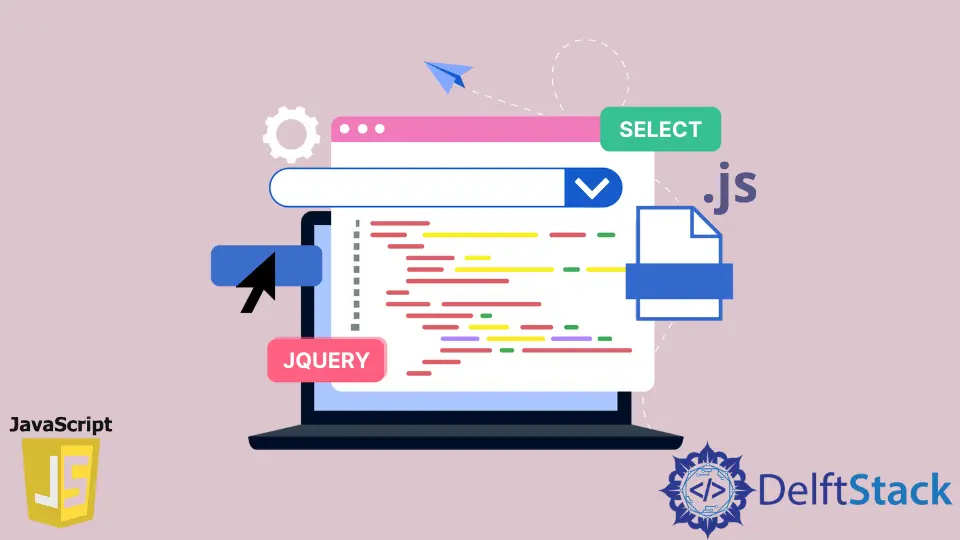
This article will teach you to get a select value using vanilla JavaScript and jQuery. We’ll explain two examples; the first shows the JavaScript approach and the second example uses jQuery.
Get the Select Value With Vanilla JavaScript
Once you’ve defined a <select>
element in HTML, you can access its value via JavaScript. That’s because JavaScript provides methods and functions that can manipulate an element.
However, they vary depending on the element. So, for every <select>
element, you can access its value using the following:
selectelement.value
options
property andselectedIndex
Get the Select Value Using Selectelement.value
The <select>
element has a value
property that contains the value of the selected element. So, we have some countries in the <select>
element in the following code.
When you click on the HTML button, you’ll get the select value. Also, you’ll note that each <option>
of the <select>
element has a value
property.
You can choose to remove this property or leave it. You’ll get the same result.
Code:
<head>
<meta charset="utf-8">
<title>Get Selected Value With Vanilla JS</title>
<style>
body {
display: grid;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
</head>
<body>
<main>
<form>
<label>Pick a food</label>
<select id="select_menu">
<option value="Rice">Rice</option>
<option value="Carrot" selected="selected">Carrot</option>
<option value="Avocado">Avocado</option>
<option value="Banana">Banana</option>
</select>
<button id="submit_button">Get Food Name</button>
</form>
</main>
<script>
let submit_button = document.getElementById("submit_button");
let select_menu = document.getElementById("select_menu")
submit_button.addEventListener("click", function(event) {
// Prevent the submission of the form
event.preventDefault();
var selected_value = "Hooray!, you selected " + select_menu.options[select_menu.selectedIndex].value;
alert(selected_value);
});
</script>
</body>
Output:
Get the Select Value Using the options
Property and selectedIndex
Accessing the <options>
property gives you access to the selectedIndex
property. This property represents the currently selected element.
Also, the selectedIndex
property has the value property that returns the selected value.
For example, we have food names in the <select>
element in the following code. So, you can select a food name and click on the button to get its value.
Code:
<head>
<meta charset="utf-8">
<title>Get Selected Value With Vanilla JS</title>
<style>
body {
display: grid;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
</head>
<body>
<main>
<form>
<label>Where are you from?</label>
<select id="select_menu">
<option value="Germany">Germany</option>
<option value="Ethiopia" selected="selected">Ethiopia</option>
<option value="Romania">Romania</option>
<option>Madagascar</option>
</select>
<button id="submit_button">Get Country Name</button>
</form>
</main>
<script>
let submit_button = document.getElementById("submit_button");
let select_menu = document.getElementById("select_menu")
submit_button.addEventListener("click", function(event) {
// Prevent the submission of the form
event.preventDefault();
var selected_value = "Hello, you are from: " + select_menu.options[select_menu.selectedIndex].value;
alert(selected_value);
});
</script>
</body>
Output:
Get the Select Value With jQuery
The jQuery library simplifies many vanilla JavaScript tasks, including getting a select value. Meanwhile, you are not obliged to solve a task in jQuery the same way you’ll do it in vanilla JavaScript.
So, we can take a different approach. Still, we’ll get the select value.
The first change is that the <option>
elements do not have the value
property. Another change is our approach to getting the selected element.
Here, we use jQuery .change()
API on the <select>
element. This means we can get the select value every time we choose it.
Code:
<head>
<meta charset="utf-8">
<title>Get Selected Value With jQuery</title>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"
integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ=="
crossorigin="anonymous"
referrerpolicy="no-referrer">
</script>
<style>
body {
display: grid;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
</head>
<body>
<main>
<form>
<label>Choose a Language</label>
<select id="programming_language">
<option>Swift</option>
<option>Obective-C</option>
<option>Erlang</option>
<option>COBOL</option>
<option>FORTRAN</option>
</select>
</form>
</main>
<script>
$(document).ready(function () {
$('#programming_language').change(function () {
var programming_language = document.getElementById("programming_language");
var selected_value = programming_language.options[programming_language.selectedIndex].value
alert("You selected " + selected_value);
});
});
</script>
</body>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn